This is tutorial number 3 in the Pygame RPG series
The most important class in this RPG tutorial is the Player class. This class is responsible for almost everything player related, including movement, attacking, collision detection, rendering, state tracking and many more.
Due to it’s total size and many concepts, we’ll be building up the Player class slowly over the rest of the tutorial series. In this tutorial and the next, we’ll focus on creating the base code for the Player class.
Creating the Player Class
If you’ve been through our previous tutorial on Backgrounds, you’ll know what the self.image
and self.rect
variables are storing (image and rect object respectively).
class Player(pygame.sprite.Sprite):
def __init__(self):
super().__init__()
self.image = pygame.image.load("Player_Sprite_R.png")
self.rect = self.image.get_rect()
# Position and direction
self.vx = 0
self.pos = vec((340, 240))
self.vel = vec(0,0)
self.acc = vec(0,0)
self.direction = "RIGHT"
Now for the variables for position and direction. self.direction
is used to store the current direction of the Player. By default we have kept the player facing right.
The other three variables, self.pos
, self.vel
and self.acc
are created using the vector object we created in the first tutorial. What this means is that these variables now have two components which can be accessed in the following format, self.pos.x
and self.pos.y
. It’s a useful way of keeping both components in a single variable, instead of two separate ones.
As to purpose of these three variables, self.pos
is for the position of the player. self.vel
is for the velocity of the player and self.acc
is for the acceleration of the player. (If you’ve studied Physics, you should know that velocity and acceleration have vertical and horizontal components)
Creating the remaining functions
We’ll be covering the remaining functions later on in this series, in the appropriate tutorials. For now just remember that they exist and what their purpose is going to be (move for movement code, attack for attacking code).
def move(self):
pass
def update(self):
pass
def attack(self):
pass
def jump(self):
pass
Once again, we use the pass
keyword as a place holder. Leaving the function blank will cause an error after all. Also keep the indentation of the methods in mind. Incorrect indentation will result in the methods not being part of the Player class.
Creating the player object
Once we’re done with class, we have to actually create the player object through which all interaction will take place. The fact that you can create multiple objects from the same class also opens up the possibility of creating a two player game.
player = Player()
Remember to declare this before the game loop, and not within it.
Finally, we need to render our player. We’ll be adding the following line of code, “after” the rendering of both the ground and background. All these render functions are of course, in the game loop since they need to be redrawn every frame.
background.render()
ground.render()
displaysurface.blit(player.image, player.rect)
We don’t “have” to create a render function. We’ve showcased this by directly writing the blit()
method for the player. Also this time we just passed player.rect
into blit()
, which works because rect
stores a pair coordinates.
Below is a screen shot of our Player sprite in the pygame window.
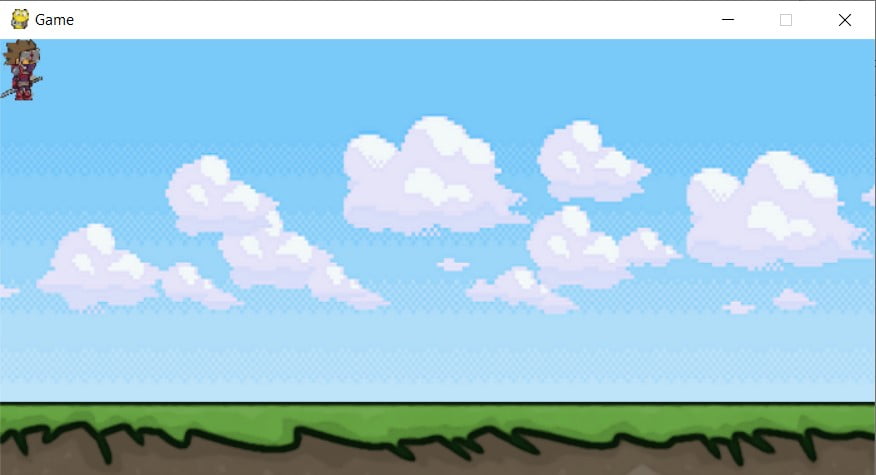
The reason why our player is at the top left corner is because we didn’t pass a center = (x,y)
. Because of this, the player rect defaults to a value of (0 , 0). This problem will be solved later on in the series, so don’t worry.
Next Section
This tutorial was just the intro to the Player class. We’ll be slowly building both the game and the Player class over the next 4 – 5 tutorials, so pay close attention.
The button below is a download link for the Player sprite image.
This marks the end of the Player Class tutorial of our Pygame RPG tutorial series. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.
can u tell me where to place the images specifically please am using pycharm
You just need to put them in the same directory as your .py file. That’s all. Otherwise you could specify full paths in the python code to where ever you have the images stored.
I’m getting this error, any solution?
Traceback (most recent call last):
File “c:\Python\Pygame\Coderslegacy\RPG\main.py”, line 85, in <module>
player = Player()
File “c:\Python\Pygame\Coderslegacy\RPG\main.py”, line 57, in __init__
self.pos = vec((340, 240))
TypeError: ‘pygame.math.Vector2’ object is not callable
Can you try removing the parenthesis for the vec() assignment to self.pos.
Like this: self.pos = vec(340, 180)
It works for me either way, but may change for you.