This article covers the PyQt5, QLabel widget.
The QLabel is one of the simplest widgets in PyQt with the ability to display lines of text. This widget comes with many supporting functions and methods to allow us to retrieve and update this text whenever we want.
A complete list of all methods associated with QLabel can be found at the bottom of this page.
Creating a QLabel
The below example will demonstrate the creation of a simple QLabel in PyQt. If you’re having any trouble understanding the non – QLabel related code, see our Getting started with PyQt5 guide.
from PyQt5 import QtWidgets
from PyQt5.QtWidgets import QApplication, QMainWindow
import sys
app = QApplication(sys.argv)
win = QMainWindow()
win.setGeometry(400,400,500,300)
win.setWindowTitle("CodersLegacy")
label = QtWidgets.QLabel(win)
label.setText("GUI application with PyQt5")
label.adjustSize()
label.move(100,100)
win.show()
sys.exit(app.exec_())
The output of the above code is shown below.
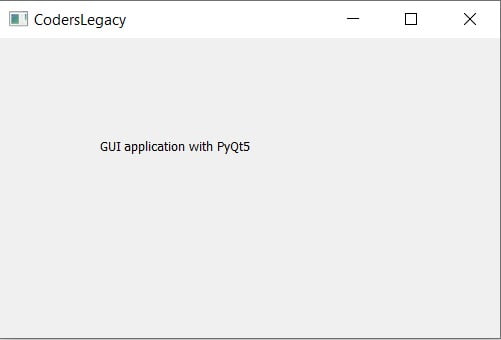
We’ll proceed to examine the QLabel related code line by line.
label = QtWidgets.QLabel(win)
Calling the QtWidgets.QLabel()
creates a label object which we can use for our GUI. Passing the window object we created (win
) into it’s parameters declares that the label is part of that window.
label.setText("GUI application with PyQt5")
A simple line of code that sets the Text for the label that gets displayed on the window.
label.adjustSize()
QLabels have a default size that is declared when they are created. For long lines of text, such as the one we passed into setText()
, the default size is too small. Luckily, we just have the call a single method adjustSize()
will cause the QLabel to automatically adjust it’s size. Not doing this will result in the text only appearing partially on the screen.
label.move(100,100)
This line determines the starting position of the QLabel when it appears on the screen. Passing (100, 100)
will result in the top left hand corner of the label appearing at coordinates (100 , 100). Keep in mind that the top left hand corner of the screen has the position (0, 0)
.
move()
is a very basic layout system, and not suitable for actual projects. PyQt5 comes with actual layouts, in charge of managing positions of the widgets inside the window. Learn more about them in our PyQt5 layout management tutorial.
Retrieving and Updating QLabel values
In this section we’ll explore how to update the text currently displayed on the QLabel, as well as how to retrieve the current text being displayed on the QLabel. To do such things we require the use of the QPushButton widget which will call the functions which contain the required commands.
To update the QLabel, we’ll be using the same setText()
method from before, and for retrieving the text value, we’re going to use the text()
method.
from PyQt5 import QtWidgets
from PyQt5.QtWidgets import QApplication, QMainWindow
import sys
def update():
label.setText("Updated")
def retrieve():
print(label.text())
app = QApplication(sys.argv)
win = QMainWindow()
win.setGeometry(400,400,500,300)
win.setWindowTitle("CodersLegacy")
label = QtWidgets.QLabel(win)
label.setText("GUI application with PyQt5")
label.adjustSize()
label.move(100,100)
button = QtWidgets.QPushButton(win)
button.clicked.connect(update)
button.setText("Update Button")
button.move(100,150)
button2 = QtWidgets.QPushButton(win)
button2.clicked.connect(retrieve)
button2.setText("Retrieve Button")
button2.move(100,200)
win.show()
sys.exit(app.exec_())
If you want to know more about how the above code works, head over to the QPushButton page where it’s explained in detail.
Here’s a short 5 second clip displaying the output of the code above.
QLabel Methods
A compilation of the most important methods available for the Label widget.
Method | Description |
---|---|
setAlignment() | Decides the Alignment of the text. Takes 4 possible values, Qt.AlignLeft, Qt.AlignRight, Qt.AlignCenter and Qt.AlignJustify. |
setIndent() | Defines the indentation for the text. |
setPixmap() | Used to display an image. |
text() | Returns the (displayed) text value for the label. |
setText() | Sets the text that is displayed on the widget. |
selectedText() | Returns the text that has been selected by the user. (For this to work, textInteractionFlag must be set to TextSelectableByMouse). |
setWordWrap() | Used to enable or disable text wrapping. |
adjustSize() | Automatically adjusts the Label size to the text being displayed. |
Head back to the main PyQt5 section to learn about other great widgets!
This marks the end of the PyQt5 QLabel widget article. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.
this tutorial is amazing
Very useful!