This Article covers the use of the Python Tkinter List box, its parameters, and common events associated with the list box.
What is the Python Tkinter List Box?
A Python Tkinter widget that is used to display a list of options for the user to select. A List Box displays all of its options at once, unlike the ComboBox for instance. Furthermore, all options are in text format.
listbox = Listbox(root, arguments........)
List Box Arguments
No. | Option | Description |
---|---|---|
1 | bg | Background color for the area around the widget. |
2 | bd | Size of the border around the widget. Default value is 2 pixels. |
3 | cursor | When the mouse is hovering over this widget, it can be changed to a special cursor type like an arrow or dot. |
4 | font | The type of font to be used for this widget. |
5 | fg | The color for the text. |
6 | height | Number of lines displayed in the List box. Defaut value is 10. |
7 | highlightcolor | The text color when the widget is under focus. |
8 | relief | It specifies the type of the border. Default is Flat, other options include RAISED and SUNKEN . |
9 | selectbackground | Background color for text that has been selected. |
10 | selectmode | It determines the number of items that can be selected from the list and the effects of the mouse on this selection. It can set to BROWSE, SINGLE, MULTIPLE, EXTENDED. |
11 | width | The width of the widget in characters. |
12 | xscrollcommand | Allows the user to scroll horizontally. |
13 | yscrollcommand | Allows the user to scroll vertically. |
List Box Example 1
Below is a demonstration on how to create a Tkinter List box in Python. It’s syntax is relatively simple. Only the List box object needs to be created and you can keep adding items into it using the insert
function.
from tkinter import *
root = Tk()
root.geometry("200x220")
frame = Frame(root)
frame.pack()
label = Label(root,text = "A list of Grocery items.")
label.pack()
listbox = Listbox(root)
listbox.insert(1,"Bread")
listbox.insert(2, "Milk")
listbox.insert(3, "Meat")
listbox.insert(4, "Cheese")
listbox.insert(5, "Vegetables")
listbox.pack()
root.mainloop()
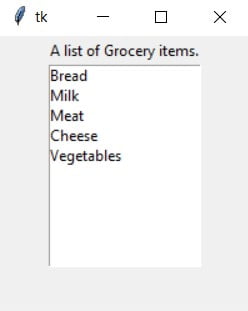
List Box Example 2
In this example we’ll demonstrate how to retrieve the selected List box values. The curselection()
returns the index of the item that is currently selected. Remember that indexing starts from 0.
from tkinter import *
def retrieve():
print(listbox.curselection())
root = Tk()
root.geometry("200x220")
frame = Frame(root)
frame.pack()
label = Label(root,text = "A list of Grocery items.")
label.pack()
listbox = Listbox(root)
listbox.insert(1,"Bread")
listbox.insert(2, "Milk")
listbox.insert(3, "Meat")
listbox.insert(4, "Cheese")
listbox.insert(5, "Vegetables")
listbox.pack()
bttn = Button(frame, text = "Submit", command = retrieve)
bttn.pack(side= "bottom")
root.mainloop()
To retrieve all values in a Tkinter list box, you can use the get() function on the list box object. The get() function takes two inputs, a start and an end point. By using the following code, the get() function will return a list of all the items.
result = listbox.get(0 , END)
print(result)
List Box Example 3
It’s common to implement user interactions with the Listbox, such as single-clicking or double-clicking on an item. This can be done using the appropriate events that are associated with the Tkinter list box.
Single-Click Event Handling:
To handle a single-click event in a Tkinter Listbox, you can bind a function to the <<ListboxSelect>>
event. This event is triggered whenever the user selects an item in the Listbox. You can then retrieve the selected item using the curselection()
method and perform the desired action.
import tkinter as tk
def single_click_handler(event):
selected_index = listbox.curselection()[0]
selected_item = listbox.get(selected_index)
print("Single-clicked on:", selected_item)
root = tk.Tk()
listbox = tk.Listbox(root)
listbox.pack()
# Bind the single-click event to the Listbox
listbox.bind("<<ListboxSelect>>", single_click_handler)
# Add some items to the Listbox
for item in ["Item 1", "Item 2", "Item 3"]:
listbox.insert(tk.END, item)
root.mainloop()
Double-Click Event Handling:
Handling a double-click event in a Tkinter Listbox is similar to handling a single-click event. However, you need to bind a function to the <Double-Button-1>
event, which is triggered when the user double-clicks an item in the Listbox. We have also changed the action that gets performed when a listbox item is deleted. Instead of a simple print message being displayed, the listbox item gets deleted.
import tkinter as tk
def double_click_handler(event):
selected_index = listbox.curselection()[0]
listbox.delete(selected_index)
print("Removed item at index:", selected_index)
root = tk.Tk()
listbox = tk.Listbox(root)
listbox.pack()
# Bind the double-click event to the Listbox
listbox.bind("<Double-Button-1>", double_click_handler)
# Add some items to the Listbox
for item in ["Item 1", "Item 2", "Item 3"]:
listbox.insert(tk.END, item)
root.mainloop()
That concludes our tutorial on the Python Tkinter List Box. You can head back to the main Tkinter article using this link. Any Questions regarding the above content can be asked in the comments section below.