This tutorial explains how to add blur an image in Pillow
The Python pillow library provides us with a variety of different functions and settings with which we may apply various filters on an image to create a blur effect. The blur effect is an important part of image processing and is commonly used.
Simple ImageFilter Blur
All of the blur effects that we’re going to discuss here are done with the use of the ImageFilter feature in Pillow. The simplest blur is applied by using the ImageFilter in it’s base form, shown in the example below.
from PIL import Image, ImageFilter
img = Image.open("C://Users/CodersLegacy/Desktop/kitten.jpg")
blurred_img = img.filter(ImageFilter.BLUR)
blurred_img.show()
Be sure to include the Pillow ImageFilter import as well.
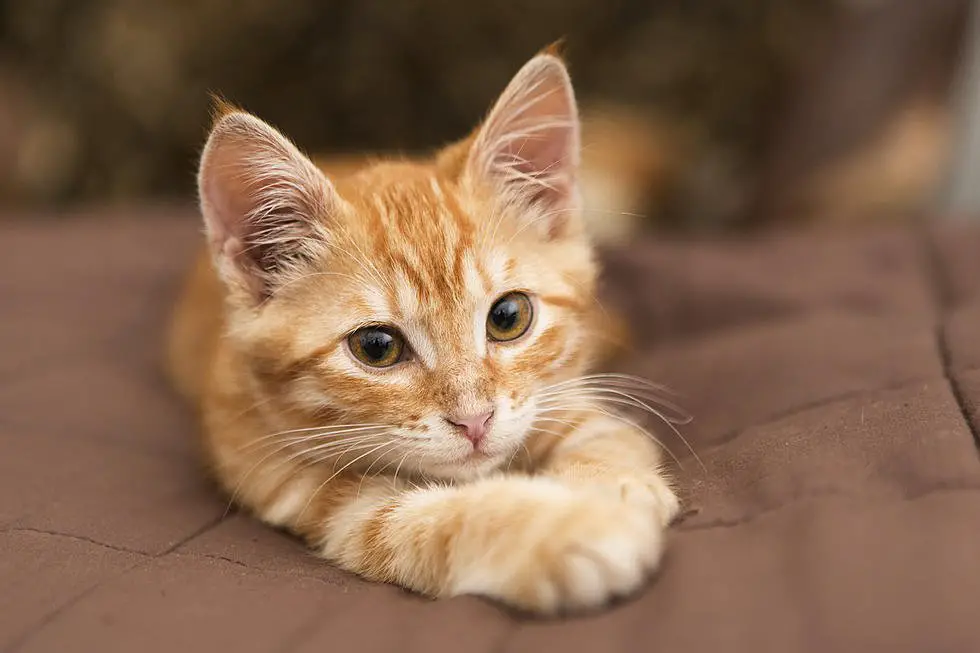
