This article covers the PyQt QCheckBox widget (PyQt5).
A Checkbox is an important part of any GUI, used when you want to present the User with a list of options. In PyQt, we have a widget called the QCheckBox which we use to create check boxes or “check buttons” as they are sometimes called.
You will find a complete list of methods and options available for QCheckBox at the end of this pyqt5 tutorial.
Creating a QCheckBox widget
Below is the simplest example of a QCheckbox widget. Calling the QtWidgets.QCheckBox()
function and passing the window (win
) that we created into it’s parameters, creates a PyQt Checkbox.
To actually display and position it we’ve used the move()
function to move it to a certain set of coordinates. For more advanced layout methods, look up our Pyqt5 layout management tutorial.
from PyQt5 import QtWidgets
from PyQt5.QtWidgets import QApplication, QMainWindow
import sys
app = QApplication(sys.argv)
win = QMainWindow()
win.setGeometry(400,400,300,300)
win.setWindowTitle("CodersLegacy")
check = QtWidgets.QCheckBox(win)
check.setText("Option1")
check.move(50,100)
win.show()
sys.exit(app.exec_())
The GUI output of the above code:
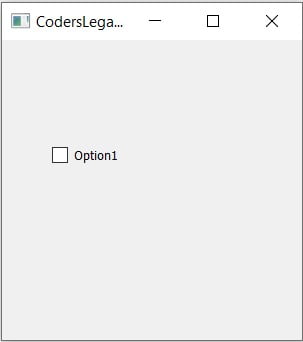
Retrieving QCheckBox values
You can retrieve QCheckBox values using the text()
method, which will return the text value you assigned to the Checkbox.
There is another method you can use called checkState()
. This returns an integer number that represents whether the checkbox is selected or not.
We’re using a rather advanced method of calling the show()
function here. Instead of creating a submit button, we’ve used the stateChanged.connect()
method on the CheckBox. This causes the show()
function to be called whenever the state of the checkbox has been changed.
from PyQt5 import QtWidgets
from PyQt5.QtWidgets import QApplication, QMainWindow
import sys
def show():
print(check.text())
print(check.checkState())
app = QApplication(sys.argv)
win = QMainWindow()
win.setGeometry(400,400,300,300)
win.setWindowTitle("CodersLegacy")
check = QtWidgets.QCheckBox(win)
check.setText("Option1")
check.stateChanged.connect(show)
check.move(50,100)
win.show()
sys.exit(app.exec_())
Below is the output of the above code. It has been clicked twice, once to select and once to de-select it. You can see the output to it’s side.
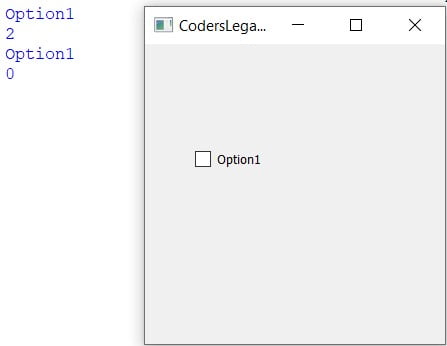
0
represents the “off” state and 2
represents the “on” state. These are fixed values. 1
represents a state where the Checkbox has not been interacted with at all.
You can use an alternative method involving a button, that when clicked will return the appropriate values. You can find examples of this in other articles of our PyQt section.
CheckButtons Groups
Most of the time you will want to be using multiple check-buttons. To do this we need the help of a grouping widget like QGridLayout. This creates a connection between the check buttons that we can use later on.
Through addWidget()
is used to add a widget to a group. The first parameter represents the widget, and the last represents it’s unique value.
from PyQt5 import QtWidgets
from PyQt5.QtWidgets import QApplication, QMainWindow, QGridLayout
import sys
app = QApplication(sys.argv)
win = QMainWindow()
win.setGeometry(400,400,300,300)
win.setWindowTitle("CodersLegacy")
layout = QGridLayout()
check = QtWidgets.QCheckBox(win)
check.setText("Option1")
check.move(50,100)
layout.addWidget(check, 0, 0)
check2 = QtWidgets.QCheckBox(win)
check2.setText("Option2")
check2.move(50,150)
layout.addWidget(check2, 0, 1)
win.show()
sys.exit(app.exec_())
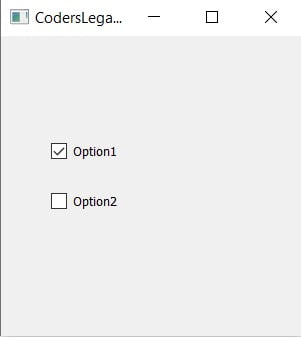
Don’t forget to add that extra import for the Grid Layout Manager.
Methods Compilation
Below is a compilation of all the methods available for the QCheckButton widget.
Method | Description |
---|---|
checkState() | Checks the current state of the Checkbox. Returns values ranging from 0 – 2 depending on the state. |
setChecked() | Changes the state of the Checkbox to checked. |
setText() | Sets the text associated with the checkbox. |
text() | Retrieves the text value of the CheckBox. |
isChecked() | Checks to see if the checkbox is selected. |
setTriState() | Provides a third kind of state called “no change” state. |
This marks the end of the PyQt QCheckbox Article. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the PyQt5 can be asked in the comments section below.