This tutorial explains how to crop an Image in Python Pillow.
Although resizing images can solve most of our image size related issues, sometimes we instead need to remove certain parts of the image, also known as “cropping”. The Pillow library gives us the crop()
function which allows us to crop images easily in Python.
Cropping with Pillow
The Pillow crop()
function takes 4 different parameters, left
, right
, top
and bottom
. Each of the parameters represent the coordinates for the cropped image.
The way the Pillow coordinate system works is that the top-left corner has the coordinates (0, 0) and the bottom-right has the coordinates (width, height). As you can see, the coordinates increase from top to bottom and left to right.
from PIL import Image
img = Image.open("C://Users/Shado/Desktop/kitten.jpg")
left = 200
right = 600
top = 100
bottom = 500
new_img = img.crop((left, top, right, bottom))
new_img.show()
Here’s the original Image, before we cropped it.
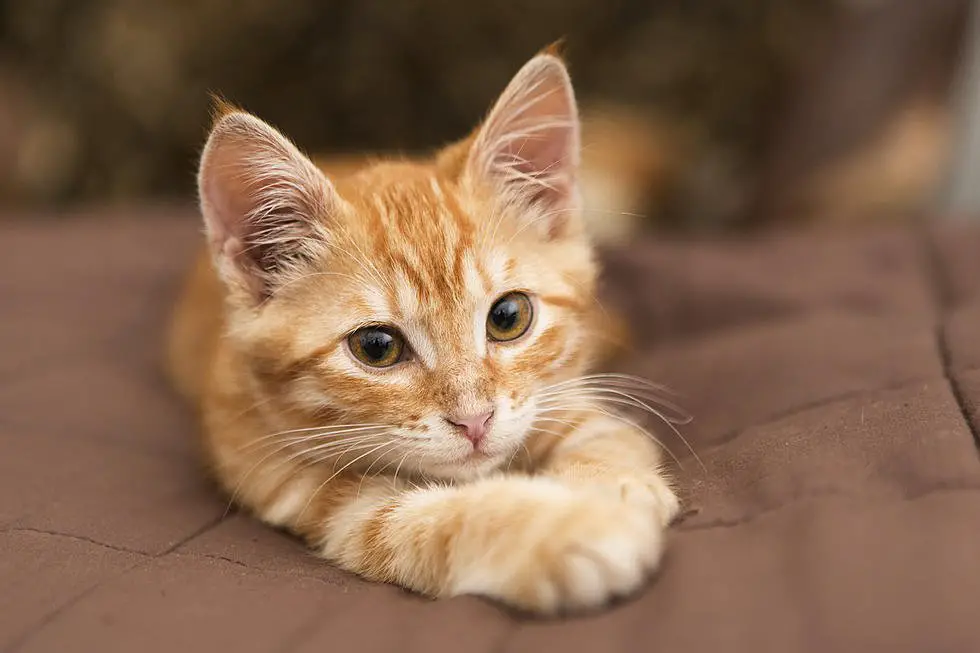
This is the image after we’ve cropped it.
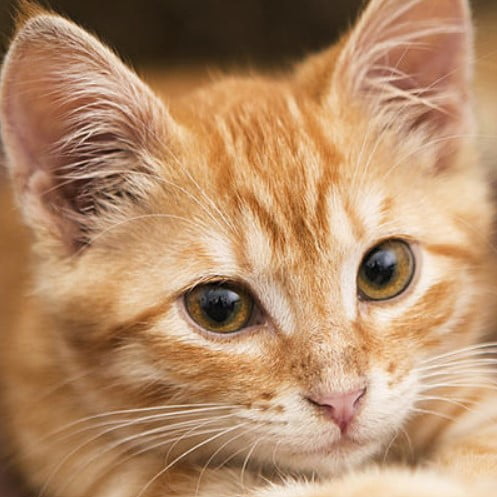
As a general rule of advice while cropping, the right parameter is always gonna be greater than the left, and the bottom is always going to be greater than the top. This is because the left and top both start at the respective 0th x and y coordinate.
In simpler words, the top left corner is the (0, 0) coordinate. And the x-coordinate grows as you move left-to-right, and the y-coordinate grows as you move from top-to-bottom.
There are many different things we can do once we have successfully read an image. Check out some of our other Pillow Guides to learn more about image processing in Python!
This marks the end of the Pillow Image Crop tutorial. Any suggestions or contributions for CodersLegacy are more than welcome Questions regarding the article content can be asked in the comments section below.