This article covers the concept of surface creation in Pygame.
Surfaces play a big role in Pygame in the representation of the appearance objects on the display screen. Any text, image or object you create in Pygame will have been made with the help of a Surface.
The most common surface object used in Pygame is created using the pygame.display.set_mode()
. I’d wager that most you didn’t know this until now.
displaysurface = pygame.display.set_mode((400,600))
In the below article, you’ll learn more about Surfaces and their role in Pygame.
The Surface() Function
The pygame.Surface()
function is used to create the Surface object. It takes as parameter a tuple with two values. The first represents it’s width and the second defines it’s length.
The below code creates two surfaces, a square and a rectangle.
mySurface = pygame.Surface((50, 50))
mySurface2 = pygame.Surface((50, 100))
This is only the first step however, and doing this alone will not display a surface object to the screen.
The fill() Function
One of the many methods possessed by the surface object is the fill()
function. The fill()
function takes a color object as an input argument and “fills” in the surface object with that color.
mySurface1.fill( (0, 255, 0) ) # Green
mySurface2.fill( (255, 0, 0) ) # Red
mySurface3.fill( (0, 0, 255) ) # Blue
The fill()
is actually an optional step. If you were to remove this step, the surface object would be the default Black.
The blit() Function
The blit()
method possessed by a surface object is used to draw a surface object onto another.
surface.blit(source, destination)
This function typically takes two parameters. The first is the source surface which is to be drawn on the surface on which this method is being called. The second is the destination. This can either be pair of coordinates representing the upper left corner of the source, or a rect
object where the top-left corner of the rectangle will be used as the position for the blit
.
displaysurface.blit(mySurface, (50,50))
Let’s take a look at the code above. displaysurface
is the surface representing the entire screen. It’s dimensions decide the size of the pygame window. Now we want to draw our new Surface object, mySurface
to the pygame window.
Using the blit()
function on displaysurface
and passing mySurface
draws mySurface
onto displaysurface
.
Remember though, until the pygame.display.update()
function has been called, none of the changes by calling the calling the blit()
function will actually show on screen.
Pygame Surface Code
Below is the full code for a Pygame Surface creation demonstration. It combines the code and concepts from the functions described earlier and puts it together into one final program.
import pygame
from pygame.locals import *
import sys
pygame.init()
displaysurface = pygame.display.set_mode((300, 300))
mySurface = pygame.Surface((50, 50))
mySurface.fill((0,255,0))
mySurface2 = pygame.Surface((100, 50))
mySurface2.fill((0,255,0))
while True:
for event in pygame.event.get():
if event.type == QUIT:
pygame.quit()
sys.exit()
displaysurface.blit(mySurface, (50,50))
displaysurface.blit(mySurface2, (50,150))
pygame.display.update()
And below is the output.
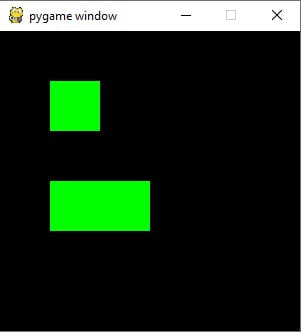
Of course, what you right now isn’t actually very practical and has little real life use. That’s because it’s just one of the many smaller concepts required in putting together an entire game. To learn more about game creation, check our Pygame Game Creation Tutorial.
There are dozens of different functions associated with Surface objects. However we won’t be covering them here, except for a few I thought would be most useful.
pygame.Surface.copy()
is a function used to create an exact copy of another Surface object with the same colors, transparency etc.
pygame.Surface.get_size()
returns the width and height of a Surface object.
You can individually return width and height of a Surface using pygame.Surface.get_width()
and pygame.Surface.get_height()
.
pygame.Surface.convert()
is used to convert a pygame.Surface
object to the same pixel format as the one you use for final display, the same one created from pygame.display.set_mode()
. If you don’t call it, then every time you blit a surface to your display surface, a pixel conversion will be needed. As this is a per pixel operation it’s very slow and will become noticeable in performance constrained systems.
There usually dozens of functions associated with such Classes, but many of them are highly situational, so chances are you might never end up using most of them. That’s what documentation is for though. Follow this link to get to the pygame surface documentation page.
This marks the end of the Pygame Surface creation article. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comment section below.
Great tutorial, will likely come back to this while I continue to learn and try to build my own stuff. Thank you!
You call these operations like .blit .fill functions when they are methods. An Class object has methods not functions. it is confusing and incorrect to call them functions as it implies that we are not working with objects, i think you should edit this article.