This article explains how to remove widget(s) the Tkinter destroy.
The Tkinter destroy method has the ability to “destroy” any Tkinter GUI objects. This includes widgets like Entry, Button, Toplevel and even the entire Tkinter application.
The syntax is very simple. You simply have to call it as a method on any Tkinter object as shown below.
root.destroy() # Destroys the whole Tkinter application
mylabel.destroy() # Destroys a label
toplevel.destroy() # Destroys a window
The Destroy Function
When used on root
the destroy function will cause Tkinter to exit the mainloop()
and simultaneously destroy all the widgets within the mainloop()
.
from tkinter import *
def close():
root.destroy()
root = Tk()
root.geometry('200x100')
button = Button(root, text = 'Close the window', command = close)
button.pack(pady = 10)
root.mainloop()
The window produced by the above code. Clicking the button will destroy the window, causing it to disappear.
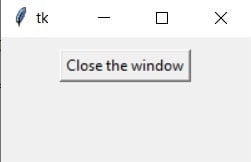
The code below is used simply to destroy a single individual widget.
from tkinter import *
def delete():
mylabel.destroy()
root = Tk()
root.geometry('150x100')
mylabel = Label(root, text = "This is some random text")
mylabel.pack(pady = 5)
mybutton = Button(root, text = "Delete", command = delete)
mybutton.pack(pady = 5)
root.mainloop()
Below is short 3 second video demonstrating the effect of the above code.
This marks the end of the Tkinter destroy widget article. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.
Related Articles:
I don’t understand you