This article covers the JavaFX CheckBox widget.
CheckBoxes are a common GUI element for taking User input. Along with RadioButtons, they display to the User a set of options in form of a selectable circle/box. Unlike RadioButtons however, there are no restrictions on the number of Checkboxes that you can select. You can even choose not to select any.
A complete list of important methods for the JavaFX CheckBox can be found at the end of this page.
Creating a CheckBox
We’ll be creating two Check-Boxes in the code below. You’ll rarely see a checkbox alone; it just feels more natural to have multiple of them.
package application;
import javafx.application.Application;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.control.CheckBox;
import javafx.scene.layout.VBox;
public class Tutorial extends Application {
public static void main(String args[]){
launch(args);
}
@Override
public void start(Stage primaryStage) throws Exception {
VBox layout = new VBox();
Scene scene = new Scene(layout, 250, 150);
CheckBox check1 = new CheckBox("Option 1");
CheckBox check2 = new CheckBox("Option 2");
layout.getChildren().addAll(check1, check2);
primaryStage.setTitle("CodersLegacy");
primaryStage.setScene(scene);
primaryStage.show();
}
}
The GUI output of the above code:
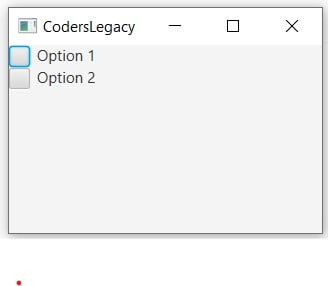
The Blue highlight does not represent a selected CheckBox. It just indicates the default CheckBox, which is the first one.
Retrieving CheckBox Values
Before we continue, you should know about the “Indeterminate stage” of the JavaFX CheckBox. One might think that a CheckBox has two stages, selected and deselected, but there are actually three. The Indeterminate stage represents a stage where the CheckBox has not been interacted with. The User has neither selected or deselected it (yet).
However, by default the Indeterminate state is disabled. You can enable it using the setAllowIndeterminate()
function. For simple purposes it’s not required, but having it will increase the control you have over your GUI. Using the isIndeterminate()
method will return True of False, depending on the state of the widget.
The rest of this is fairly simple. We’ll use a button widget which when clicked will call the isSelected()
method on the CheckBox, returning a True of False value depending on whether it was selected or not.
package application;
import javafx.application.Application;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.CheckBox;
import javafx.scene.layout.VBox;
public class Tutorial extends Application {
public static void main(String args[]){
launch(args);
}
@Override
public void start(Stage primaryStage) throws Exception {
VBox layout = new VBox();
Scene scene = new Scene(layout, 250, 150);
CheckBox check1 = new CheckBox("Option 1");
CheckBox check2 = new CheckBox("Option 2");
Button button = new Button("Submit");
button.setOnAction(e -> {
System.out.println(check1.isSelected());
System.out.println(check2.isSelected());
});
layout.getChildren().addAll(check1, check2, button);
primaryStage.setTitle("CodersLegacy");
primaryStage.setScene(scene);
primaryStage.show();
}
}
Since their two CheckBoxes, each button click will return two values. Below is a nice little GIF showing a demo of the above code.
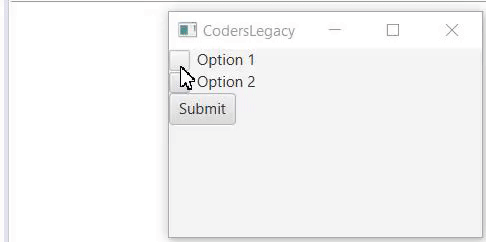
CheckBox Methods
This is a compilation of many useful methods that can be used with the CheckBox widget.
Method | Description |
---|---|
setSelected(bool) | Sets the value of the checkbox in it’s selected state. |
isSelected() | Returns a Boolean value indicating whether the checkbox was selected or not. |
setAllowIndeterminate() | Allows the possibility of the indeterminate state. |
setInderminate(bool) | Sets the value of the checkbox in it’s indeterminate state. |
isIndeterminate() | Returns a Boolean value indicating whether the checkbox was in an indeterminate state or not. |
This marks the end of the JavaFX CheckBox article. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.