In this Pygame Tutorial we will be discussing how to use the “draw” module for drawing shapes to our Pygame Window. This module is essentially a collection of various drawing functions, each used to draw a unique shape or object.
Drawing Functions in Pygame
Here is a list of all the drawing functions available to us in Pygame.
- pygame.draw.polygon(surface, color, pointlist, width)
- pygame.draw.line(surface, color, start_point, end_point, width)
- pygame.draw.lines(surface, color, closed, pointlist, width)
- pygame.draw.circle(surface, color, center_point, radius, width)
- pygame.draw.ellipse(surface, color, bounding_rectangle, width)
- pygame.draw.rect(surface, color, rectangle_tuple, width)
We will now proceed to discuss each one of these one by one, followed by an example + output.
Drawing Lines in Pygame
pygame.draw.line(surface, color, start_point, end_point, width=1)
- surface: Window object to which we want to draw our Shape.
- color: Color of the drawn shape.
- start_point: A tuple/list consisting of an x and y value pair, which defines the starting position of where the line will be drawn.
- end_point: A tuple/list consisting of an x and y value pair, which defines the ending position of where the line will be drawn.
- width: Default value of 1. If width < 1, then the line will not be drawn. Values above 1 will increase the line thickness.
import pygame
import sys
pygame.init()
display = pygame.display.set_mode((300, 300))
display.fill((255, 255, 255))
pygame.draw.line(display, (0, 0, 255),
(10,10),(100,100), width=2)
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
pygame.display.update()
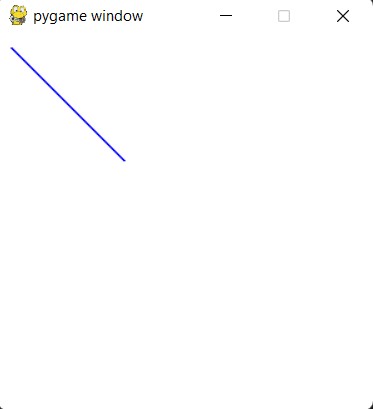
We can also use the lines()
function instead, if we have to draw many lines at the same time.
pygame.draw.lines(surface, color, closed, pointlist, width=1)
- surface: Window object to which we want to draw our Shape.
- color: Color of the drawn shape.
- closed: Specifies whether the first and last points have a line between them (
False
by default) - pointlist: A list of x-y coordinate pairs, where each adjacent pair is connected to the other with a line.
- width: Default value of 1. If width < 1, then the line will not be drawn. Values above 1 will increase the line thickness.
import pygame
import sys
pygame.init()
display = pygame.display.set_mode((300, 300))
display.fill((255, 255, 255))
pygame.draw.lines(display, (0, 0, 255), closed=True,
points= [(20,20), (60,120), (200, 180)],
width=2)
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
pygame.display.update()
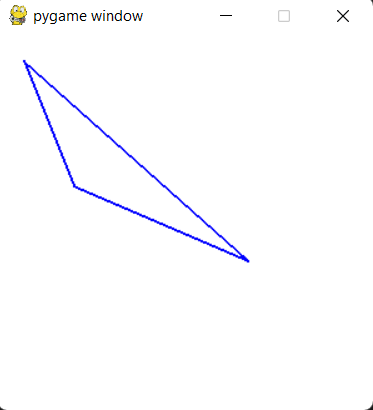
Drawing Polygons in Pygame
pygame.draw.polygon(surface, color, pointlist, width=0)
- surface: Window object to which we want to draw our Shape.
- color: Color of the drawn shape.
- The pointlist parameter is a tuple containing co-ordinates or “points”. For instance, for a rectangle you will pass a tuple with 4 co-ordinate pairs inside it. (First and last point are connected automatically)
- width: Default value of 0, which means polygon will be filled (color). Values above 0 will make it unfilled, and width will control border thickness.
import pygame
import sys
pygame.init()
display = pygame.display.set_mode((300, 300))
display.fill((255, 255, 255))
pygame.draw.polygon(display, (0, 0, 255),
[(120,120), (40,160), (40,220),
(200, 220), (200,160)], width=0)
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
pygame.display.update()
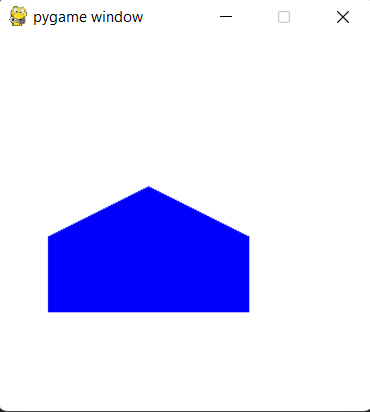
Polygon is filled because the width = 0. Try changing the value to see how it effects the output.
