This is the “New Level” tutorial (20) in our Pygame RPG series
Any good RPG has more than a single dungeon/level for it’s players to battle in. The different dungeons are made interesting and unique with different environments, different enemies and other twists and turns.
In this Pygame RPG tutorial, as well as the next few, we’ll be working on creating on a new Level and populating it with new enemies and other new features.
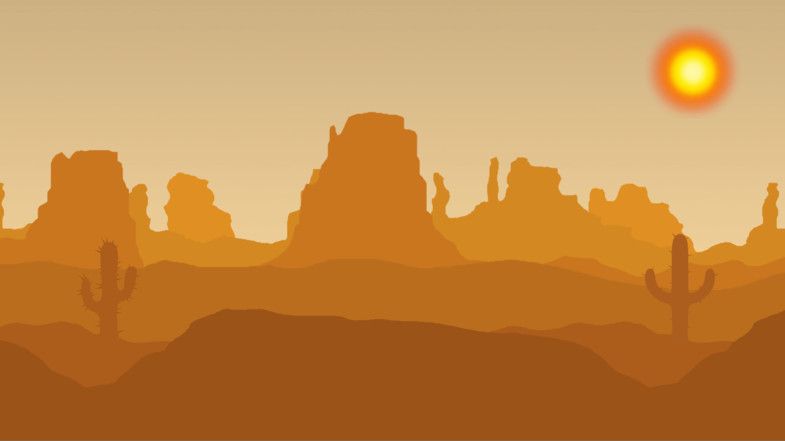
The new background for our new level. (pictured above)
EventHandler
Most of the changes that we make, will be to the EventHandler class, which handles most of the world building, enemy generation and other related fields.
First we have to add a new event, self.enemy_generation2
, which will generate the enemies in our new world.
class EventHandler():
def __init__(self):
...
self.enemy_generation = pygame.USEREVENT + 2
self.enemy_generation2 = pygame.USEREVENT + 3
...
self.world = 0
Next we’ll begin filling in our world2()
function. We’ll also load up the new background images that we’ve acquired for this world.
def world2(self):
self.root.destroy()
background.bgimage = pygame.image.load("desert.jpg")
ground.image = pygame.image.load("desert_ground.png")
pygame.time.set_timer(self.enemy_generation2, 2500)
self.world = 2
button.imgdisp = 1
castle.hide = True
self.battle = True
We’re also going to set the timer for the generation of enemies. We’ll give it a slightly higher time interval between enemies (2500), than the previous 2000, because the new enemies we create will be stronger.
Next we’ll edit the next_stage()
function. Previously we would just call the self.enemy_generation
event again (with a lower interval), but this time we’re going to have to pick between enemy_generation
and enemy_generation2
.
def next_stage(self): # Code for when the next stage is clicked
button.imgdisp = 1
self.stage += 1
self.enemy_count = 0
self.dead_enemy_count = 0
if self.world == 1:
pygame.time.set_timer(self.enemy_generation, 1500 - (50 * self.stage))
elif self.world == 2:
pygame.time.set_timer(self.enemy_generation2, 1500 - (50 * self.stage))
Of course, we’ll make this choice using self.world
to detect which level we are on, and which enemy will generated.
We haven’t actually added any enemies yet, as we are just setting up the framework. Similarly, we’re going to create the code blocks where the new enemy will be generated. We’re going to add a new detected statement in the game loop for the new event we made.
if event.type == handler.enemy_generation2:
if handler.enemy_count < handler.stage_enemies[handler.stage - 1]:
pass
We’ll leave the if statement blank for now, using a placeholder loop statement called pass
.
Home Function
Lastly, we need to make 2 additions in the Home function. We need to set the enemy_generation2
to 0, to effectively disable it (we don’t want enemies spawning in the home area).
def home(self):
# Reset Battle code
pygame.time.set_timer(self.enemy_generation, 0)
pygame.time.set_timer(self.enemy_generation2, 0)
self.battle = False
self.enemy_count = 0
self.dead_enemy_count = 0
self.stage = 0
self.world = 0
Secondly, we need to switch the world level, back to 0. Which means, we are at “home”. There’s also alot of other code, which resets the positions and counters back to normal. We explained all that and more in the Button/Home Tutorial (If you want a recap).
We are also going to reset the stage position back to 0. This means that any progress made in the dungeon/world was lost. Whether you want to reset it or not is upto you, and how you want to handle your game.
Next Section
Now we’re all set and ready for introducing our second enemy type into this game. Unlike our current enemies, we’ll be putting in more effort and newer features into these. For one, they have ranged attacks of their own, similar to the Player’s Fireballs.
This marks the end of the Pygame RPG New Level Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.