Tkinter actually has a variety of ways in which we may change the font type and size. Tkinter has several built in fonts, which can complicate things, especially when you realize that Each widget only uses one of these fonts. However, this also gives us the option to individually change the font type and size for different types of widgets.
We’ll start off with a general way of changing the font size and type that effects everything in the tkinter window.
Technique 1
The following code will only change the Font.
import tkinter as tk
root = tk.Tk()
root.option_add('*Font', '19')
root.geometry("200x150")
label = tk.Label(root, text = "Hello World")
label.pack(padx = 5, pady = 5)
root.mainloop()
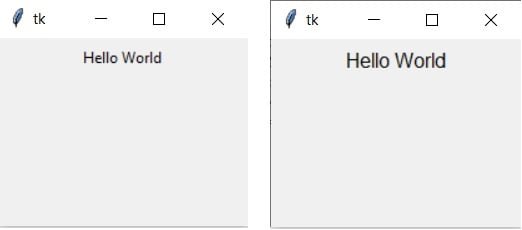
The following code changes only the font type. Tip:
import tkinter as tk
root = tk.Tk()
root.option_add('*Font', 'Times')
root.geometry("200x150")
label = tk.Label(root, text = "Hello World")
label.pack(padx = 5, pady = 5)
root.mainloop()
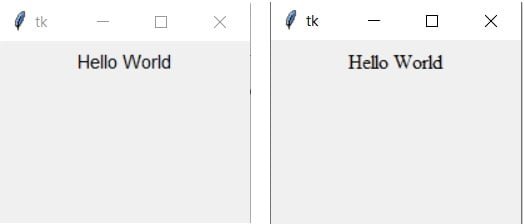
Tip: If you want a list of font families, you can use the following code. It will return a list of different font types.
from tkinter import Tk, font
root = Tk()
print(font.families())
Finally, you can change both simultaneously by writing both at the same time.
import tkinter as tk
root = tk.Tk()
root.option_add('*Font', 'Times 19')
root.geometry("200x150")
label = tk.Label(root, text = "Hello World")
label.pack(padx = 5, pady = 5)
root.mainloop()
Note, however, that option_add
only affects widgets created after you’ve called option_add
, so you need to do it before creating any other widgets.
Technique 2
Here narrow down our area of effect from the whole tkinter program to certain segments of it. As mentioned earlier, tkinter has several built in fonts which different widgets use. We can change each one of these individually.
import tkinter as tk
import tkinter.font as tkFont
root = tk.Tk()
print(tkFont.names())
('fixed', 'oemfixed', 'TkDefaultFont', 'TkMenuFont', 'ansifixed', 'systemfixed', 'TkHeadingFont', 'device', 'TkTooltipFont', 'defaultgui', 'TkTextFont', 'ansi', 'TkCaptionFont', 'system', 'TkSmallCaptionFont', 'TkFixedFont', 'TkIconFont')
Above we can notice several font types like TkHeadingFont
and TkMenuFont
. These appear to be the fonts that determine the font type and size for headings and Menu’s respectively. Let’s try to change the TkDefaultFont
. Any widget that uses the TkDefaultFont
type will automatically change to the new settings.
import tkinter as tk
import tkinter.font as tkFont
root = tk.Tk()
root.geometry("200x150")
def_font = tk.font.nametofont("TkDefaultFont")
def_font.config(size=24)
label = tk.Label(root, text = "Hello World")
label.pack(padx = 5, pady = 5)
root.mainloop()
The tricky part however is figuring out which widgets are affected by which Font types. Due to a lack of material, this information is currently unavailable. Though you are free to search for it on your own.
This marks the end of the change font type and size in Tkinter article. Any suggestions or contributions for CodersLegacy are more than welcome. Any questions can be asked in the comments section below.
See more Tkinter related tit bits below.
How to improve Tkinter screen resolution?
How to prevent resizing in Tkinter Windows?
Using Lambda with Tkinter “command” option