In this tutorial we will discuss the Python Tkinter Config function, which is used to configure certain options in widgets.
When you create a widget in Tkinter, you have to pass in several values as parameters, used to configure certain features for that Widget. The question that may arise at some point, is whether you can change these settings after the widget has been created.
For this we have the Tkinter Config() function, which can be used on any widget to change settings that you may have applied earlier, or haven’t applied yet.
How to use Tkinter Config()
In our first example here, we’ll take a look at a simple use of the Python Tkinter Config() function, used to simply change the text on a label.
The below example features two widgets, a label and a button. The button is linked to a function that calls the config()
on the label when the button is pressed. All you have to do is assign a new value to the option you want changed in the config() function.
import tkinter as tk
root = tk.Tk()
frame = tk.Frame(root)
def dosomething():
mylabel.config(text = "Goodbye World")
mylabel = tk.Label(frame, text = "Hello World", bg = "red")
mylabel.pack(padx = 5, pady = 10)
mybutton = tk.Button(frame, text = "Click Me", command = dosomething)
mybutton.pack(padx = 5, pady = 10)
frame.pack(padx = 5, pady = 5)
root.mainloop()
Before Clicking the Button:
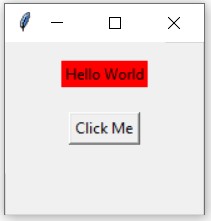
After Clicking the Button:
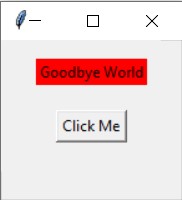
Python Tkinter Config – Continued
Let’s take a further look at using config() to change widgets values.
In the below example we are going to change several things about the widgets, not just the text. We’ll change the color, the background color, as well as the text on both widgets.
import tkinter as tk
root = tk.Tk()
frame = tk.Frame(root)
def dosomething():
mylabel.config(text = "Goodbye World", bg = "blue", fg = "yellow")
mybutton.config(text = "I have been Clicked")
mylabel = tk.Label(frame, text = "Hello World", bg = "red")
mylabel.pack(padx = 5, pady = 10)
mybutton = tk.Button(frame, text = "Click Me", command = dosomething)
mybutton.pack(padx = 5, pady = 10)
frame.pack(padx = 5, pady = 5)
root.mainloop()
Before Clicking the Button:
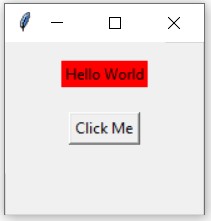
After Clicking the Button:
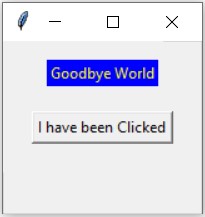
Finding all possible options with Config()
You can also use the config() funciton in an interesting way, to find all possible options for that specific widget. Remember, widgets have some unique options as well, which may not be present in others.
So if you want to see a list of options for a widget, simply call the config() function on that widget, without any parameters as shown below.
import tkinter as tk
root = tk.Tk()
frame = tk.Frame(root)
def dosomething():
print(mylabel.config())
mylabel = tk.Label(frame, text = "Hello World", bg = "red")
mylabel.pack(padx = 5, pady = 10)
mybutton = tk.Button(frame, text = "Click Me", command = dosomething)
mybutton.pack(padx = 5, pady = 10)
frame.pack(padx = 5, pady = 5)
root.mainloop()
Running the above code, and clicking the button will produce the following output in the form of a dictionary.
{'activebackground': ('activebackground', 'activeBackground', 'Foreground', <string object: 'SystemButtonFace'>, 'SystemButtonFace'), 'activeforeground': ('activeforeground', 'activeForeground', 'Background', <string object: 'SystemButtonText'>, 'SystemButtonText'), 'anchor': ('anchor', 'anchor', 'Anchor', <string object: 'center'>, 'center'), 'background': ('background', 'background', 'Background', <string object: 'SystemButtonFace'>, 'red'), 'bd': ('bd', '-borderwidth'), 'bg': ('bg', '-background'), 'bitmap': ('bitmap', 'bitmap', 'Bitmap', '', ''), 'borderwidth': ('borderwidth', 'borderWidth', 'BorderWidth', <string object: '2'>, <string object: '2'>), 'compound': ('compound', 'compound', 'Compound', <string object: 'none'>, 'none'), 'cursor': ('cursor', 'cursor', 'Cursor', '', ''), 'disabledforeground': ('disabledforeground', 'disabledForeground', 'DisabledForeground', <string object: 'SystemDisabledText'>, 'SystemDisabledText'), 'fg': ('fg', '-foreground'), 'font': ('font', 'font', 'Font', <string object: 'TkDefaultFont'>, 'TkDefaultFont'), 'foreground': ('foreground', 'foreground', 'Foreground', <string object: 'SystemButtonText'>, 'SystemButtonText'), 'height': ('height', 'height', 'Height', 0, 0), 'highlightbackground': ('highlightbackground', 'highlightBackground', 'HighlightBackground', <string object: 'SystemButtonFace'>, 'SystemButtonFace'), 'highlightcolor': ('highlightcolor', 'highlightColor', 'HighlightColor', <string object: 'SystemWindowFrame'>, 'SystemWindowFrame'), 'highlightthickness': ('highlightthickness', 'highlightThickness', 'HighlightThickness', <string object: '0'>, <string object: '0'>), 'image': ('image', 'image', 'Image', '', ''), 'justify': ('justify', 'justify', 'Justify', <string object: 'center'>, 'center'), 'padx': ('padx', 'padX', 'Pad', <string object: '1'>, <string object: '1'>), 'pady': ('pady', 'padY', 'Pad', <string object: '1'>, <string object: '1'>), 'relief': ('relief', 'relief', 'Relief', <string object: 'flat'>, 'flat'), 'state': ('state', 'state', 'State', <string object: 'normal'>, 'normal'), 'takefocus': ('takefocus', 'takeFocus', 'TakeFocus', '0', '0'), 'text': ('text', 'text', 'Text', '', 'Hello World'), 'textvariable': ('textvariable', 'textVariable', 'Variable', '', ''), 'underline': ('underline', 'underline', 'Underline', -1, -1), 'width': ('width', 'width', 'Width', 0, 0), 'wraplength': ('wraplength', 'wrapLength', 'WrapLength', <string object: '0'>, <string object: '0'>)}
This line here is responsible for displaying all the possible options for the label widget.
def dosomething():
print(mylabel.config()) # <-----------
If you pay close attention to the output, you’ll also notice the current value of those options are also saved in in the key:value
pairs of the dictionary.
There are other interesting things we can do as well, such as printing out a specific key-value pair as shown below.
def dosomething():
print(mylabel.config()["text"])
This gives out the following value. Can you see the current text value stored in it?
('text', 'text', 'Text', '', 'Hello World')
Now we’ll try to access that current value, which we can do using the following format.
def dosomething():
print(mylabel.config()["text"][4])
This will print it out for us.
Hello World
From my observation, the last value in the tuple is typically the current value.
This marks the end of the Python Tkinter Config. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.
Very nice tutorial bro! Joe rogan approves!
fax
ratio