Pyinstaller is a really great library in Python, used to create an EXE for your Python code. However, it is quite error prone, and many people have run into alot of constant errors and problems while using Pyinstaller. This article was created for the purpose of addressing many of these commons problems and errors in Pyinstaller, and how to fix them.
Compiling an EXE is quite a complicated task, and even a slight misstep can ruin it. Furthermore, there are some actual problems with Pyinstaller itself that we need to discuss.
Common Problems and Errors in Pyinstaller
There are in total 5 different solutions that we have for resolving problems and errors in Pyinstaller. Ideally you should implement all 5 of these changes/solutions into your code and setup for an idea experience.
Using Auto-py-to-exe
Perhaps you have heard of Auto-py-to-exe before? It’s an alternative library that you can use to compile Python code into EXE’s. But here’s the fun part, it actually uses Pyinstaller in the backend to do everything! So what’s the point of this library then?
Well, it actually just does one thing. It provides you with a GUI interface through you can use Pyinstaller commands. It even generates a Pyinstaller command after you have picked which options you want. You can directly copy paste that command and use it like you would normally use Pyinstaller, or just click the Compile button on Auto-py-to-exe, which automatically does that for you.
Here’s a look at the GUI for auto-py-to-exe.
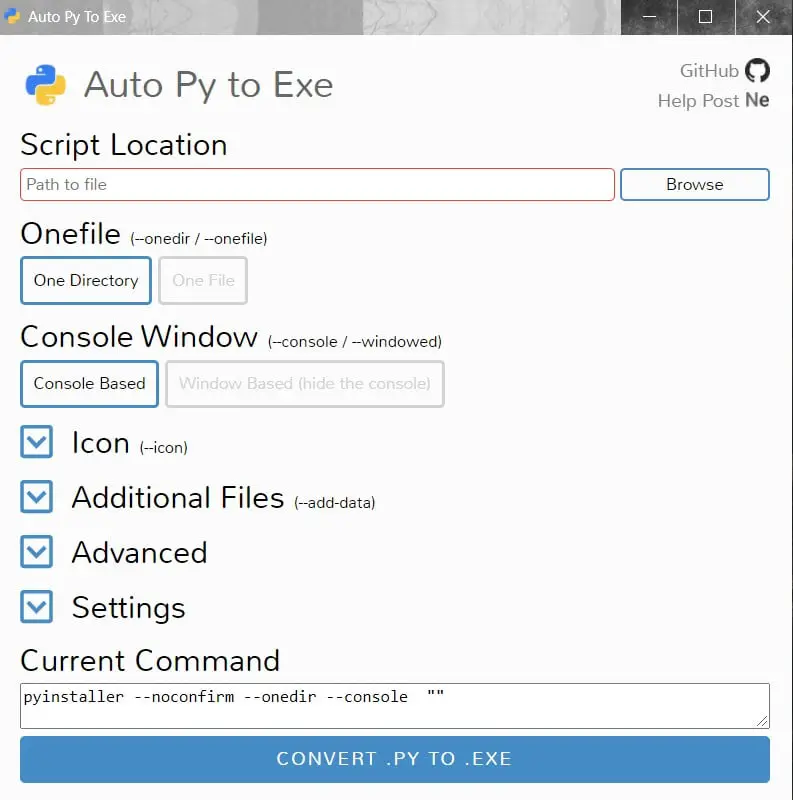
The reason why I’m suggesting this, is because it makes it much easier to use Pyinstaller and is actually less error-prone. It’s also faster to use and is generally considered to be more stable. All in all, a great option for anyone using Pyinstaller.
Developers version of Pyinstaller
Another big problem with Pyinstaller itself, is that there is an issue present with the default pyinstaller version that gets installed when you use “pip”. What you need to do, is run the two below commands in your terminal. This will remove the problem version, and install the developers version which does not have this issue.
pip uninstall pyinstaller
pip install https://github.com/pyinstaller/pyinstaller/archive/develop.zip
Relative File Paths, not Absolute
One mistake I see alot of people doing is hard-coding their file paths when including additional files and folders. That is a terrible mistake to make, because when distributing your exe to other people, there is a very small chance that the filepath will remain valid.
For example, in your code you used the following filepath to load in Image. (Where PythonProgram
is the name of the folder with the script + files)
Image.open("C:/Users/JohnSmith/PythonProgram/Images/image1.jpg")
Now you compiled this into an exe, and gave it to a friend of yours called “Hubbard”. Now Hubbard does not have a user called “John Smith” on his PC, so he is going to run into an error.
Instead we use relative paths. While Hubbard will not have a User called John smith, he will have the PythonProgram
Folder that you give him. So what you can do, is the use the following filepath.
Image.open("/Images/image.jpg")
This removes the need to specify the full file path, and keep it relative. This will ensure it runs on any computer, and in any directory, as long as the contents of the PythonProgram
folder remain the same.
Generate Correct Path when using OneFile
When using the --onefile
option in pyinstaller, there is a slight problem that can occur when including additional files. The correct file is not generated, which results in the additional file you included, not being found.
To resolve this, add the following function to your code, and use it whenever you are using a filepath in your code.
def resource_path(relative_path):
""" Get absolute path to resource, works for dev and for PyInstaller """
try:
# PyInstaller creates a temp folder and stores path in _MEIPASS
base_path = sys._MEIPASS
except Exception:
base_path = os.path.abspath(".")
return os.path.join(base_path, relative_path)
For example, I want to load an image here, so I will pass it through the above function first.
img = Image.open(resource_path("M_Cat_Loaf.jpeg"))
This generates a proper path, that will not cause any errors with Pyinstaller.
Using the OneFile Option
If nothing is working out for you, you can try using the OneFile option instead. Many people have reported this option to work, where as the OneDir option didn’t. So it’s definitely worth trying.
If you are using Auto-py-to-Exe, just click on the OneFile option. Otherwise include the following option in the Pyinstaller command, --onefile
, when you run it in the terminal.
For example:
pyinstaller --onefile pythonfile.py
This marks the end of the “Solving Common Problems and Errors in Pyinstaller” Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.