In this tutorial we will discuss how to do Event Handling in JavaFX using the EventHandler.
Whenever we introduce the concept of “events” into our GUI application, such as a “button click event” or a “menu selection event”, we need to define a function which “handles” the event. This function will execute whenever the event occurs. In order to define this function, we need the help of the JavaFX EventHandler Interface.
Using JavaFX EventHandler with Button
The most common use of the JavaFX EventHandler is with a button. Lets take a look at an example code where we use the EventHandler to define a function which prints out “Hello World” whenever the button is clicked.
First we need to make an import for the EventHandler.
import javafx.application.Application;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
There are a few other extra imports that we made, for other essential components in our GUI. It is also important to import “Action Event” as we will be needing this along with EventHandler.
Next we need to define our Class.
public class Tutorial extends Application {
public static void main(String args[]){
launch(args);
}
}
In order to use Event Handling inside this Class, we need to implement the EventHandler interface into our Class as shown below.
public class Tutorial extends Application implements EventHandler<ActionEvent> {
public static void main(String args[]){
launch(args);
}
}
We will now define the rest of our JavaFX application.
@Override
public void start(Stage primary) throws Exception {
StackPane layout = new StackPane();
Scene scene = new Scene(layout, 300, 300);
button1= new Button("Click Me!");
button1.setOnAction(this);
layout.getChildren().addAll(button1);
primary.setTitle("Event Handling Tutorial");
primary.setScene(scene);
primary.show();
}
The main thing to focus on here, is that we created a button, and used setOnAction()
to bind it. Now it will trigger an event whenever it is clicked. But we need to actually “handle” this event ourselves and define what happens when it is clicked.
We will define a new method in our Class as shown below. This function will be executed when any event is triggered, not just the events from the button we defined. We need to setup proper if-statements to first check who triggered the event, then take action accordingly.
public void handle(ActionEvent event) {
if(event.getSource() == button1) {
System.out.println("Hello World");
}
}
Note: button1 is the name of the variable we defined earlier.
And that’s all! We have now added Event Handling into our JavaFX Application.
Final Result (Output)
Here is the complete code + output screenshot.
We had to make one additional modification here, which was to add the Button as a field in our Class. This was to make it accessible in other functions of our Class (like the handle function).
package application;
import javafx.application.Application;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
public class Tutorial extends Application implements EventHandler<ActionEvent> {
private Button button1;
public static void main(String args[]){
launch(args);
}
@Override
public void start(Stage primary) throws Exception {
StackPane layout = new StackPane();
Scene scene = new Scene(layout, 300, 300);
button1= new Button("Click Me!");
button1.setOnAction(this);
layout.getChildren().addAll(button1);
primary.setTitle("Event Handling Tutorial");
primary.setScene(scene);
primary.show();
}
public void handle(ActionEvent event) {
if(event.getSource() == button1) {
System.out.println("Hello World");
}
}
}
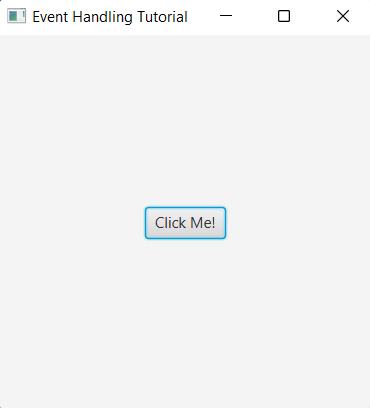
There is an easier and faster way of handling events in JavaFX, without having to define a separate function or use EventHandler. Follow the link for more information.
This marks the end of the JavaFX EventHandler Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.