This tutorial explains how to rotate images in Python Pillow
The Pillow library allows us to easily rotate and display images in Python, while also providing us with extra functionality to make sure the original quality of the image is retained during the rotation.
Image Rotation
In this section we’re going to try rotating images by different angles and checking the corresponding output. We’ll start off by using the rotate()
function to rotate the image 45 degrees.
from PIL import Image
img = Image.open("C://Users/Shado/Desktop/kitten.jpg")
new_img = img.rotate(45)
new_img.show()
The output:
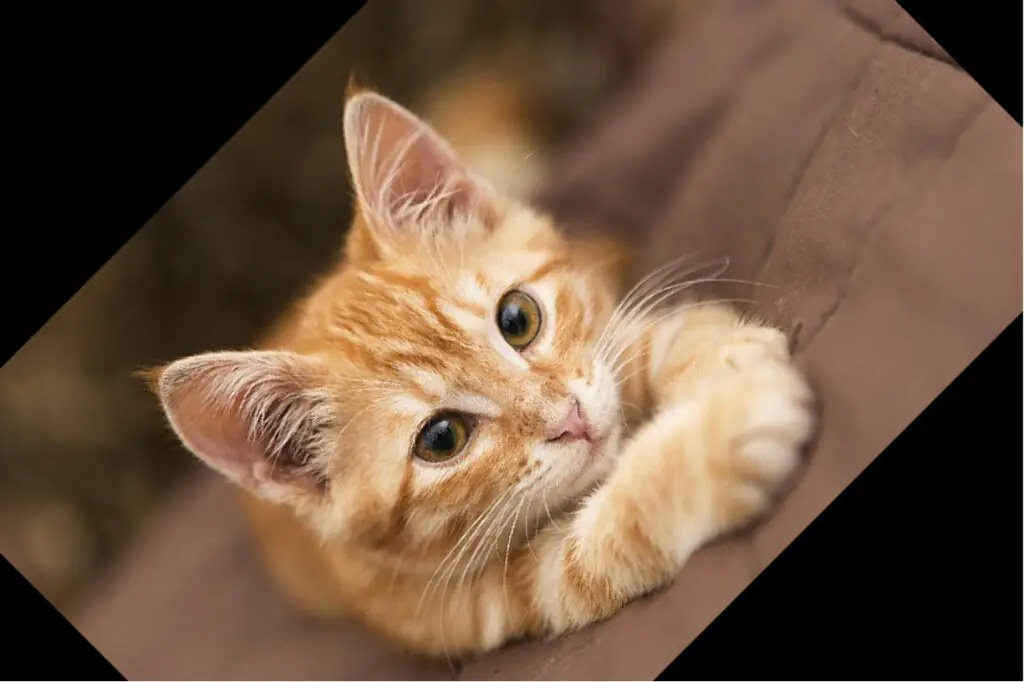
Now let’s try the rotation again, but with a different angle.
