This tutorial introduces you to the PyQt6 QProgressBar Widget
Progress bars are a great way to visualize in a user-friendly way, the progression or “completion status” for long tasks, such as file transferring, downloading, uploading, copying etc. The alternative to this would be a text-based GUI which is rather lacking in comparison.
PyQt6 offers us support for creating progress bars with it’s QProgressBar widget class. As with all PyQt6 widgets, there are a variety of ways in which we can customize these Progress Bars.
At the end of this tutorial, you’ll find a complete list of methods and options available for the ProgressBar widget.
PyQt ProgressBar Example
Using the QProgressBar()
Class from the QtWidgets Module, we will create a progress bar widget.
The setGeometry()
method, can be used to define the starting position and the size of the progressbar. The first two parameters for setGeometry()
represent the X and Y position of the progress bar’s top-left corner, on the PyQt6 window. The third and fourth parameters are the width and height of the progressbar.
from PyQt6.QtWidgets import QApplication, QWidget, QProgressBar
import sys
class Window(QWidget):
def __init__(self):
super().__init__()
self.resize(320,250)
self.setWindowTitle("CodersLegacy")
prog_bar = QProgressBar(self)
prog_bar.setGeometry(50, 100, 250, 30)
prog_bar.setValue(0)
app = QApplication(sys.argv)
window = Window()
window.show()
sys.exit(app.exec())
The GUI output of the above example:
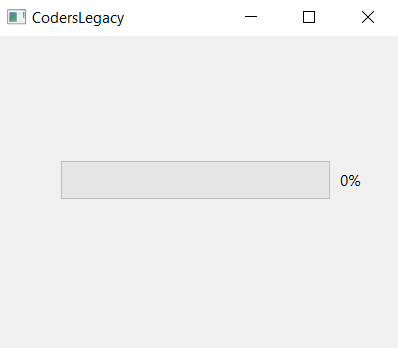
Updating ProgressBar Values
Using the setValue()
method we set values for the QProgressBar in PyQt. Keep in mind however, the range for a progressbar is from 0 to 100. If you want to change this, use the setRange()
(discussed in more detail at the end of tutorial).
Using the value()
method we can return the current value of the progressbar. We’ve used the values returned by this method to keep incrementing the progress bar.
from PyQt6.QtWidgets import QApplication, QWidget, QProgressBar, QPushButton
import sys
class Window(QWidget):
def __init__(self):
super().__init__()
self.resize(320,250)
self.setWindowTitle("CodersLegacy")
self.prog_bar = QProgressBar(self)
self.prog_bar.setGeometry(50, 50, 250, 30)
button = QPushButton("Update", self)
button.clicked.connect(self.update)
button.move(50,100)
reset_button = QPushButton("Reset", self)
reset_button.clicked.connect(self.reset)
reset_button.move(50, 150)
def update(self):
value = self.prog_bar.value()
self.prog_bar.setValue(value + 1)
def reset(self):
value = 0
self.prog_bar.setValue(value)
app = QApplication(sys.argv)
window = Window()
window.show()
sys.exit(app.exec())
The image below shows the what the GUI window and QProgressBar widget look like after the update button has been clicked 10 times.
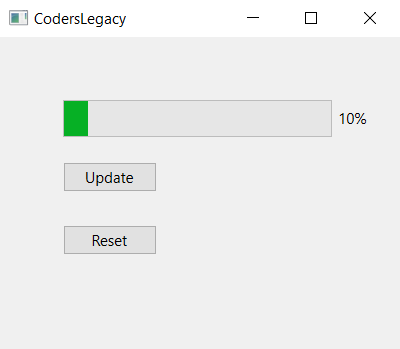
ProgressBar Methods
A compilation of the different methods available for ProgressBars in PyQt.
Method | Description |
---|---|
setValue(n) | Used to the value of the progressbar to “n”. |
reset() | Resets the value on the progressbar. |
value() | Returns the current integer value of the Sliders. |
setRange(n1,n2) | Takes two numbers as parameters to define a start and end point. Default values are 0 and 100 respectively. |
This marks the end of the PyQt6 QProgressBar article. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the PyQt5 article can be asked in the comments section below.