What is a List in Python?
A list is an ordered, mutable collection of items. The “items” within the list may be of different of data types. The items may also be other collection data types like dictionaries, tuples, arrays or even another list. Python lists are a very powerful tool used widely.
Lists are great, but not suitable for every task. There are other data structures out there like arrays, tuples and dictionaries which may be more suitable for certain tasks. First scope out your problem and then decided which data type fits the best. There are also special data structures like “deque” which can offer some performance benefits over lists.
Python Lists Syntax
newlist = ["Python","Java","C++","Ruby"]
The items within the list must be enclosed within square brackets, and each item must be separated by a comma. Unlike arrays, Lists can store any type of data type as well as store variables of different datatypes at the same time.
The double quotes are not part of the syntax of the list. They are only necessery if the item is a string.
List Indexing
You can access the contents of a list by using it’s index. A list is an ordered collection, so the values can be called in the order in which they were placed inside.
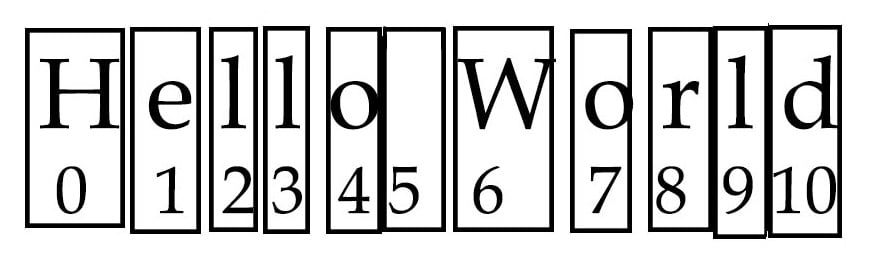
Don’t forget that indexing starts from zero in python. The code below will output “Java”.
newlist = ["Python","Java","C++","Ruby"]
print(newlist[1])
Using negative integers will reverse the order of the list. (Negative indexing starts from -1)
newlist = ["Python","Java","PHP","C++","Ruby"]
print(newlist[-1])
#Output
Ruby
Selecting a range of items from Lists
If you wish to pick up more than just one item in a python list, you can create “slices”. You can specify a range, by giving the starting and ending number. Everything in between will be placed into the slice.
Note that the ending number will not be included in this slice.
newlist = ["Python","Java", "PHP", "C++","Ruby"]
print(newlist[1:4])
# Output
Java
PHP
C++
You don’t nessacerily how to define the starting point, as the default will be 0, or the start of the list. The semi-colon and ending point must be present though.
newlist = ["Python","Java", "PHP", "C++","Ruby"]
print(newlist[:4])
#Output
Python
Java
PHP
C++
It also works the other way around, where you define the start point, and leave out the end point, while keeping the semi-colon.
newlist = ["Python","Java", "PHP", "C++","Ruby"]
print(newlist[2:])
# Output
PHP
C++
Ruby
Updating an Item in a List
Updating an item value in a list is fairly straight forward. Refer to the index where you want to change the value and then assign a value to it.
newlist = ["Python","Java", "PHP", "C++","Ruby"]
newlist[1] = "HTML"
print(newlist)
#Output
["Python","HTML","PHP","C++","Ruby"]
Adding an Item to a List
The append()
adds an item to the end of the list.
newlist = ["Python", "Java","HTML","CSS"]
newlist.append("Ruby")
print(newlist)
#Output
["Python","Java","HTML","CSS","Ruby"]
The insert()
function takes two parameters. The first is the position you want to add a value, and the second parameter is the value itself.
newlist = ["Python", "Java","HTML","CSS"]
newlist.insert(2,"Ruby")
print(newlist)
#Output
["Python","Java","Ruby", "HTML","CSS"]
Note that the former second position item, “HTML” was moved forward to the third position.
Removing an Item from a List
The remove()
function removes a specific item from a list.
newlist = ["Python","Java","Ruby", "HTML","CSS"]
newlist.remove("Java")
print(newlist)
#Output
["Python","Ruby","HTML","CSS"]
Unlike remove()
, the pop()
function takes an index as a parameter, and removes the item at that index. However, the pop function is also often used without any parameters, in which case, it will remove the last item.
newlist = ["Python","Java","Ruby", "HTML","CSS"]
newlist.pop(3)
print(newlist)
#Output
["Python","Java","Ruby","CSS"]
Pop()
function without any parameters.
newlist = ["Python","Java","Ruby", "HTML","CSS"]
newlist.pop()
print(newlist)
#Output
["Python","Java","Ruby","HTML"]
The del()
function has two functions. Like, the pop()
function it can delete an item at a specific index, as well as delete a whole list in one go.
newlist = ["Python","Java","Ruby", "HTML","CSS"]
del newlist
You can delete the value at a specific index using the del keyword in the following manner.
newlist = ["Python","Java","Ruby", "HTML","CSS"]
del newlist[1]
The clear function is used to empty out a list, clearing all the values inside it.
newlist = ["Python","Java","Ruby", "HTML","CSS"]
newlist.clear()
Other Useful Operations
To check for a specific item in a list, you use the membership operator "in"
as shown below.
list = [1,2,3,4,5]
if 2 in list:
print("Found")
Finding the length of a list using the len()
function:
list = [1,2,3,4,5]
length = len(list)
Iterating through a list using a for-loop:
list = [1,2,3,4,5]
for x in list:
print(x)
Sorting a list: Using the sort function you can sort a list in ascending order. Works on both alphabets and numbers. Be careful as this function alters the list itself.
list = ["c","g","z","a"]
list.sort()
print(list)
# Output
['a', 'c', 'g', 'z']
Concatenating two lists using the “+” operator.
list1 = [2,3,4,5]
list2 = ["a","b","c"]
list3 = list1 + list2
Copying a list: The copy()
will copy into list2
all the elements within list1
. In other words, it results in a perfect copy of list1
being made.
list1 = [1,2,3,4]
list2 = list1.copy()
Problems caused due to Shallow References
Sometimes people try to copy lists by simply assigning an existing list to a new variable. This results in a significant problem later on due to how Python Lists work. The concept of referencing comes into play here.
For instance, list2 = list1, does not result in two separate entities with the same values. It results in list2 being assigned a “reference” to list1. What this means that any changes made to either list will be made into the other list. Below is an example.
list1 = [1,2,3,4]
list2 = list1
list1.append(5)
print(list2)
# Output
[1, 2, 3, 4, 5]
Keep in mind that you can safely assign variables to other variables if there are numbers. This problem only occurs in data types like Lists and Dictionaries. This is actually because numbers in Python are immutable, and a new number object is created during such operations.
Extra Examples
Finding the index of an item. The index()
function comes in handy here.
list = [2,3,5,1,2]
print(list.index(3))
Sorting an item in descending order using the reverse
parameter.
list = [2,3,5,1,2]
list.sort()
print(list)
list.sort(reverse = True)
print(list)
# Output
[1, 2, 2, 3, 5]
[5, 3, 2, 2, 1]
List Comprehension
A rather useful technique that you can use to create lists using little code. Here is a small example:
mylist = [i for i in range(1, 10)]
print(mylist)
[1, 2, 3, 4, 5, 6, 7, 8, 9]
To learn more about List comprehension, and see more examples, follow this link to our List comprehension tutorial.
This marks the end of the Python Lists Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.