The orientation of your coordinates actually matters quite alot in libraries that deal with geometry, such as Shapely. If the orientation is incorrect, then there may be some issues, such when you are calculating properties or when plotting your shapely object. In this tutorial, we will teach you about the Shapely orient() function, and how you can use it to avoid this problem(s).
Shapely orient() function on Polygons
We will be using Shapely Polygons to demonstrate a simple problem that happens due to incorrect orientation. When we try to plot a Polygon with incorrect orientation in the plotting library Matplotlib, it results in the interior not being drawn.
This is because geometric/plotting based libraries need the exterior coordinates to be in counter-clockwise order, and the interior coordinates to be in clockwise order.
We will show you how to fix this problem using orient().
First we need to actually import the orient() function, from the shapely.geometry.polygon
module.
from shapely.geometry.polygon import orient
Next lets create our Polygon object, which has both an exterior and an interior.
exterior = [(20, 20), (200, 20), (200, 180), (20, 180)]
interior = [(110, 40), (160, 120), (60, 120)]
object = Polygon(exterior, holes=[interior])
If we plot this Polygon in Matplotlib, we can only see the exterior here. The interior is missing!
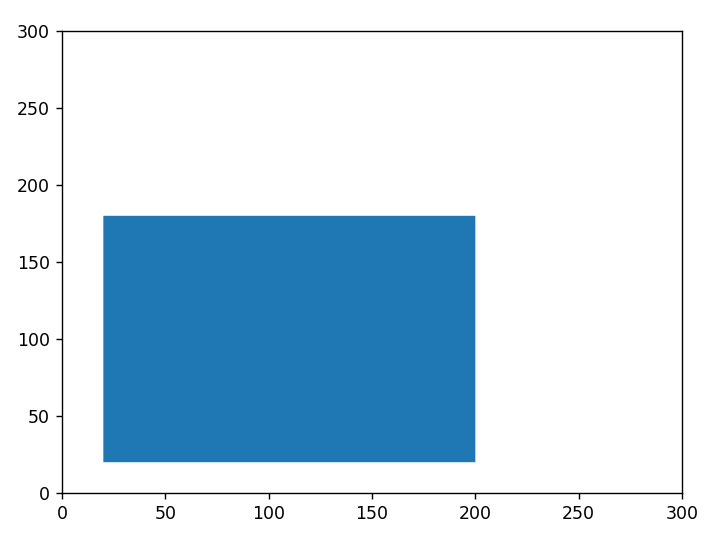
It’s not actually gone, but its not being shown in the resulting figure, because of the incorrect orientation. Let us know try the same thing, but we will wrap our Polygon object, in the orient() function as shown below.
exterior = [(20, 20), (200, 20), (200, 180), (20, 180)]
interior = [(110, 40), (160, 120), (60, 120)]
object = orient(Polygon(exterior, holes=[interior]))
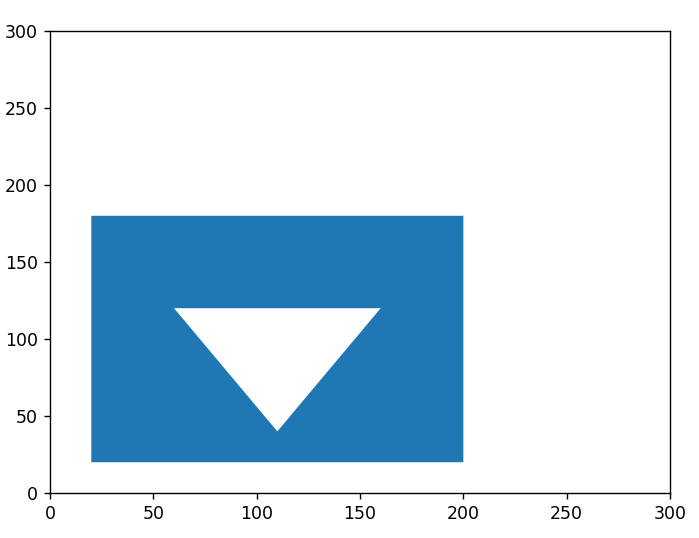
As you can see, its now working! You can extract the coordinates using the exterior.coords
attribute to take a look at the new and properly oriented coordinates.
print(list(object.exterior.coords))
print(list(object.interiors[0].coords))
[(20.0, 20.0), (200.0, 20.0), (200.0, 180.0), (20.0, 180.0), (20.0, 20.0)]
[(110.0, 40.0), (60.0, 120.0), (160.0, 120.0), (110.0, 40.0)]
By default, the orient function will make the exterior coordinates counter-clockwise and the interior coordinates clockwise. If you wish to reverse this, you can pass in “-1” to the sign parameter (which is 1 by default).
orient(polygon, sign = -1)
Happy coding!
This marks the end of the Shapley orient() function Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.