The output:
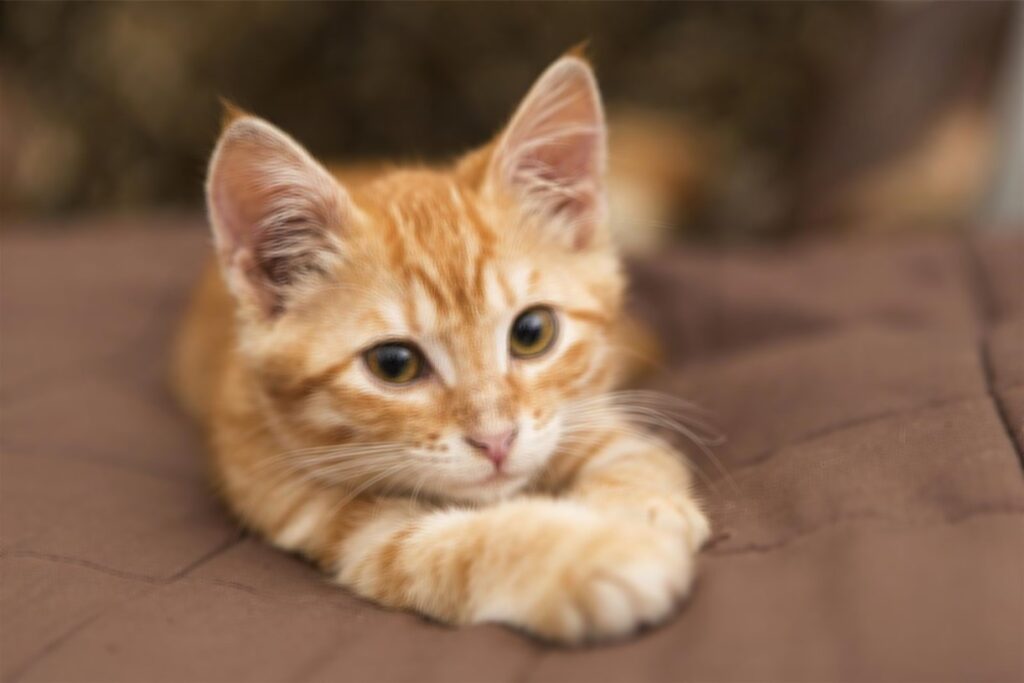
One reason why this is probably called the simple blur is because you can’t customize how much the image is blurred. Either you apply the blur or you don’t.
Pillow Box Blur
The next type of blur is the box blur which takes a radius value to determine the amount of blurring to be done. The higher the value, the more the blur effect will be applied on the image.
from PIL import Image, ImageFilter
img = Image.open("C://Users/CodersLegacy/Desktop/kitten.jpg")
blurred_img = img.filter(ImageFilter.BoxBlur(20))
blurred_img.show()
The output:
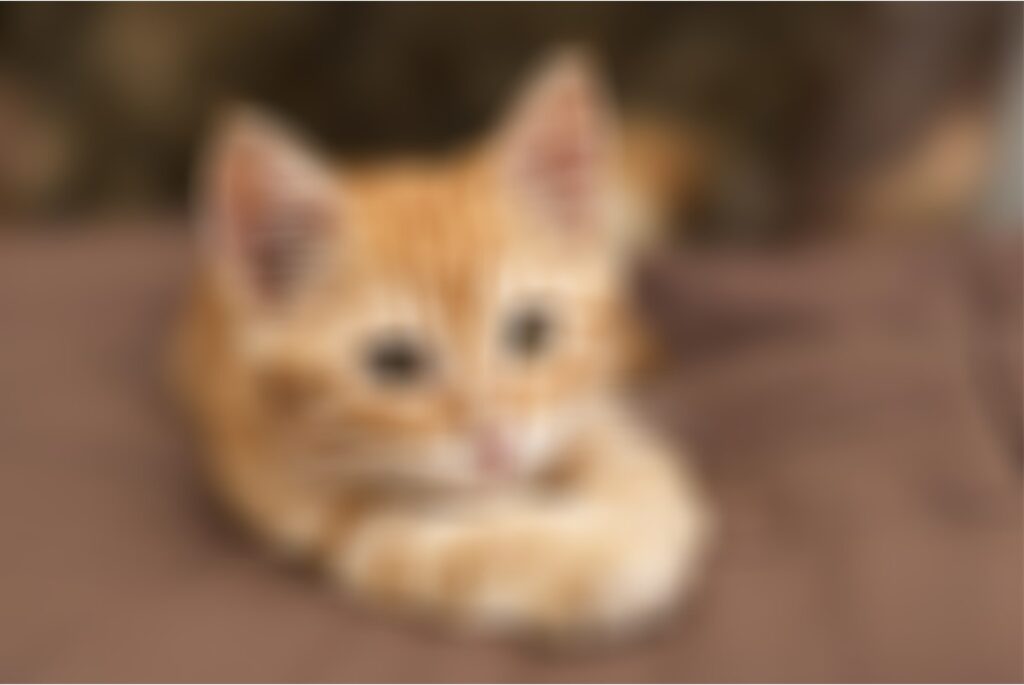
We have applied strong blurring here, hence why the image is so blurred out now. We’ll explore a wider range of blur values in the next section.
