This article covers the JavaFX TextArea widget.
Most GUI’s have multiple ways in which they may take input from the User. For JavaFX, the most common method is through the use of the TextField widget, which takes input in a single line, which is fine for most cases.
However, you may wish to take multi-line input from the user at certain points, such as a written paragraph. The JavaFX TextArea widget helps us accomplish this with it’s multi-line input ability.
You can import this class with the following name: import javafx.scene.control.TextField
.
JavaFX TextArea Example
To create a JavaFX TextArea widget, we simply need to create an object using it’s class and then add it to the layout we picked for our GUI.
package application;
import javafx.application.Application;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.control.TextArea;
import javafx.scene.layout.VBox;
public class Tutorial extends Application {
public static void main(String args[]){
launch(args);
}
@Override
public void start(Stage primaryStage) throws Exception {
VBox layout = new VBox();
Scene scene = new Scene(layout, 300, 300);
TextArea text = new TextArea();
layout.getChildren().addAll(text);
primaryStage.setTitle("CodersLegacy");
primaryStage.setScene(scene);
primaryStage.show();
}
}
Below is the GUI output of the above JavaFX TextArea example.
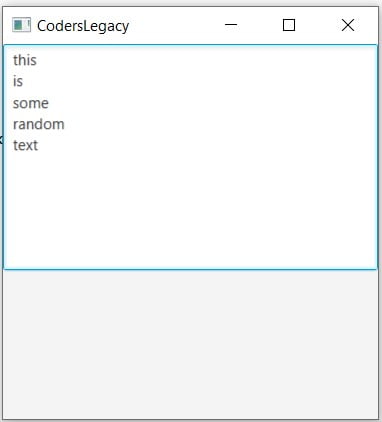
Retrieving TextArea values
Now that we’ve created the TextArea, it’s time to get the text from it that the user has entered.
To do we need the help of the getText()
method and the JavaFX Button widget. The getText()
method will “get” the text for us, but we need the button widget to trigger it.
package application;
import javafx.application.Application;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.TextArea;
import javafx.scene.layout.VBox;
public class Tutorial extends Application {
public static void main(String args[]){
launch(args);
}
@Override
public void start(Stage primaryStage) throws Exception {
VBox layout = new VBox();
Scene scene = new Scene(layout, 300, 300);
TextArea text = new TextArea();
text.setMaxWidth(200);
Button button = new Button("Click me");
button.setOnAction(e -> {
System.out.println(text.getText());
});
layout.getChildren().addAll(text, button);
primaryStage.setTitle("CodersLegacy");
primaryStage.setScene(scene);
primaryStage.show();
}
}
Below is the screen-shot of the GUI output side by side the console output that occurred when we clicked the button. Notice how even the text formatting was copied.
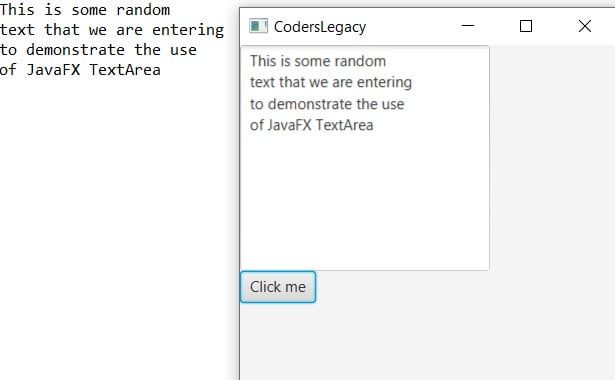
Also notice how we added an extra method there called setMaxWidth()
. You can find a description of it in the table below.
TextArea methods
List of a few useful methods available for the JavaFX TextArea. If you wish to change the size of the TextArea widget, you can use the size methods below.
Method | Description |
---|---|
getText() | Returns the currently entered text in the widget. |
setText() | Used to insert some text into the widget. |
setMaxWidth() | Sets the maximum possible width allowed for the TextArea. |
setMinWidth() | Sets the minimum possible width allowed for the TextArea. |
setMaxHeight() | Defines the maximum possible height allowed for the TextArea. |
setMinHeight() | Defines the minimum possible height allowed for the TextArea. |
This marks the end of the JavaFX TextArea article. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.