In this tutorial we will discuss the ImageTk module within the Pillow Library and how it can be used with Tkinter.
Tkinter is a powerful library in Python used to create GUI applications, complete with all kinds of widgets and features. One key component is making good GUI’s is the inclusion of Images. In order to display images in Tkinter, we need to have a way of reading them and converting them to python objects that can be used in our code.
Tkinter has it’s own built-in module, called PhotoImage which can be used for this purpose. However, PhotoImage is a little limited by the fact it doesn’t recognize many image formats. The Pillow Image Library on the other hand is a good alternative.
Pillow ImageTK
Pillow is primarily an Image Processing library, rather than a part of Tkinter. It’s features include image filtering and image-related operations such as cropping, rotating, blurring etc. However, it has a module within it called ImageTk that can be used to load images from files in a format that is compatible with Tkinter.
Pillow being the large and powerful library that it is, can read more image formats than Tkinter’s PhotoImage. Let’s take a look at how to use this library!
pip install pillow
The first step is to download and install pillow if you haven’t. The name of the library itself is “pillow”, so if you are using pip to install it, the command would be “pip install pillow”.
Importing it however, is done a bit differently, requiring you to import from PIL, not pillow.
from PIL import Image, ImageTk
The Image Class in PIL is the regular Image object used, whereas ImageTk is the one that is compatible with Tkinter.
Displaying an Image
self.img = Image.open("M_Cat_Loaf.jpeg")
The first thing to do is use the Image.open() function to load an image, using a filepath. (Remember to use the full filepath instead of the name, if the image is in a different directory)
self.img = self.img.resize((320, 300), Image.ANTIALIAS)
Next we need resize the image a little. This is because the original image is pretty large, and not suitable for displaying on a small window. The resize()
function is one of the many functions that can be used on Pillow Image Objects. (Remember to do all processing before converting to an ImageTk object, as ImageTk Objects do not have the same functions available)
The Image.ANTIALIAS
defines the sampling mode used while resizing.
self.img = ImageTk.PhotoImage(self.img)
This here converts the Image object into a Tkinter PhotoImage object. Now we can use this in tkinter widgets.
label = tk.Label(master, image = self.img)
Many widgets have the image option, such as labels and buttons, allowing them to display images.
The full code:
import tkinter as tk
from PIL import Image, ImageTk
class Window:
def __init__(self, master):
self.img = Image.open("M_Cat_Loaf.jpeg")
self.img = self.img.resize((320, 300), Image.ANTIALIAS)
self.img = ImageTk.PhotoImage(self.img)
label = tk.Label(master, image = self.img)
label.pack(expand = True, fill = tk.BOTH)
root = tk.Tk()
window = Window(root)
root.mainloop()
The output:
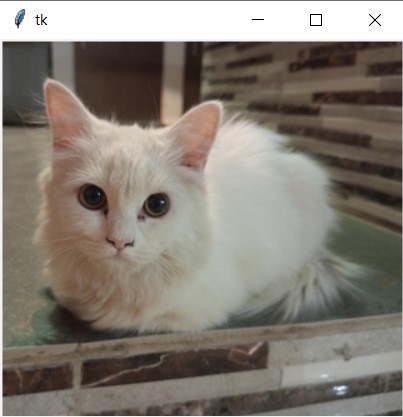
This marks the end of the Pillow ImageTk with Tkinter Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.
Cute catto
Wow !! that was so clear , Really appreciate YOU :))) <3+99
only one Question :
could you please explain why ?