from PIL import Image
img = Image.open("C://Users/Shado/Desktop/kitten.jpg")
new_img = img.rotate(90)
new_img.show()
The output:
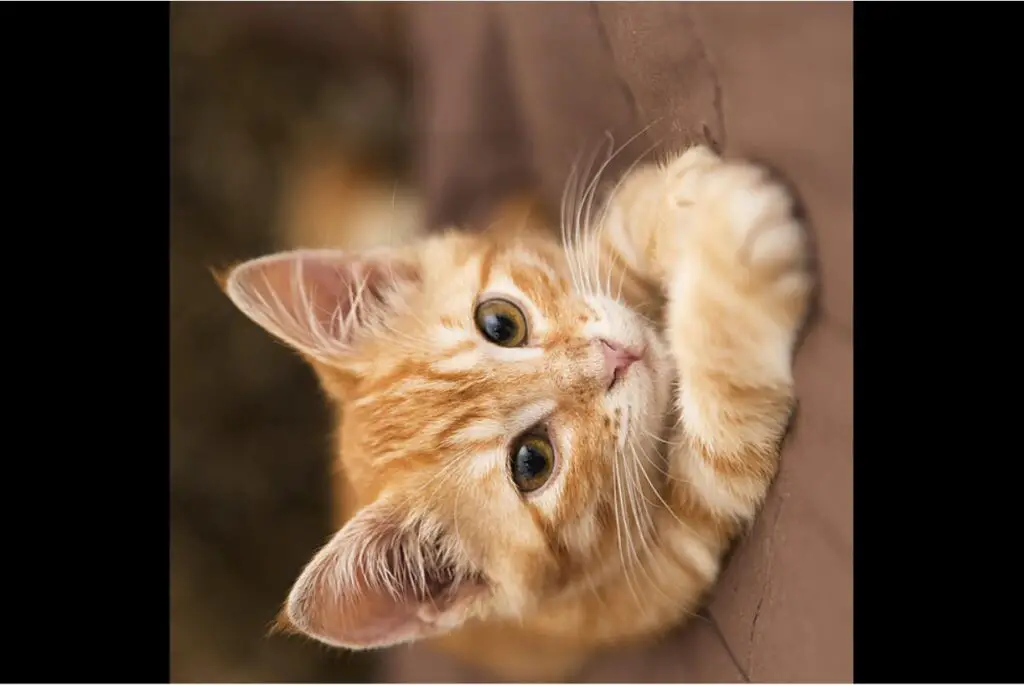
Have you been noticed that certain parts of the image have been removed? And that there are now black areas in the pictures? We’ll be fixing this in the next section.
Image Rotate with Expand
What expand function basically does is to swap the height and width dimensions. This is important because if you rotate an image, the height and width boundaries due not change. This results in some of the image being cropped off and areas of black where the image no longer resides.
You just have to pass in the value 1
to turn the expand setting on.
from PIL import Image
img = Image.open("C://Users/Shado/Desktop/kitten.jpg")
new_img = img.rotate(90, expand = 1)
new_img.show()
Now let’s take at the new output of the image.
We can see that the black edges are completely gone, and we can see the full image, as compared to the previous one where certain parts were cropped out.
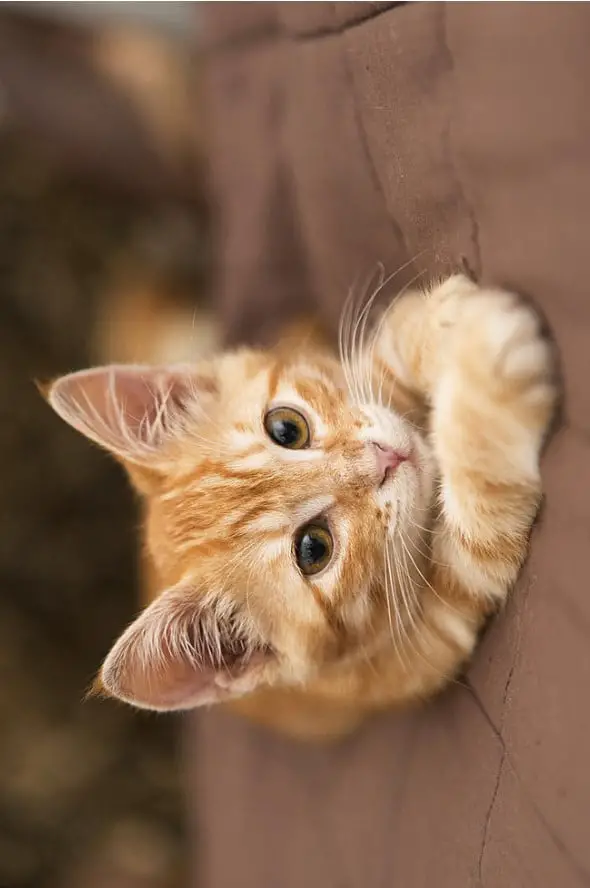
This doesn’t always work so perfectly unfortunately. In the case that the image is rotated 45 degrees, it will recover the full image but the black areas will not be removed entirely.
There are many different things we can do once we have successfully read an image. Check out some of our other Pillow Guides to learn more about image processing in Python!
This marks the end of the Pillow Image Rotate Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.