What is Python Tkinter Entry?
Python Tkinter Entry widget is used to take input from the user through the user interface. A simple box is provided on the interface where the user can input text. This text can then be retrieved and used by us through the use special functions.
Entry Syntax
from tkinter import *
Entry = Entry(master, option.........)
No. | Option | Description |
---|---|---|
1 | bg | The Background color for the label of this widget. |
2 | bd | Size of border around the Entry box. Default is 2. |
3 | command | If the state of this widget is changed, this procedure is called. |
4 | cursor | When the mouse is hovering over this widget, it can be changed to a special cursor type like an arrow or dot. |
5 | font | The type of font to be used for this widget. |
6 | exportselection | If text within the entry widget is selected, it will copied into the clipboard. Set exportselection = 0 to disable this. |
7 | fg | The color for the text. |
8 | highlightcolor | The text color when the widget is under focus. |
9 | Justify | Specifies how the text is aligned within the widget. One of “left”, “center”, or “right”. |
10 | relief | It specifies the type of the border. Default is Flat, other options include RAISED and SUNKEN . |
11 | selectbackground | Background color used for text that has been selected. |
12 | selectborderwidth | Width of border around the selected text. Default is 1. |
13 | selectforeground | The foreground color of the selected text |
14 | show | Controls how the user input appears in the entry box. If you want to make a password entry box, use show = “*”, and all the text will appear to be as * characters. |
15 | State | Default is NORMAL. DISABLED causes the Widget to gray out and go inactive. ACTIVE is the state of the Widget when mouse is over it |
16 | width | Width of the Entry widget in terms of characters. Width = 20 allows for a Entry Field large enough for 20 characters to be shown at once. |
17 | xscrollcommand | If you feel the user may be inputting alot of data, you can use this option to link the widget to a scroll bar to enable scroll. |
18 | set(text) | Used to “set” the value of the text displayed in the entry. Rewrites pre-existing text. |
19 | insert(index, text) | Insert Text at the given index into the entry. |
Tkinter Entry Example
In the example below, we created two Entry fields. Since Entry fields by default show up blank, they need supporting text to explain their purpose to the user. This is usually done through the use of a label, but in some cases, you can use the insert()
function instead. The insert function takes two arguments. The first is the position for the text be inserted and the second is the text which is to be inserted.
from tkinter import *
root = Tk()
root.geometry("200x150")
frame = Frame(root)
frame.pack()
my_entry = Entry(frame, width = 20)
my_entry.insert(0,'Username')
my_entry.pack(padx = 5, pady = 5)
my_entry2 = Entry(frame, width = 15)
my_entry2.insert(0,'password')
my_entry2.pack(padx = 5, pady = 5)
root.mainloop()
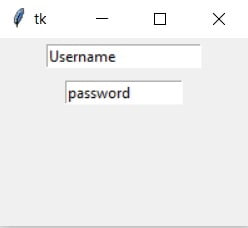
Retrieving Entry Values
Once the user has typed in his input, we need a way to retrieve the input. For this we need a button which the User must trigger. The button calls a function that uses the get()
function to retrieve the input.
from tkinter import *
def retrieve():
print(my_entry.get())
print(my_entry2.get())
root = Tk()
root.geometry("200x150")
frame = Frame(root)
frame.pack()
my_entry = Entry(frame, width = 20)
my_entry.insert(0,'Username')
my_entry.pack(padx = 5, pady = 5)
my_entry2 = Entry(frame, width = 15)
my_entry2.insert(0,'password')
my_entry2.pack(padx = 5, pady = 5)
Button = Button(frame, text = "Submit", command = retrieve)
Button.pack(padx = 5, pady = 5)
root.mainloop()
Try running this code for yourself and see the magic.
This marks the end of our Python Tkinter Entry Box Article. Any suggestions or contributions are more than welcome. Any questions can be asked below in the comments section.
You can head back to the main Tkinter article using this link.