Matplotlib by default only has a few different fonts available to us. If you try using a font like “Helvetica” for example, it will likely not be found and matplotlib will revert back to using the default font. In this tutorial we will discuss how we can add custom fonts to our matplotlib application.
There are several ways that we can add Custom Fonts in Matplotlib. Some of these techniques are OS specific and require to install the fonts into the OS you are using. But in today’s tutorial we will discuss a method that is not OS specific, neither does it require you to install the font.
Adding a Custom Font in matplotlib
For the purpose of this tutorial, I will be adding the font “Machine Gunk” to my matplotlib application. While we don’t have to install anything in this method, we still need the .ttf
font file. You can download any font file off the internet and use the below method to add it into matplotlib.
Here is a download link to the Machine Gunk Font that I will be using.
from matplotlib import font_manager
font_dirs = ['D:\VSCode_Programs\Python\matplotlib']
font_files = font_manager.findSystemFonts(fontpaths=font_dirs)
for font_file in font_files:
font_manager.fontManager.addfont(font_file)
The above code is responsible for adding the Machine Gunk font into our matplotlib application. The variable font_dirs
holds the filepath to a directory which contains the folder which holds the font files. Do not give it the file path of the actual font, or the file path of the directory in which the fonts are.
Inside 'D:\VSCode_Programs\Python\matplotlib'
, I have a folder called fonts
which holds a bunch of different .ttf font files, including the Machine Gunk Font.
Here is a complete example where we both add the font, and also use it in our application. You can learn more about the various ways for adding a font to matplotlib in a separate tutorial.
import matplotlib.pyplot as plt
from matplotlib import font_manager
import matplotlib
font_dirs = ['D:\VSCode_Programs\Python\matplotlib']
font_files = font_manager.findSystemFonts(fontpaths=font_dirs)
x = [0, 1, 2, 3, 4, 5]
y = [0, 6, 3, 8, 7, 5]
for font_file in font_files:
font_manager.fontManager.addfont(font_file)
plt.rcParams['font.family'] = 'Machine Gunk'
plt.rcParams['font.size'] = '14'
plt.title('title')
plt.xlabel('xlabel')
plt.ylabel('ylabel')
plt.plot(x, y)
plt.show()
The output of the above code:
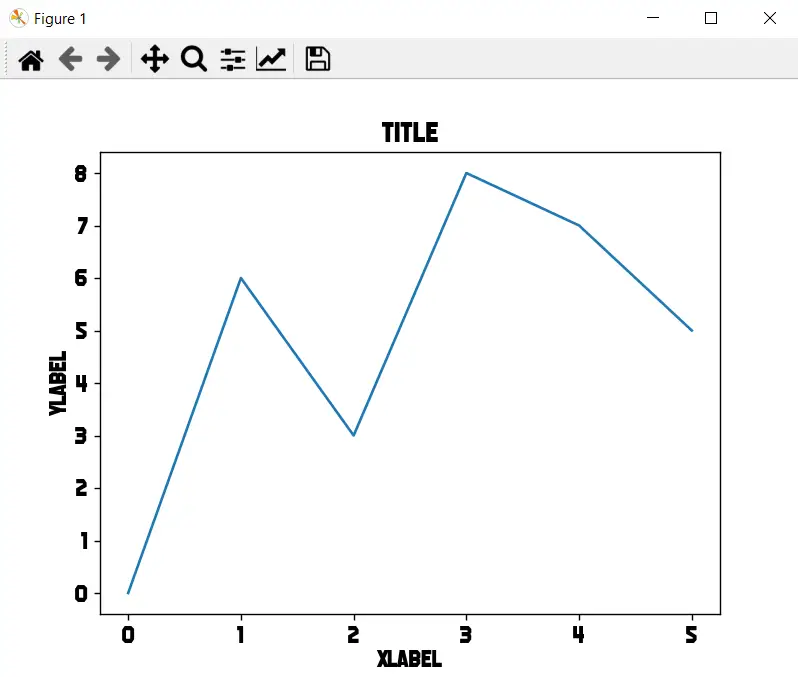
This marks the end of the Add Custom Fonts in Matplotlib Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.