This tutorial covers the QTimer widget in PyQt5.
PyQt5 brings in support for Timers with it’s QTimer widget. A timer is a kind of feature waits for a certain duration and then sends out a signal to activate something, usually a function. The QTimer widget offers a variety of different timers such as repetitive and single shot ones. More on this later.
You will find a complete list of methods and functions for the PyQt5 QTimer widget at the end of this article.
Creating a QTimer
The QTimer widget is part of the QtCore library in PyQt5. Using this class we create our QTimer object called timer
.
timeout()
is a signal sent by the QTimer widget once the time is up. We use the connect()
method to link the timeout signal to the display
function.
Finally we begin the timer using the start method and passing 1000 milliseconds as the time for it to activate. By default a timer is repetitive and after it activates, it will keep repeating itself every 1000 milliseconds or 1 second.
from PyQt5 import QtWidgets
from PyQt5.QtWidgets import QApplication, QMainWindow
from PyQt5 import QtCore
import sys
def display():
print("Hello World")
app = QApplication(sys.argv)
win = QMainWindow()
win.setGeometry(400,400,300,300)
win.setWindowTitle("CodersLegacy")
timer = QtCore.QTimer()
timer.timeout.connect(display)
timer.start(1000)
win.show()
sys.exit(app.exec_())
Creating an End Point
The problem with out current version of the QTimer implementation is that it has no end point. The Timer we created will continue to call the display function every 2 seconds forever.
Sometimes you may not want this to happen. Maybe you just want it to happen once, or twice or just 10 times. In this section we’ll explain how to achieve this.
from PyQt5 import QtWidgets
from PyQt5.QtWidgets import QApplication, QMainWindow
from PyQt5 import QtCore
import sys
def display():
global count
count += 1
if count > 5:
print("Finished")
timer.stop()
return
print("Hello World")
count = 0
app = QApplication(sys.argv)
win = QMainWindow()
win.setGeometry(400,400,300,300)
win.setWindowTitle("CodersLegacy")
timer = QtCore.QTimer()
timer.timeout.connect(display)
timer.start(1000)
win.show()
sys.exit(app.exec_())
The output of the above code next to the console output.
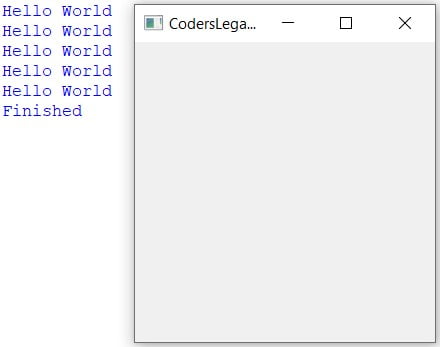
There is also an alternative (and simpler) option if you only want the Timer to run once using the setSingleShot() function. See the details below in the functions list.
If you use a Class based approach, you won’t have to worry about the global variable keyword.
QTimer Functions
A compilation of useful and important functions/methods for the QTimer widget.
Method | Description |
---|---|
interval() | Returns the time interval set for the Timer in milliseconds. |
isActive() | Returns True of False depending on whether the timer is active or not. |
remainingTime() | Returns (in milliseconds) the time remaining for the next timer call. |
start(time) | Used to start the timer. Takes as an argument the interval for the timer. |
stop() | Used to end/stop the timer. |
setSingleShot(bool) | Setting Single Shot to True will cause the timer to run only once. |
isSingleShot() | Returns True or False depending on whether Single Shot is on or not. |
This marks the end of the PyQt5 QTimer article. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.
Head back to the main PyQt5 tutorial to learn about the other great widgets!