This article covers the TextField widget in JavaFX.
Every GUI has a way of taking input from the User. In JavaFX this is done through the use of the TextField widget. It’s a simple rectangular box which takes single line input from the User. It has an alternate version called TextArea which takes multi-line input.
At the end of this article you’ll find a list of methods and options available for the TextField widget.
Table of Contents
- Creating a TextField
- Retrieving TextField Values
- TextField Event-handlers
- TextField Methods
Creating a TextField
Your code for the TextField widget must include the following import in order to work: javafx.scene.control.TextField
.
To create a basic TextField, we simply have to create a TextField object and then add it to the layout of your choice.
package application;
import javafx.application.Application;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.control.TextField;
import javafx.scene.layout.StackPane;
public class Tutorial extends Application {
public static void main(String args[]){
launch(args);
}
@Override
public void start(Stage primaryStage) throws Exception {
StackPane layout = new StackPane();
Scene scene = new Scene(layout, 200, 200);
TextField text = new TextField();
layout.getChildren().addAll(text);
primaryStage.setTitle("CodersLegacy");
primaryStage.setScene(scene);
primaryStage.show();
}
}
Below is the output of the above code.
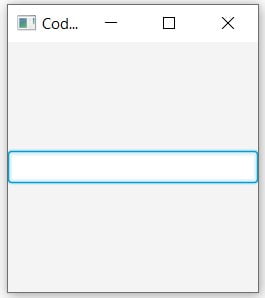
Retrieving a TextField value
We have successfully created a TextField widget, but currently we don’t have any way of actually knowing what the User typed in. And that’s a problem.
Using the getText()
method and a button widget, we will be able return the current text value of the TextField. (We’ll discuss a non-button method later in the article)
package application;
import javafx.application.Application;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.TextField;
import javafx.scene.layout.VBox;
public class Tutorial extends Application {
public static void main(String args[]){
launch(args);
}
@Override
public void start(Stage primaryStage) throws Exception {
VBox layout = new VBox();
Scene scene = new Scene(layout, 200, 200);
TextField text = new TextField();
text.setMaxWidth(100);
Button button = new Button("Print");
button.setOnAction(e -> {
System.out.println(text.getText());
});
layout.getChildren().addAll(text, button);
primaryStage.setTitle("CodersLegacy");
primaryStage.setScene(scene);
primaryStage.show();
}
}
Below is a screen-shot of the GUI next to the console output after pressing the button. Notice that the TextField is smaller this time. This is because we set a Maximum width in the code above.
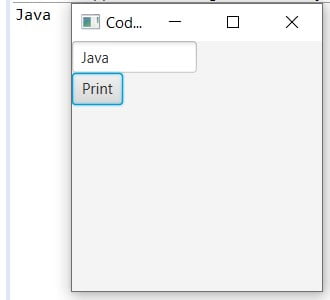
TextField – Event Handling
We’re going to make a very slight change. We’re going to remove the word “button” and replace it with “text” in the event handler function. Next, we remove all traces of the button widget, since we don’t need it anymore.
The result is that instead of the event handler being linked to the button, it’s now linked directly to the TextField itsefl.
package application;
import javafx.application.Application;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.control.TextField;
import javafx.scene.layout.VBox;
public class Tutorial extends Application {
public static void main(String args[]){
launch(args);
}
@Override
public void start(Stage primaryStage) throws Exception {
VBox layout = new VBox();
Scene scene = new Scene(layout, 200, 200);
TextField text = new TextField();
text.setMaxWidth(100);
text.setOnAction(e -> {
System.out.println(text.getText());
});
layout.getChildren().addAll(text);
primaryStage.setTitle("CodersLegacy");
primaryStage.setScene(scene);
primaryStage.show();
}
}
What’s changed now is that instead of pressing a button, we have to press the Enter key instead of the button.
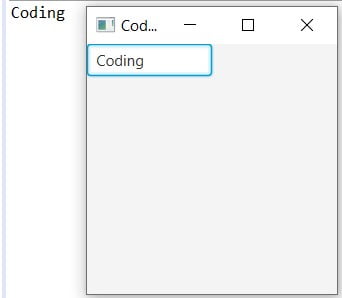
In the end, whether you use this method or button one, it’s all up-to you. Both of them are used widely, and sometimes even both are used together.
TextField Methods
A list of the different methods available for the TextField Widget.
Method | Description |
---|---|
setText(text) | Used to set the current Text value. |
getText() | Returns the current text value. |
setMaxWidth(n) | Sets the maximum possible value of the width. Measured in pixels. |
setMinWidth(n) | Sets the minimum possible value of the width. Measured in pixels. |
setAlignment(pos) | Used to set the text alignment of the text in the widget. Full list of positions available here. |
getAlignment() | Returns the current Alignment of the TextField. |
setOnAction(event) | Sets the value of the property onAction. |
getAction() | Gets the value of the property onAction. |
setPrefColumnCount(n) | Sets the preferred number of columns in the widget. Used to determine preferred width. |
getPrefColumnCount() | Returns the value of the preferred column count. |
To learn more about the various widgets offered by JavaFX, follow the link for more information.
This marks the end of the JavaFX TextField article. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.