This article covers the PyQt QDateEdit widget (PyQt5).
The PyQt5 QDateEdit widget is a interactive and visually pleasing way for the user to enter in a Date as input. It’s a simple widget where you can edit the date manually or through the use of the in-built arrow keys.
You will find a full list of methods for the QDateEdit widget at the end of this article.
Creating a QDateEdit widget
Using the QDateEdit()
function from the QtWidgets class will create the widget. Be sure to pass the window
(win) object you created into the parameters of the function, else it won’t display.
You don’t have to assign it a minimum or maximum, PyQt will assign default values if you don’t. But it’s nice to have the ability to do so.
from PyQt5 import QtWidgets
from PyQt5.QtWidgets import QApplication, QMainWindow
import sys
app = QApplication(sys.argv)
win = QMainWindow()
win.setGeometry(400,400,250,250)
win.setWindowTitle("CodersLegacy")
date = QtWidgets.QDateEdit(win)
date.setMinimumDate(QDate(1900, 1, 1))
date.setMaximumDate(QDate(2100, 12, 31))
date.move(50,50)
win.show()
sys.exit(app.exec_())
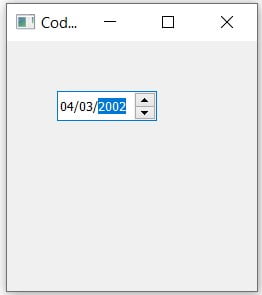
Retrieving QDateEdit values
Once again, we’ll be using the currentDate()
and the button widget together to successfully get the Date value.
You’ll notice the use of special methods and classes such as QDate
and toPyDate
. This is because the QDateEdit widget’s value are all in a special Date format.
When passing a date value, you must use QDate to convert it into an appropriate format and when returning the date value, use toPyDate
to print it in a more readable format.
from PyQt5 import QtWidgets
from PyQt5.QtWidgets import QApplication, QMainWindow
from PyQt5.QtCore import QDate
import sys
def display():
print(date.date().toPyDate())
app = QApplication(sys.argv)
win = QMainWindow()
win.setGeometry(400,400,250,250)
win.setWindowTitle("CodersLegacy")
date = QtWidgets.QDateEdit(win)
date.setMinimumDate(QDate(1900, 1, 1))
date.setMaximumDate(QDate(2100, 12, 31))
date.move(50,50)
button = QtWidgets.QPushButton(win)
button.setText("Press me")
button.clicked.connect(display)
button.move(50,100)
win.show()
sys.exit(app.exec_())
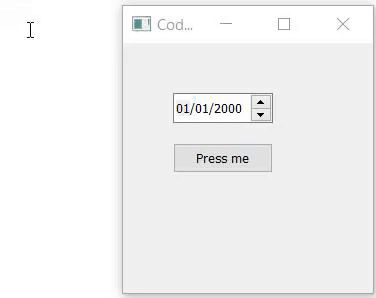
You don’t have to use toPyDate()
. Sometimes you may require the actual returned Date object. In that case, simple remove the toPydate()
method from the above code.
QDateEdit Methods
Below is a compilation of a few useful methods for the QDateEdit widget.
Method | Description |
---|---|
date() | Returns the current date displayed on the widget, |
setDate() | Used to set the currently displayed date. |
setMinimumDate() | Sets the minimum possible date the User can select. |
setMaximumDate() | Sets the maximum possible date the User can select. |
Head back over to the main pyqt tutorial to learn about the other great widgets.
This marks the end of the PyQt5 QDateEdit article. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.