This article covers the layout manager, HBox in JavaFX.
The JavaFX HBox layout component creates a layout which positions all the GUI components in a horizontal row next to each other. With it’s Default state, the HBox layout is very simplistic and not suited to be used in an actual GUI program.
In this Tutorial we will go through the many different options and settings available to make our JavaFX HBox layout more practical and appealing.
HBox Layout Example
We’ll be using three buttons to demonstrate how their position changes in the VBox layout. We haven’t added any functionality to the buttons, they are just for show.
Take a good look at the imports because they won’t be repeated in the code that comes after this.
package application;
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.geometry.Insets;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.HBox;
public class Tutorial extends Application {
public static void main(String args[]){
launch(args);
}
@Override
public void start(Stage primaryStage) throws Exception {
Button Button1 = new Button("Button1");
Button Button2 = new Button("Button2");
Button Button3 = new Button("Button3");
HBox layout = new HBox(Button1, Button2, Button3);
Scene scene = new Scene(layout, 300, 300);
primaryStage.setTitle("JavaFX HBox Example");
primaryStage.setScene(scene);
primaryStage.show();
}
}
We passed all three buttons that we created into the HBox classes while we created it, automatically adding them to it’s layout. Alternatively you could have used the getChildren().add()
method, but that takes longer.
This is how these three buttons look with the default HBox layout.
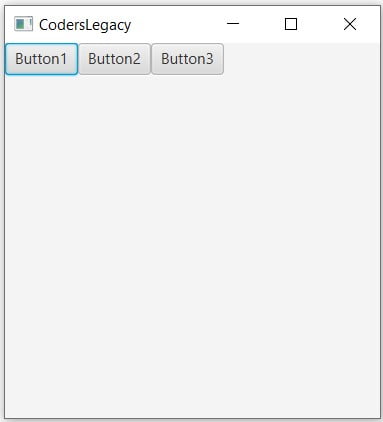
Alignment Options
You can control the alignment of the GUI components in the layout with the setAlignment()
function. There are over a dozen options available so it’s best to experiment with them and try them out yourself. The format is quite simple as shown below.
layout.setAlignment(Pos.BASELINE_CENTER);
A list of all the possible alignment options that can be passed into the setAlignment()
function.
- Pos.BASELINE_LEFT
- Pos.BASELINE_RIGHT
- Pos.BASELINE_CENTER
- Pos.BOTTOM_LEFT
- Pos.BOTTOM_RIGHT
- Pos.BOTTOM_CENTER
- Pos.CENTER_LEFT
- Pos.CENTER_RIGHT
- Pos.CENTER
- Pos.TOP_LEFT
- Pos.TOP_RIGHT
- Pos.TOP_CENTER
Spacing Options
The Spacing property determines the amount of “spacing” or “gap” between the GUI components in the layout. By default, the value of this is 0, but by with the setSpacing()
method we can modify it’s value.
HBox layout = new HBox(Button1, Button2, Button3);
layout.setSpacing(30);
Scene scene = new Scene(layout, 300, 250);
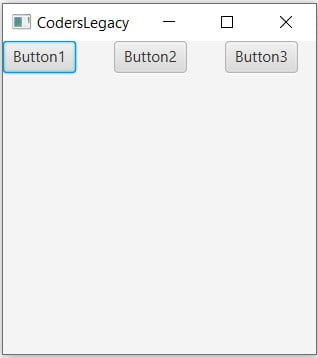
HBox layout = new HBox(20, Button1, Button2, Button3);
Instead of using the setSpacing()
function you can instead pass the spacing as the first argument in the HBox class.
Margin Options
You can also set Margins to the HBox layout using the setMargin()
function. If you’ve studied CSS, this is the same thing as the padding property. Margins are useful to create some distance between the GUI components and the edge of the GUI window.
We’ll have to make use of the Inset class here, using four parameters which represent the 4 sides of our VBox layout.
HBox layout = new HBox(Button1, Button2, Button3);
HBox.setMargin(Button1, new Insets(20,20,20,20));
HBox.setMargin(Button2, new Insets(20,20,20,20));
HBox.setMargin(Button3, new Insets(20,20,20,20));
Scene scene = new Scene(layout, 300, 250);
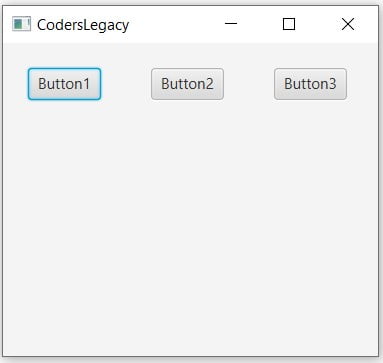
Hgrow
The Vgrow property determines whether a GUI component will grow if there is horizontal space available in the Window.
HBox.setHgrow(Button1, Priority.ALWAYS);
HBox.setHgrow(Button2, Priority.ALWAYS);
HBox.setHgrow(Button3, Priority.ALWAYS);
There are three different Priority settings that you may select from, each determining whether or whether not the GUI component will grow if there is space available.
- Priority.ALWAYS
- Priority.SOMETIMES
- Priority.NEVER
You have to change many things while using Hgrow, including the Max Width properties of the GUI components in the window.
You will likely see no effect of Hgrow in the layout until you actually begin changing (increasing) the Max Width property on the GUI components.
Tip: The default “Preferred Width” for the VBox is automatically set to the Preferred Width of the largest GUI component.
Using CSS with HBox
A list of possible CSS styles that can be used with the JavaFX HBox layout component.
CSS Property | Description |
---|---|
-fx-padding | Minimum distance between the outermost GUI component and the edge of the HBox. |
-fx-border-style | Defines the type of border around the HBox. By default there is none. |
-fx-border-width | Defines the width of the border in pixels. |
-fx-border-insets | Sets the inset values for the border. |
-fx-border-color | Determines the Color of the border. |
-fx-border-radius | Determines the curve of the corners of the border. |
Other Alternatives
You can choose from a variety of different Layouts that JavaFX offers, such as “VBox” which is the polar opposite of HBox. You can also go for something with a more “Grid-like” pattern which arranges your widgets into rows and columns.
This marks the end of the JavaFX HBox tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.