Sometimes, Tkinter developers will need to keep a window on top of other windows, which is useful in many scenarios, such as when creating a pop-up or a notification window. You don’t want the pop-up being hidden behind the main window, now do you? In this article, we will discuss three methods to keep a Tkinter window on top, each with its own pros and cons.
Note: There is a small chance that some methods may not work on some operating systems. Which is why we have discussed so many. So if one doesn’t work, try the other!
Using wm_attributes
The first method to keep a Tkinter window on top is to use the wm_attributes
method. This method allows developers to set window attributes such as the window title, size, position, and the window’s state, including whether it is on top or not.
Here is an example code that demonstrates how to use the “top-most
” attribute to keep a Tkinter window on top. Setting the “top-most” attribute to “True
” places the window forcibly above all the others.
import tkinter as tk
root = tk.Tk()
root.title("Keep Window on Top")
root.geometry("300x300")
# Create a new window
top_window = tk.Toplevel(root)
top_window.title("Top Window")
top_window.geometry("200x200")
# Set the top_window on top of the root window
top_window.wm_attributes("-topmost", True)
root.mainloop()
You can pass in “False” instead of “True” to disable this behavior.
Sometimes just "attributes"
instead of "wm_attributes"
will also suffice, depending on your OS and tkinter version.
Using lift() to raise the Window
Another way is to use the lift() method. This method can “lift” a window above all other windows. However, does not “keep” your window above all the others, so if you select another window, then the first window will go behind the new one.
Nonetheless, this is a very useful method, that can be used together with the other two methods discussed here.
Here is an example.
import tkinter as tk
root = tk.Tk()
root.title("Keep Window on Top")
root.geometry("300x300")
def create_window():
# Create a new window
top_window.lift()
top_window = tk.Toplevel(root)
top_window.title("Top Window")
top_window.geometry("200x200")
tk.Button(root, text = "Create Window", command = create_window).pack()
root.mainloop()
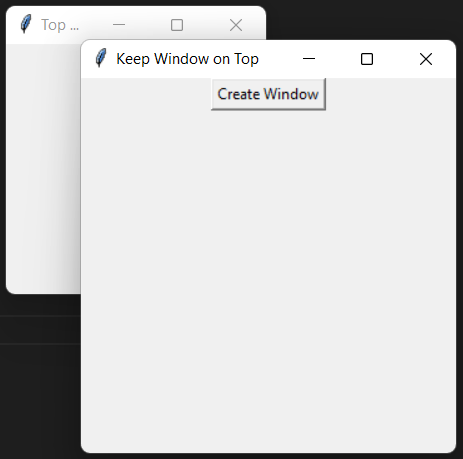
After clicking on the button, the window gets “lifted” to the top.
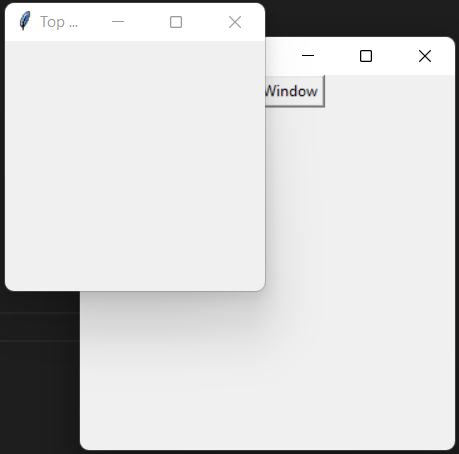
Using grab_set() to maintain position on top
Another interesting technique is to use the grab_set()
method. This is similar to what we did in method 1 with “topmost”, but is even more extreme.
Tkinter uses an event system for it’s windows, which detects mouse clicks and keyboard presses. Only the window currently being focused will receive any events. You can tell which window is being focused by observing the top navigation bar, with the title and buttons. If it is greyed out, then it not focused.
Grab_set()
forces tkinter to focus on a single window, and does not allow this focus to shift, until the window has been destroyed.
Here is an example. Trying playing around with it yourself to observe the effect.
import tkinter as tk
root = tk.Tk()
root.title("Keep Window on Top")
root.geometry("300x300")
def create_window():
# Create a new window
top_window = tk.Toplevel(root)
top_window.title("Top Window")
top_window.geometry("200x200")
top_window.lift()
top_window.grab_set()
tk.Button(root, text = "Create Window", command = create_window).pack()
root.mainloop()
To “release” the focus, use the grab_release()
method which will undo the effects of grab_set()
.
This marks the end of the How to keep a Tkinter Window on Top Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.