This article covers the PyQt QProgressBar Widget (PyQt5)
Progress bars is a great way to visualize progression of computer operations such as file transferring, downloading, uploading, copying etc. The alternative would be text-based which is rather boring and lacking in comparison.
PyQt has support for creating progress bars with it’s QProgressBar widget class.
At the end of this tutorial, you’ll find a complete list of methods and options available for the ProgressBar widget.
PyQt ProgressBar Example
Using the QtWidgets.QProgressBar()
function we created a progress widget which we stored in prog_bar
.
Using the setGeometry()
method we decide both the location and the dimensions of the progressbar. The first two parameters represent the X and Y position of the progressbar on the window. The third and fourth parameters are the width and height of the progressbar.
from PyQt5 import QtWidgets
from PyQt5.QtWidgets import QApplication, QMainWindow
import sys
app = QApplication(sys.argv)
win = QMainWindow()
win.setGeometry(400,400,300,300)
win.setWindowTitle("CodersLegacy")
prog_bar = QtWidgets.QProgressBar(win)
prog_bar.setGeometry(50, 50, 250, 30)
prog_bar.setValue(0)
win.show()
sys.exit(app.exec_())
The GUI output of the above example:
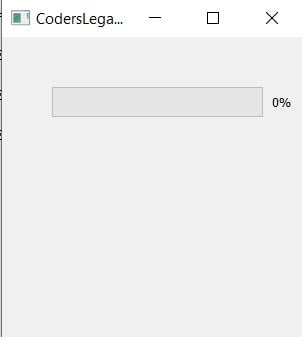
Updating ProgressBar Values
Using the setValue()
method we set values for the QProgressBar in PyQt. Keep in mind however, the range for a progressbar is from 0 to 100. If you want to change this, use the setRange()
(discussed at the bottom of page).
Using the value()
method we can return the current value of the progressbar. We’ve used the values returned by this method to keep incrementing the progress bar.
from PyQt5 import QtWidgets
from PyQt5.QtWidgets import QApplication, QMainWindow
import sys
def update():
value = prog_bar.value()
prog_bar.setValue(value + 1)
def reset():
value = 0
prog_bar.setValue(value)
app = QApplication(sys.argv)
win = QMainWindow()
win.setGeometry(400,400,300,300)
win.setWindowTitle("CodersLegacy")
prog_bar = QtWidgets.QProgressBar(win)
prog_bar.setGeometry(50, 50, 250, 30)
prog_bar.setValue(0)
button = QtWidgets.QPushButton(win)
button.setText("Update")
button.clicked.connect(update)
button.move(50,100)
reset_button = QtWidgets.QPushButton(win)
reset_button.setText("Reset")
reset_button.clicked.connect(reset)
reset_button.move(50, 150)
win.show()
sys.exit(app.exec_())
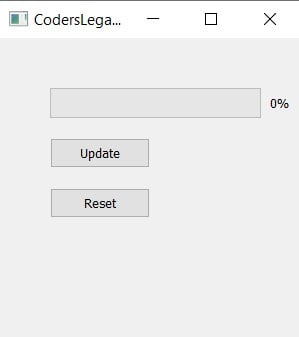
A classes approach would have better here, but for explanatory purposes, we chose a simpler approach.
ProgressBar Methods
A compilation of the different methods available for ProgressBars in PyQt.
Method | Description |
---|---|
setValue() | Sets the value of the progressbar. |
reset() | Resets the value on the progressbar. |
value() | Returns the current integer value. |
setRange(n1,n2) | Takes two numbers as parameters to define a start and end point. Default values are 0 and 100 respectively. |
This marks the end of the PyQt QProgressBar article. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the PyQt5 article can be asked in the comments section below.
Head back to the main tutorial page to learn about other great widgets!