Pillow Gaussian Blur
The Gaussian Blur is another type of blur which, like the box blur uses a radius value to apply a blur onto am image. It uses a slightly different algorithm called the Gaussian function, to determine the blur amount.
from PIL import Image, ImageFilter
img = Image.open("C://Users/CodersLegacy/Desktop/kitten.jpg")
blurred_img = img.filter(ImageFilter.GaussianBlur())
blurred_img.show()
The output: (With the default Gauss Blur)
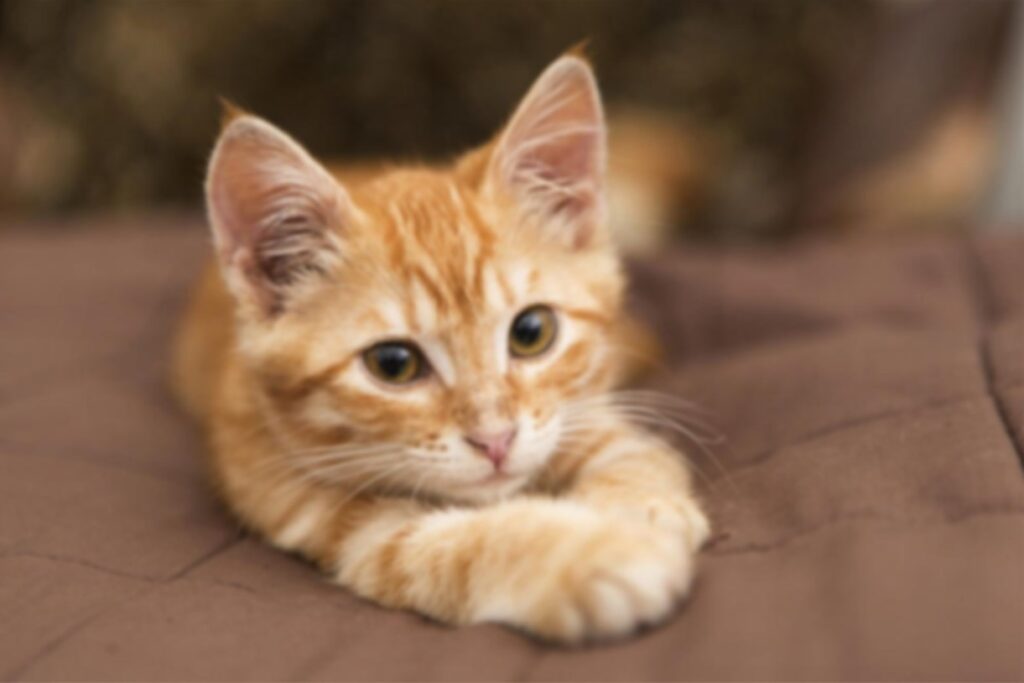
Increasing the blur to a radius of 20:
blurred_img = img.filter(ImageFilter.GaussianBlur(20))
