This tutorial explains how to resize an image using Python Pillow.
The Pillow Imaging Library allows us to easily change or “re-size” the dimensions of an image through the use of the “resize” function.
Resize in Pillow
Here is us normally reading the image in Pillow and displaying it.
from PIL import Image
img = Image.open("C://Users/Shado/Desktop/kitten.jpg")
img.show()
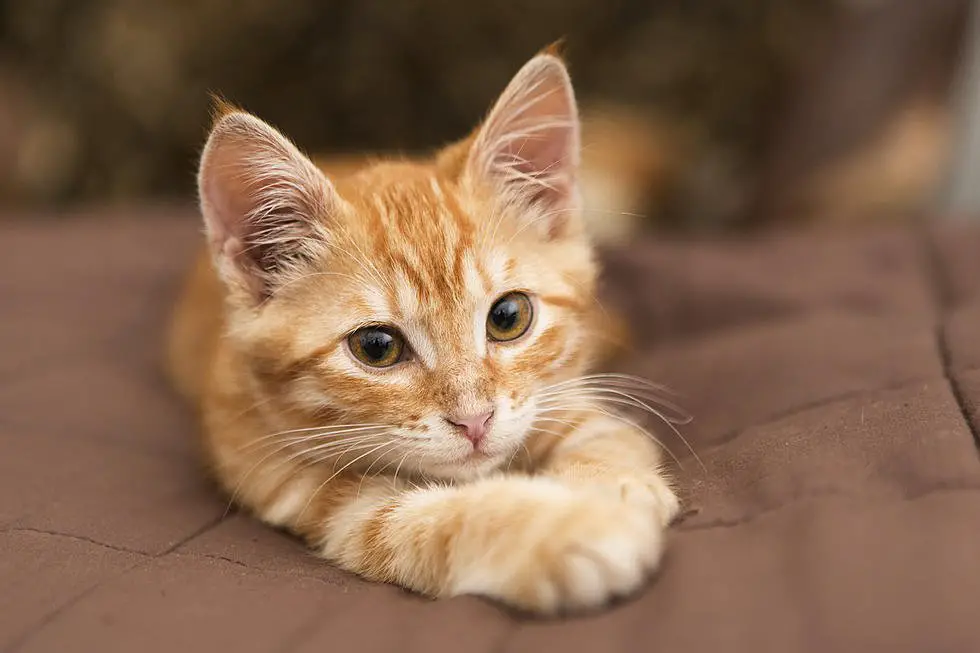
Here we are opening the same image, but using the resize()
function on it. However, the resize()
function does not change the image itself. It actually returns a new image object. You can either reassign this new object to the same image to overwrite it, or create a new one like we did below.
from PIL import Image
img = Image.open("C://Users/Shado/Desktop/kitten.jpg")
new_img = img.resize((500, 300))
new_img.show()
We passed 500 (the width) and 300 (the height) into the resize() function as a Tuple (a pair of values). This gives us the following image, which is clearly smaller than the previous one.
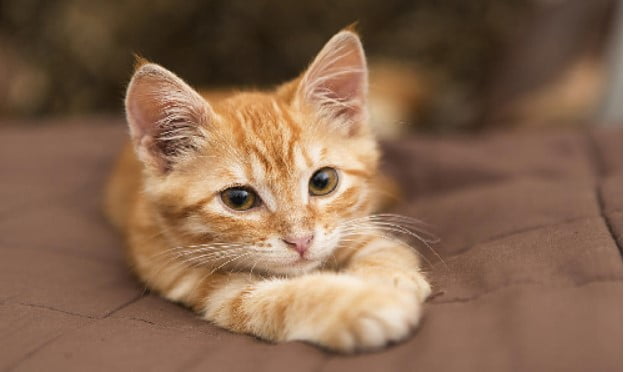
Maintaining Aspect Ratio in Pillow
Previously we tried to roughly half the dimensions of the image based of an approximation, if you look closely the aspect ratio of the resized image is a bit different. This results in some slight distortion, which ruins the quality. A simple way to maintain aspect ratio is to multiply both dimensions with the same value or fraction.
In the below example we have perfectly halved the dimensions by directly calling upon the width and size attributes for the image, and multiplying them by 0.5
.
from PIL import Image
img = Image.open("C://Users/Shado/Desktop/kitten.jpg")
new_img = img.resize((round(img.width * 0.5), round(img.height * 0.5)))
new_img.show()
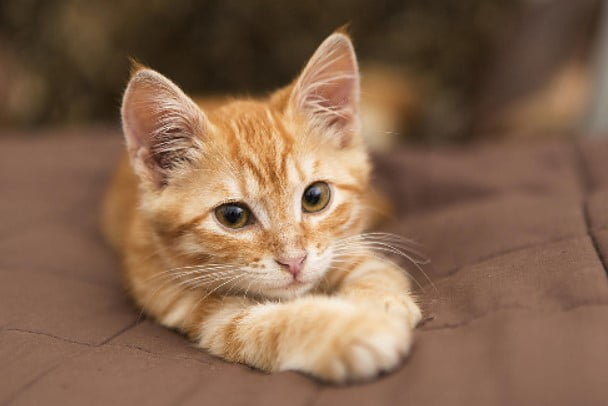
The image you see now has the same aspect ratio as the original.
As an alternative, you can divide both dimensions by each other to get a “ratio” which you can then use to ensure the aspect ratio in the image is maintained.
This marks the end of the Image Resize with Pillow Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.