In this Tkinter Tutorial we will discuss the ttk Separator widget. It’s a very simple widget, that has just one purpose and that is to help “separate” widgets into groups/partitions by drawing a line between them. We can change the orientation of this line (separator) to either horizontal or vertical, and change it’s length/height.
Tkinter Separator – Syntax
The syntax required to create the ttk Separator.
separator = ttk.Separator(parent, **options)
The only widget-specific option this widget has is “orient
“, which can either be tk.VERTICAL or tk.HORIZTONAL, for a vertical and horizontal separator respectively.
Tkinter Separator Example
In this example we will discuss how to create a simple separator.
In this example below we have created two Label widgets, and then created a Horizontal Separator widget to create a division between them.
A few important points to remember; be sure to have the fill and expand option enabled on the separator and it’s container widget. If you do not do this, you likely will not get the desired output as the separator will not be able expand to it’s proper size. Applying these settings on the container widget is important too, because otherwise the separator won’t have any space to expand to.
import tkinter as tk
import tkinter.ttk as ttk
class Window:
def __init__(self, master):
self.master = master
frame = ttk.Frame(self.master)
label = ttk.Label(frame, text = "Hello World")
label.pack(padx = 5)
separator = ttk.Separator(frame,orient= tk.HORIZONTAL)
separator.pack(expand = True, fill = tk.X)
label = ttk.Label(frame, text = "GoodBye World")
label.pack(padx = 5)
frame.pack(padx = 10, pady = 50, expand = True, fill = tk.BOTH)
root = tk.Tk()
root.geometry("200x150")
window = Window(root)
root.mainloop()
The output:
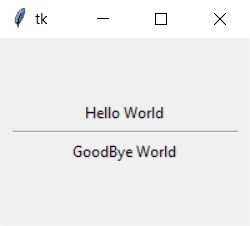
Changing the Orientation
If you want to change the orientation, just the change the value passed into the orient
option from tk.HORIZONTAL
to tk.VERTICAL
. Remember to change the fill from tk.X
to tk.Y
.
import tkinter as tk
import tkinter.ttk as ttk
class Window:
def __init__(self, master):
self.master = master
frame = ttk.Frame(self.master)
label = ttk.Label(frame, text = "Hello World")
label.pack(padx = 5, side = tk.RIGHT)
sep = ttk.Separator(frame,orient= tk.VERTICAL)
sep.pack(expand = True, fill = tk.Y, side = tk.RIGHT)
label = ttk.Label(frame, text = "GoodBye World")
label.pack(padx = 5, side = tk.RIGHT)
frame.pack(padx = 10, pady = 20, expand = True, fill = tk.BOTH)
root = tk.Tk()
root.geometry("200x150")
window = Window(root)
root.mainloop()
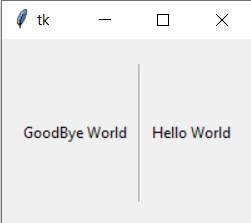
Alternatively, you can also pass “horizontal” or “vertical” into orient.
This marks the end of the Tkinter ttk Separator Widget Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.