This article explains how to clear the contents of the tkinter entry widget.
The Entry function has a method called delete()
which is used to delete the data currently within the Entry box. Don’t worry, the “delete” function will not in any way delete the widget. That’s what the destroy() function is for.
Syntax
The delete()
method takes two parameters in total. The first is compulsory, and without it an error will occur. The second is optional.
Passing only one parameter allows us to delete a single character using it’s index position. Remember, indexing in Python starts from 0. The below code will delete the second character, located at index 1.
from tkinter import *
def delete():
myentry.delete(1)
root = Tk()
root.geometry('180x120')
myentry = Entry(root, width = 20)
myentry.pack(pady = 5)
mybutton = Button(root, text = "Delete", command = delete)
mybutton.pack(pady = 5)
root.mainloop()
Below is a sample output of what the above code would produce.
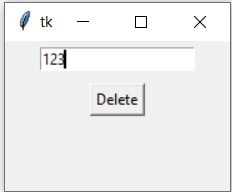
The second parameter defines the end point. Any characters in the between the indexes of the first and second parameters will be deleted (including the characters at the indexes). For instance, passing 1 and 3 into the delete() method will delete the characters at the 1st, 2nd and 3rd Indexes.
An example demonstrating this is shown below.
Example
Below we’ve used a special character called ‘end’. Using this will automatically determine the end point of the contents and set it to that.
The code below will delete all the characters from index 3 to the end of the contents of the entry widget.
from tkinter import *
def delete():
myentry.delete(3, 'end')
root = Tk()
root.geometry('180x120')
myentry = Entry(root, width = 20)
myentry.pack(pady = 5)
mybutton = Button(root, text = "Delete", command = delete)
mybutton.pack(pady = 5)
root.mainloop()
Below we’ve included a short video of us interacting with the output of the above code.
As expected, it will delete characters starting from index “3”. Any characters before that will remain.
Bonus fact: You can also use END
in place of ‘end’. If you didn’t use the import *
statement from tkinter, it will be Tkinter.END
.
This marks the end of the Tkinter Clear Entry article. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article can be asked in the comments section below.
um thanks
yeah
how would you delete the last character of the entry box every time
text = entry.get()
text = text[:-1] # all chars without the last one
entry.insert(0, text)
u should use e.delete() before inserting or it will keep on adding text at first instead of deleting
text = entry.get()
entry.delete(len(text)-1)
I think you can just type