This article covers the QDial widget in PyQt
While not very common, one way of taking input from the user in a interactive and visually pleasing way is through the use of Dials. Most uses of Dials tend to be for things like Volume and Audio control, where turning the dial lowers or increases the volume.
PyQt5 with it’s QDial widget, gives us the functionality we need to create Dials in our GUI program.
Creating a QDial
After creating the Dial with the QDial()
function, we can customize it with the help of various methods belonging to the QDial widget class. A full explanation and listing for these methods (and others) is included at the end of the article.
from PyQt5 import QtWidgets
from PyQt5.QtWidgets import QApplication, QMainWindow
import sys
app = QApplication(sys.argv)
win = QMainWindow()
win.setGeometry(400,400,300,300)
win.setWindowTitle("CodersLegacy")
dial = QtWidgets.QDial(win)
dial.setMinimum(0)
dial.setMaximum(100)
dial.move(100,50)
win.show()
sys.exit(app.exec_())
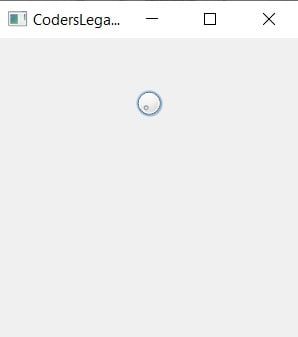
Here’s a slightly zoomed in version of the QDial. That little circle within the Dial is what you move to turn the Dial.
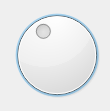
Returning Dial Values
Now that we’ve created a Dial, it’s time to learn how to actually begin returning the values as the Dial is moved.
The only two new things in this code is the display()
function we created, and the use of the valueChanged()
method on the dial. This method causes the display()
function to be called whenever the dial is moved or the “value is changed”.
from PyQt5 import QtWidgets
from PyQt5.QtWidgets import QApplication, QMainWindow
import sys
def display():
print(dial.value())
app = QApplication(sys.argv)
win = QMainWindow()
win.setGeometry(400,400,300,300)
win.setWindowTitle("CodersLegacy")
dial = QtWidgets.QDial(win)
dial.setMinimum(0)
dial.setMaximum(100)
dial.move(100,50)
dial.valueChanged.connect(display)
win.show()
sys.exit(app.exec_())
Below is a screen-shot of what happened when we moved the dial a bit. As you can see, a continuous stream of values was printed out till we stopped moving the dial.
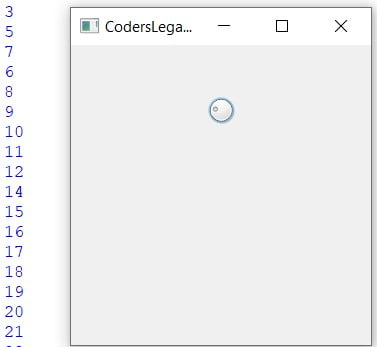
QDial Methods
A list of the different method available for the PyQt QDial widget.
Method | Description |
---|---|
setValue() | Sets the current value of the Dial |
value() | Returns the current value of the Dial |
setMinimum() | Sets the minimum possible value for the Dial |
setMaximum() | Sets the Maximum possible value for the Dial |
setNotchesVisible(boolean) | Depending on whether it’s True or False, the Notches are displayed on the Dial. False by default. |
QDial Signals
The QDial has more signals generating methods than just valueChanged()
. You may find some of these useful, so here’s a short list of them.
Method | Description |
---|---|
valueChanged() | Generates a signal whenever the value is changed. |
sliderMoved() | Generated whenever the Dial is moved |
sliderPressed() | Generates a signal when the Dial is Pressed |
sliderReleased() | Generates a signal when the Dial is released. |
You can use the connect()
method to connect these signals to functions as well.
This marks the end of the PyQt QDial article. Any suggestions or contributions for CodersLegacy are more than welcome. Questions can be asked in the comments section below.
Head over to the main PyQt5 article to learn about other great widgets!