This article covers the uses of Strings in Java.
Strings are an integral part of any programming language due to their ability to store text. In Java, Strings have dozens of different methods associated with them which are used to manipulate and retrieve data from them efficiently.
Most of these methods are designed for “String Parsing” which is the act of going through pieces of texts extracting information form them. We’ll discuss several such examples of string parsing below.
Declaring Strings
Strings are declared using the String keyword.
String text = "Hello World";
Any text assigned to the variable text
must be in double quotations. Though it can vary from system to system, there is almost no limit to the amount of text a String can hold.
Converting Strings
Often, you’ll need to convert other data types into String format, mostly Integers to Strings. There are many ways in Java to do conversions, and one way may not apply to all data types, but the most reliable one is the valueof()
function. It works on Long, Double, Float and Integers.
String a = String.valueof(123);
String b = String.valueof(1.5);
Sub-strings in Java
The substring
function in Java is used to pick out certain parts of a string and return them. The substring
function can takes either one or two parameters. If only one parameter is created, like the one below, the substring function will return all characters starting from the index specified. Remember that indexing starts from zero in Java.
String s = "Java Strings";
System.out.println(s.substring(7));
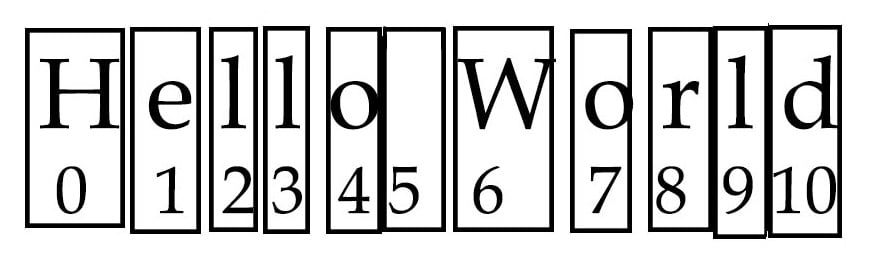
Creating two parameters in the substring
allows you to define a start point and an end point. Anything within these two indexes is returned. substring(6,11)
will include the character in the 6th index, but not the 11th index. A slight benefit of this is that subtracting both indexes gives you the length of the substring.
String s = "Hello World";
System.out.println(s.substring(0, 5));
System.out.println(s.substring(6, 11));
Once again, the 5th and 11th indexes in the examples above are not included in the output.
Hello
World
Java String Indexes
Strings in Java have a wide variety of different functions related to their indexes.
The indexOf
function is applied on a string, and returns the index of the character(s) you wish to find within that string. Only returns the index of the first character that it encounters. Returns -1 if not found.
String s = "Hello World";
System.out.println(s.indexOf('e'));
System.out.println(s.indexOf('l'));
System.out.println(s.indexOf('a'));
For the character l
only the first index is returned, which is at 2. The l
at index three was not reached.
1
2
-1
You aren’t restricted to just single characters either. You can insert entire Strings in the indexOf
function. Keep in mind however, the index returned will be of the start of the string, or in other words the index of the first character of the string.
String s = "Hello World";
System.out.println(s.indexOf("Hello"));
System.out.println(s.indexOf("World"));
0
6
The indexOf
function allows us to use two parameters, where the first is the string or character we’re looking for and the second is the starting position. By default the starting position is 0.
We’ll use this function creatively to find the location of both l’s in Hello World.
String s = "Hello World";
int pos;
System.out.println(s.indexOf('l'));
pos = s.indexOf('l');
System.out.println(s.indexOf('l', pos+1));
First, we found the location of the first l and then did ran the indexOf
function again, starting after the location of the first l
. pos
has the value 2, the location of the first l, so we add one, so that the indexOf
function starts from 3.
Finally we have the lastIndexOf
function. As you might have guessed by it’s name, it returns the index of the last found character passed into it’s parameters.
String s = "Hello World";
System.out.println(s.lastIndexOf('l'));
The last l was located at index 9, hence 9 was returned.
9
Other Java Strings Functions
The toLowerCase()
function converts all the uppercase characters in a string to lower case characters.
String s = "Hello World";
System.out.println(s.toLowerCase());
hello world
The toUpperCase()
function converts all the lower case characters in a string to upper case characters.
String s = "Hello World";
System.out.println(s.toUpperCase());
HELLO WORLD
The startsWith()
function is used on a string to see if starts with a specific string or character. Keep in mind that this function can distinguish between Upper and Lower case characters. In some cases, you might find it useful to convert the string to all upper or lower case before using the startsWith()
function.
String s = "Hello World";
System.out.println(s.startsWith("H"));
System.out.println(s.startsWith("h"));
System.out.println(s.startsWith("Hello"));
true
false
true
The opposite of the startsWith()
function, the endsWidth()
checks to see if a string ends with a certain character or string.
String s = "Hello World";
System.out.println(s.endsWith("d"));
System.out.println(s.endsWith("W"));
System.out.println(s.endsWith("World"));
true
false
true
The charAt()
is the reverse of the indexOf()
function. You pass a index into it, and it will return character at that index.
String s = "Hello World";
System.out.println(s.charAt(6));
System.out.println(s.charAt(2));
W
l
The isEmpty()
checks a string to see if it’s empty or not. (A null string has the value ""
).
String s = "Hello World";
String a = "";
System.out.println(s.isEmpty());
System.out.println(a.isEmpty());
false
true
This marks the end of our Java Strings article. Any suggestions or contributions for CodersLegacy are more than welcome. Any questions can be asked below in the comments.