Pygame allows us to load, create and render images to the screen. Naturally, when dealing with images we often wonder “How do we rotate an image in Pygame” before drawing it to the screen. Luckily Pygame provides built in functions for this purpose, so we do not have to rely on other libraries.
Rotate Images in Pygame
The image we will be practicing on in this tutorial is shown below.
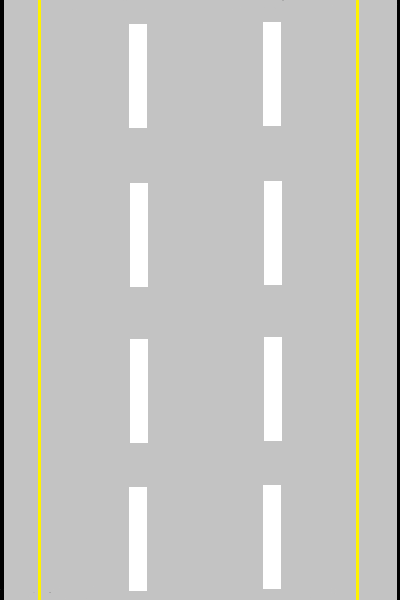
The image above shows us a segment of a road. Our first goal will be to create a road segment that goes from left-to-right by rotating the above image by 90 degrees.
To rotate surfaces in Pygame, we will be using the pygame.transform.rotate()
function.
Don’t be confused when we use the word “surfaces”. A surface is an object that can be drawn to the screen. This can be an image, a shape or even text. The image.load()
function actually returns a surface object for the image in your filepath.
Here is the syntax for the pygame.transform.rotate()
function.
new_surface = pygame.transform.rotate(surface, angle)
This function does not the rotate the surface/image directly, instead it returns a new surface object that has been rotated.
To rotate our street image by 90 degrees (counter-clockwise) we need to pass in image object in the first parameter and 90 in the second.
The code for this is as follows:
import pygame
import sys
pygame.init()
screen = pygame.display.set_mode((400, 300))
image = pygame.image.load("D:\pygame\Street.png")
image = pygame.transform.rotate(image, 90)
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
screen.blit(image, (180, 100))
pygame.display.update()
Remember that the image is being rotated before it is drawn to the screen. The origin point will also be the same, as we made no change to that in the screen.blit()
function.
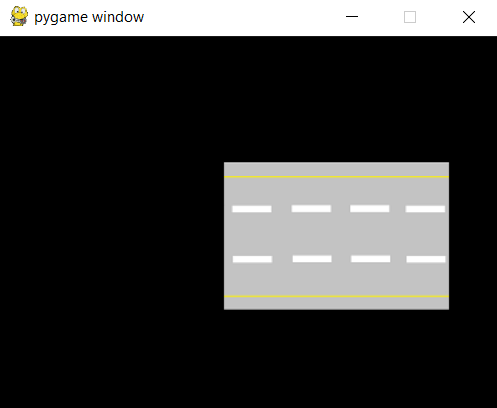
To clarify, the image rotated anti-clockwise.
Rotating Clockwise
We can also rotate images in the clockwise direction by passing negative values. This is not very obvious with the street image, so we will be using a different one this time.

Now let’s try rotating this with -90 and 90 as the angles.
image = pygame.transform.rotate(image, 90)
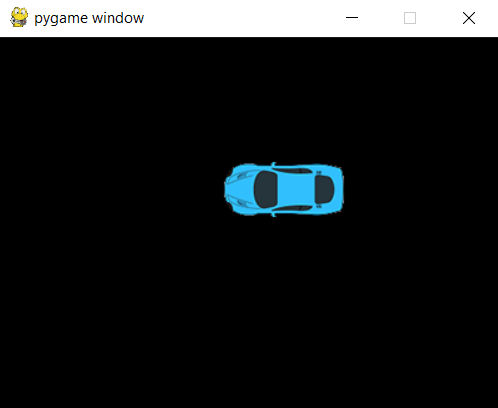
Now with negative values (for clockwise direction)
image = pygame.transform.rotate(image, -90)
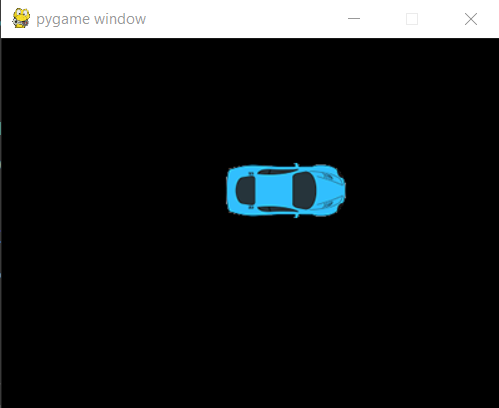
This marks the end of the How to Rotate an Image in Pygame Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.