In this Pygame tutorial we will explore how to “Resize” an Image in Pygame. There are several re-sizing and scaling functions in Pygame that we can use to resize images, each with it’s own unique twist. Let’s begin exploring the right away!
Before we begin resizing an image however, we first need to load it into our Pygame application. This can be done using the image.load()
function as shown below.
image = pygame.image.load("D:\Programs\pygame\Player.png")
Now that we have our image object, let’s explore how we can change it’s dimensions.
Resize an Image in Pygame
We will be using the following image for our tutorial.
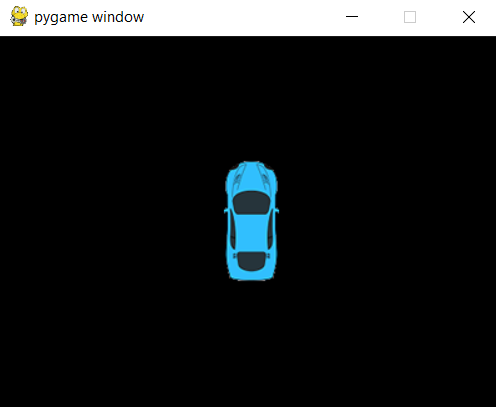
Before we begin resizing an image, it’s a good idea to acquire it’s dimensions before hand. We can do this using the get_rect()
method, which returns a Rect object with width and height attributes.
width = image.get_rect().width
height = image.get_rect().height
Now that we have the width and the height, resizing becomes easier. This is because we can simply “scale” the image using it’s base dimensions.
For example, if we want to double the size of the image, all we have to do is multiply the width and height by 2 and pass these as parameters to the pygame.transform.scale()
function.
image = pygame.transform.scale(image, (width*2, height*2))
It is important to note that these functions are not “in-place” and do not modify the image object directly. Instead they will return a new surface object which we can either pass into a new variable or update the old one.
Here is the complete version with all the supporting code:
import pygame
import sys
pygame.init()
screen = pygame.display.set_mode((400, 300))
image = pygame.image.load("D:\Programs\pygame\Player.png")
width = image.get_rect().width
height = image.get_rect().height
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
image = pygame.transform.scale(image, (width*2, height*2))
screen.blit(image, (180, 100))
pygame.display.update()
Here we can see the size of image has doubled.
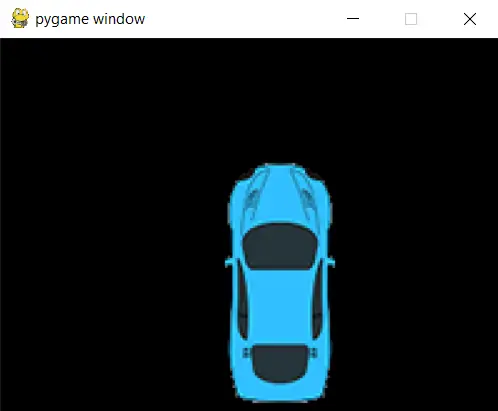
Now let’s try the reverse. Let us scale it down to 1/2 of it’s size. It is important to pass only integers to the scale()
function, hence we wrapped the values in the int()
function.
image = pygame.transform.scale(image, (int(width*0.5), int(height*0.5)))
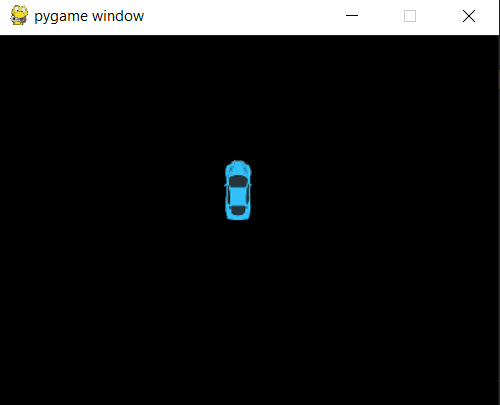
We can also pass in any values of our own into the second parameter of scale()
. We may wish to do this with images where aren’t too worried about maintaining the original aspect ratio.
image = pygame.transform.scale(image, (60, 100))
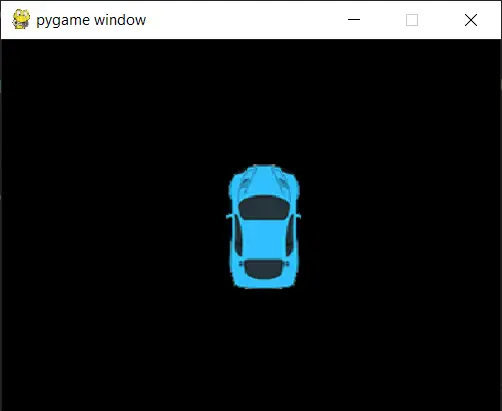
Scaling with smoothscale()
Another function that we can use for re-sizing is pygame.transform.smoothscale()
. This function is almost identical to pygame.transform.scale()
, but with one difference in how it works.
Normally when you scale images up, this can result in a rather “jagged” image as the pixels become more clear. The smoothscale()
function minimizes this problem by “blurring” the edges of the image to give it a smoother look.
The syntax otherwise remains the exact same.
image = pygame.transform.smoothscale(image, (width*2, height*2))
This marks the end of the How to resize an image in Pygame Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.