Drawing Circles in Pygame
pygame.draw.circle(surface, color, center, radius, width=0)
- surface: Window object to which we want to draw our Shape.
- color: Color of the drawn shape.
- center: Tuple/List which defines coordinate pair for center of origin of the Circle.
- radius: Defines the radius for the circle. (Distance from center to edge)
- width: Default value of 0, which means Circle will be filled (color). Values above 0 will make it unfilled, and width will control border thickness.
import pygame
import sys
pygame.init()
display = pygame.display.set_mode((300, 300))
display.fill((255, 255, 255))
pygame.draw.circle(display, (0, 0, 255),
center=(150,150), radius=50, width=0)
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
pygame.display.update()
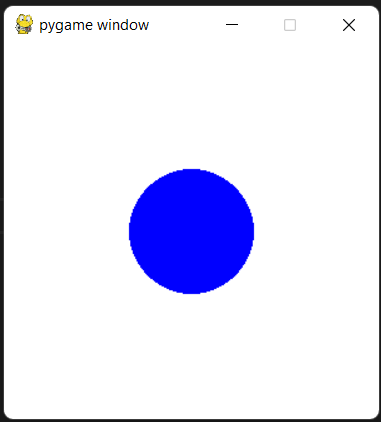
Drawing Ellipses in Pygame
Despite being very similar in its structure to a Circle, the parameters for an ellipse are slightly different.
pygame.draw.ellipse(surface, color, bounding_rectangle, width=0)
- surface: Window object to which we want to draw our Shape.
- color: Color of the drawn shape.
- bounding_rectangle: Takes a Rect object which defines the rectangle it will be confined in.
- width: Default value of 0, which means Ellipses will be filled (color). Values above 0 will make it unfilled, and width will control border thickness.
import pygame
import sys
pygame.init()
display = pygame.display.set_mode((300, 300))
display.fill((255, 255, 255))
pygame.draw.ellipse(display, (0, 0, 255),
pygame.Rect(40, 80, 200, 80), width=0)
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
pygame.display.update()
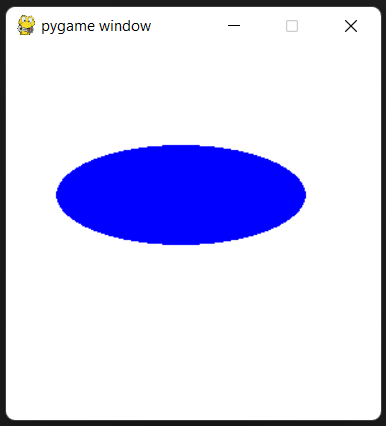
Drawing Rectangles in Pygame
pygame.draw.rect(surface, color, rectangle_tuple, width=0, border_radius=0)
- surface: Window object to which we want to draw our Shape.
- color: Color of the drawn shape.
- rectangle_tuple: (Read full description below)
- width: Default value of 0, which means Rectangle will be filled (color). Values above 0 will make it unfilled, and width will control border thickness.
- border_radius: Higher values can make the border edges “rounded”.
Rather than taking a set of co-ordinates like a Circle or Line function does, this function takes a parameter called rectangle_tuple
, which is a tuple containing 4 values. The purpose of each value is shown below in order. (The order is very important and must not be mixed up).
- The X co-ordinate of the upper left corner of the rectangle, also known as the X co-ordinate from where the Rectangle begins.
- The Y co-ordinate of the upper left corner of the rectangle, also known as the Y co-ordinate from where the Rectangle begins.
- The Width (Length) of the Rectangle in pixels.
- The Height of the Rectangle in pixels.
import pygame
import sys
pygame.init()
display = pygame.display.set_mode((300, 300))
display.fill((255, 255, 255))
pygame.draw.rect(display, (0, 0, 255),
(40, 80, 200, 80), width=0, border_radius=20)
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
pygame.display.update()
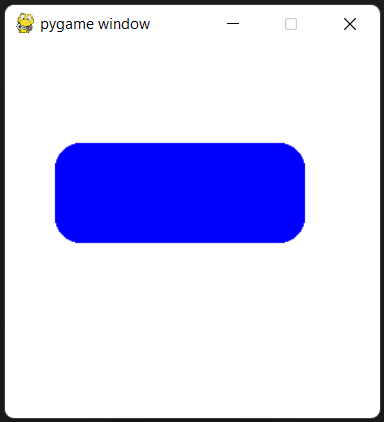
Rectangles in Pygame are used for more than just to draw shapes. They are used extensively in collision detection between entities (follow the link for a proper tutorial).
This marks the end of the How to Draw Shapes in Pygame Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the Tutorial content can be asked in the comments section below.