blurred_img.show()
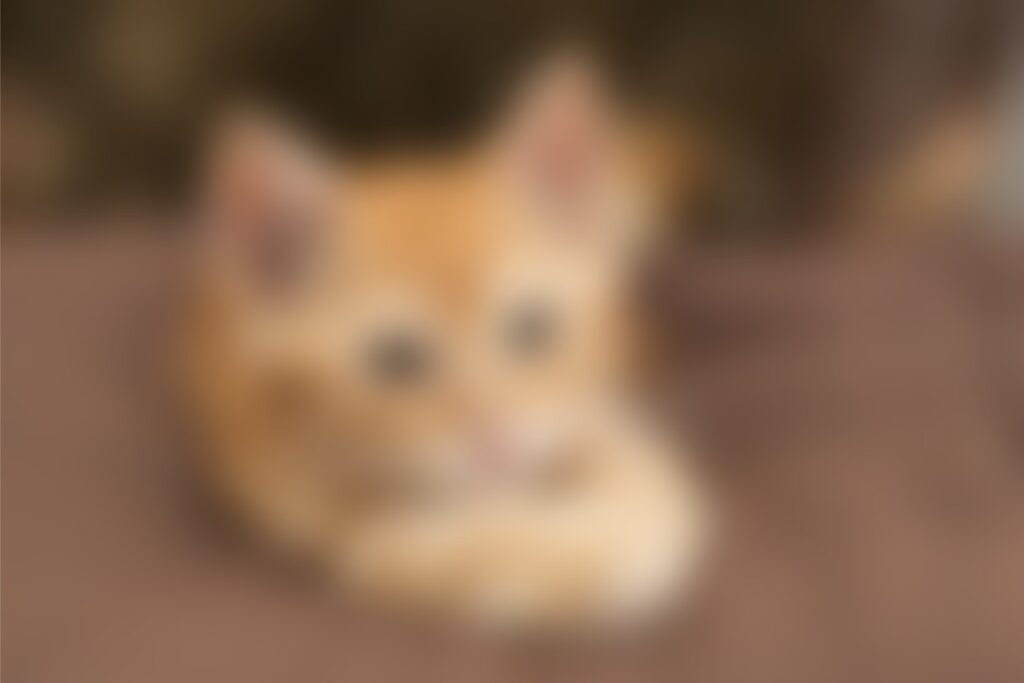
On a side note, compare it to the box blur at radius 20 to see the slight difference. The Gauss Blur appears to have a applied a more smoother blur than the box blur, giving a better effect.
Increasing the blur to a radius of 50:
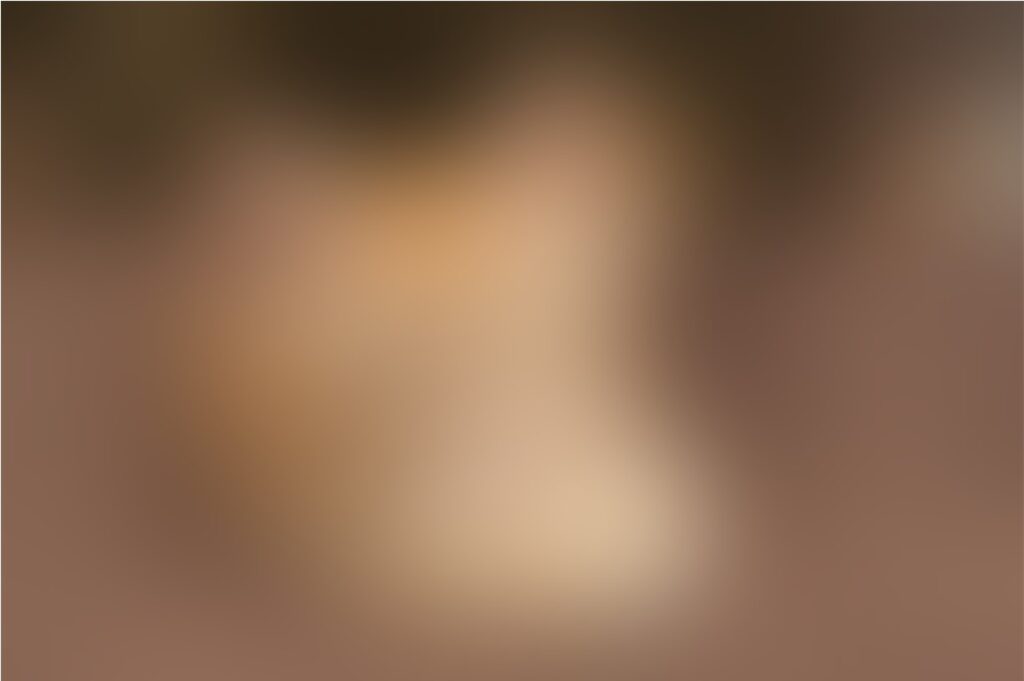
We can see that increasing the value of the Gaussian beyond 20 makes the image blurry to a point where it can not be identified.
This marks the end of the Python Pillow – Image Blur Effect Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.