From the Python Tkinter Library, messagebox is used to display a variety of prompts to the user. Messagebox is useful due to it’s numerous types of prompts and the user friendly way it displays them to the user.
Keep in mind, that Python messagebox goes hand in hand with the GUI library tktinter. A tkinter gui window is required for prompt to spawn. Hence, even if you don’t declare one yourself python will automatically create one during execution. If you only want the prompt to be showing however, we will explore some ways you may do so.
Importing messagebox
The messagebox module comes bundled with the tkinter library, so if you have the tkinter library installed, you’re all set. Just use the following import statement to bring the messagebox module into your code.
from tkinter import messagebox
Types of Message Boxes
The messagebox module introduces several types of prompts that we may present, such as confirmation messages, warning messages and information messages.
messagebox.showinfo()
A very simple type of prompt that simply displays text to the user. Comes with an “i” for information sign embedded on the prompt.
from tkinter import messagebox
prompt = messagebox.showinfo(title = "Info",
message = "Certain information")
messagebox.showwarning()
Identical to the messagebox.showinfo()
function but it comes with a warning sign embedded onto the prompt. Used to give the user a sense of urgency regarding the information in the message.
from tkinter import messagebox
prompt = messagebox.showwarning(title = "Warning!",
message = "Warning, errors may occur")
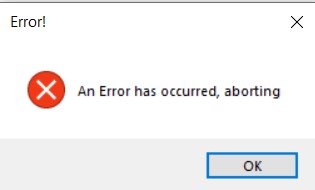
messagebox.showerror()
Identical to the messagebox.showinfo()
function but it comes with a error sign embedded onto the prompt. Gives the impression that an error has occurred.
from tkinter import messagebox
prompt = messagebox.showerror(title = "Error!",
message = "An Error has occurred, aborting")
messagebox.askquestion()
The messagebox.askquestion()
function asks the user a question giving them the option of saying yes or no. Depending on their answer, “yes” and “no” strings are returned.
from tkinter import messagebox
prompt = messagebox.askquestion(title = "New file",
message = "Save changes?")
messagebox.askyesno()
The messagebox.askyesno()
function outputs a message to the user giving them the option of saying yes or no. Depending on their answer, Boolean values True
and False
are returned.
prompt = messagebox.askyesno(title = "Confirm",
message = "Do you wish to continue")
messagebox.askyesnocancel()
The messagebox.askyesnocancel()
function outputs a message to the user giving them three options, yes, no and cancel. Depending on their answer, Boolean values True
, False
and None
are returned.
prompt = messagebox.askyesnocancel(title = "Confirm",
message = "Do you wish to continue")
messagebox.askokcancel()
The messagebox.askokcancel()
function outputs a message to the user giving them the option of saying yes or cancelling the prompt. Depending on their answer, Boolean values True
and False
are returned.
prompt = messagebox.askokcancel(title = "Confirm",
message = "Do you wish to continue")
messagebox.askretrycancel()
The messagebox.askretrycancel()
function outputs a message to the user giving them the option of retrying or cancelling the prompt. Depending on their answer, Boolean values True
and False
are returned.
prompt = messagebox.askretrycancel(title = "Confirm",
message = "Do you wish to try again")
Displaying messagebox without Tkinter Window
You cannot display a messagebox without a Tkinter window being created. However, you can hide it right after it’s created, thus giving the illusion that it was never created in the first place.
import tkinter as tk
from tkinter import messagebox
root = tk.Tk()
root.withdraw()
prompt = messagebox.showerror(title = "Error!",
message = "An Error has occurred, aborting")
No need to worry about it appearing as a minimized window either. It will be entirely hidden, and can only be displayed again through code.
This marks the end of the Python Tkinter Messagebox Article. Any suggestions or contributions for CodersLegacy are more than welcome at any time. Questions can be asked below in the comments section.
Here’s a link to the main Python Libraries Article: link
very useful