This article covers the PyQt5 QPushButton widget with examples.
One of the most basic and common widgets in PyQt5, is QPushButton. As the name implies, it’s a button that triggers a function when pushed (clicked).
You will find a list of all the methods for the PyQt QPushButton available at the bottom of this page.
Creating a QPushButton Widget
Using the QtWidgets.QPushButton()
function, we’re able to create a button widget. We can then customize this button with methods like setText()
and move()
which determine the text and location of the widget respectively.
from PyQt5 import QtWidgets
from PyQt5.QtWidgets import QApplication, QMainWindow
import sys
WIDTH = 500
HEIGHT = 300
app = QApplication(sys.argv)
win = QMainWindow()
win.setGeometry(400,400,WIDTH,HEIGHT)
win.setWindowTitle("CodersLegacy")
button = QtWidgets.QPushButton(win)
button.setText("A Button")
button.move(WIDTH/2,HEIGHT/2)
win.show()
sys.exit(app.exec_())
Below is the output of the code we just wrote. We haven’t mapped this button to any function yet, so clicking it won’t do anything.
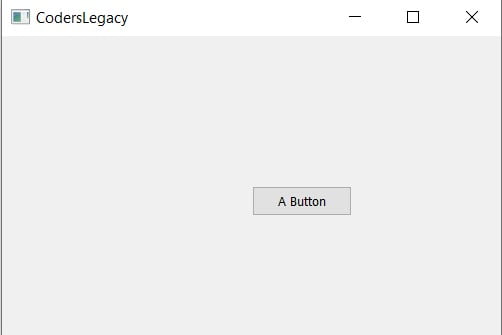
We added a little extra code by creating two variables WIDTH and HEIGHT in the attempt to get the widget to appear in the center of the screen. However, the move()
function does not center the QPushButton at the coordinates passed to it, rather it starts drawing the widget from there. In other words, the top left hand corner of the button we created above is located at the center of the screen.
move()
is a very basic layout system, and not suitable for actual projects. PyQt5 comes with actual layouts, in charge of managing positions of the widgets inside the window. Learn more about them in our PyQt5 layout management tutorial.
Connecting Buttons to Functions
A button which isn’t connected to a function is a useless button. Buttons are meant to be connected to a function which will execute once the button has been pressed.
We’ll explore several popular usages of the QPushButton widget in PyQt5, such as closing the window, updating text and retrieving widget values.
Buttons with Labels
You can learn more about PyQt Labels in it’s own dedicated tutorial.
from PyQt5 import QtWidgets
from PyQt5.QtWidgets import QApplication, QMainWindow
import sys
def update():
label.setText("Updated")
def retrieve():
print(label.text())
app = QApplication(sys.argv)
win = QMainWindow()
win.setGeometry(400,400,500,300)
win.setWindowTitle("CodersLegacy")
label = QtWidgets.QLabel(win)
label.setText("GUI application with PyQt5")
label.adjustSize()
label.move(100,100)
button = QtWidgets.QPushButton(win)
button.clicked.connect(update)
button.setText("Update Button")
button.move(100,150)
button2 = QtWidgets.QPushButton(win)
button2.clicked.connect(retrieve)
button2.setText("Retrieve Button")
button2.move(100,200)
win.show()
sys.exit(app.exec_())
Here’s a short video to show you how the output of the above code would look like.
Shutting down a PyQt application
Another popular usage of QPushButton is to create a “Quit Button” that closes the PyQt5 application when clicked. Here’s how to do it.
We simply use the close()
method on the window that we created using the QMainWindow()
function.
Instead of creating a whole separate function and then calling win.close()
, we can take a shortcut and simply pass win.close
to the connect function. Remember to leave off the parenthesis, else the function will call itself automatically.
We’ve also thrown in an extra method, called resize()
which is used to change the size of the QPushButton. The first parameter defines the new width, and the second defines the new height.
from PyQt5 import QtWidgets
from PyQt5.QtWidgets import QApplication, QMainWindow
import sys
app = QApplication(sys.argv)
win = QMainWindow()
win.setGeometry(400,400,500,300)
win.setWindowTitle("CodersLegacy")
button = QtWidgets.QPushButton(win)
button.clicked.connect(win.close)
button.setText("Quit Button")
button.resize(120,60)
button.move(350,220)
win.show()
sys.exit(app.exec_())
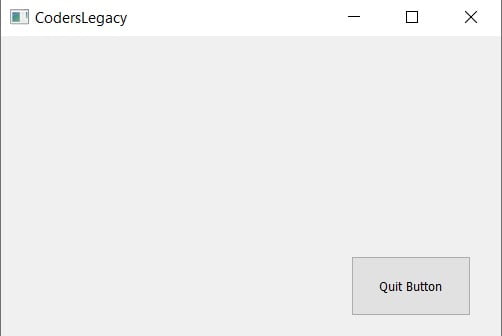
Setting an Image
You can set an image onto your button to make it look more stylish using the QIcon widget. Just pass the filepath into it and you’re done.
from PyQt5 import QtGui
button = QPushButton(win)
button.setIcon(QtGui.QIcon('myImage.jpg'))
QPushButton Methods
This is a compilation of all important and common methods for the QPushButton widget.
Method | Description |
---|---|
isDefault() | A button with this property set to true (i.e., the dialog’s default button,) will automatically be pressed when the user presses enter |
setIcon() | Displays an image icon on the button. |
setEnabled() | True by Default. If set to False, but will become grey and unresponsive. |
setText() | Sets the displayed text on the button. |
text() | Returns the displayed text on the button. |
toggle() | Toggles the state of the button. |
This marks the end of the PyQt5 QPushButton widget article. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.
Head over to the main PyQt5 section to learn more about other great widgets!
In QT pushbutton tutorial you introduce putting a graphic on a button with QtGui.QIcon(‘path to image file’)
But don’t mention what import you need in order to use that function.
Fixed!