This tutorial will cover the python library pyinstaller and how to use it.
Before we get into the inner workings of pyinstaller, we’ll have a brief discussion on software distribution. This section is optional and you can skip ahead to the next section if you want.
Software Distribution
There’s a 9 out of 10 chance you’re here because you’re looking for a way to protect your source code while also distributing your precious software to clients and customers. Piracy is an issue that has plagued distributors and developers for years, and it isn’t about to stop any time soon. So how is pyinstaller supposed to help us here?
Normally, you might think to distribute your software, that you have to give someone your source code. Technically yes, you can do it that way. But giving someone your source code allows him to further distribute and modify your software without your consent. (Without you getting any profit out of it)
This is where compilers like pyinstaller come in. Compilers translate source code from a high-level programming language to a lower level language to create an executable program. This compiled program can then be distributed safely as it’s not the source code.
Pyinstaller Setup
Python doesn’t natively come with a way to create compiled programs. However, pyinstaller is a python library that can be used for this purpose. You install it as you would a regular library using the pip install
command as shown below.
pip install pyinstaller
Check our getting started with Python guide if you’re having trouble installing the library.
Understanding Dependencies
Before we move forward you should understand the concept of dependencies in Python. Before using Python on a device, what is an essential step you need to do before this? Install python of course. But what’s to say that your customers/users will have python installed? Those 3rd party libraries you downloaded and installed for your program aren’t included with your source code. We call such requirements, “dependencies”.
The whole point of a compiled program is that it can run without the need for the user to install any additional software before using your program. So how does it handle this? It compiles along with your code, any libraries that you used and allows it to run on a computer which doesn’t have a python interpreter installed. The end result is that the compiled file is much larger than the source code. The more dependencies your source code has, the larger the end file size.
Knowing how dependencies work isn’t required to use this library, but it’s good to know the concepts upon which pyinstaller is based. Furthermore, this knowledge comes in handy if something goes wrong, as you’ll see further on.
Using pyinstaller in Python
Now that you’re ready, go to the command prompt, and navigate to the file you wish to convert. In this case, we’ll be converting a file called “TestFile.py“. First we will navigate to the directory where our File is stored. In our case the file is located in the Desktop.

Once you’ve successfully reached your python file, run the above command, and pyinstaller will take care of the rest.
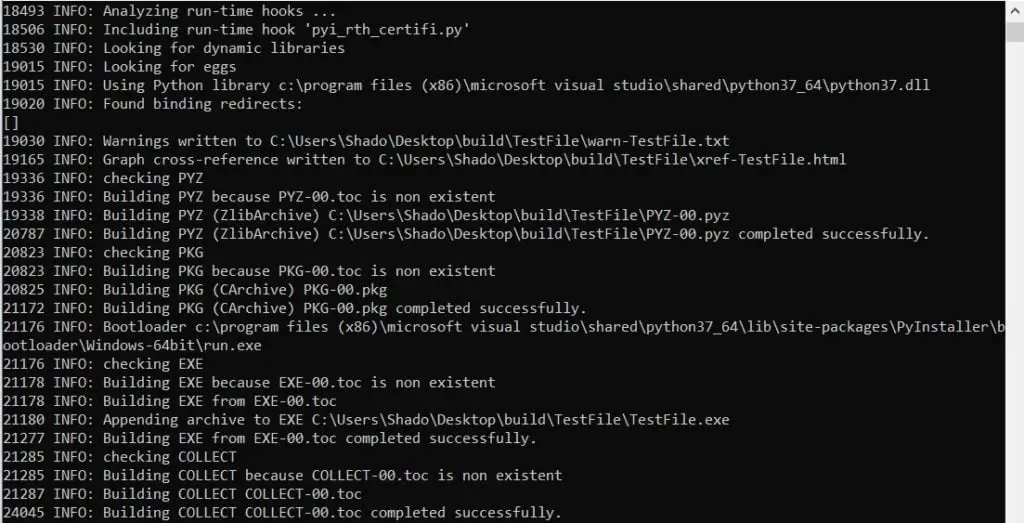
You’ll now see a bunch of folders pop up in the same location as your file. These folders contained your compiled code, your exe
and the dependencies which your code uses. You’ll also find a log file and a list of warnings in there too.
Of course, things may not go as smoothly as we just showed. Maybe you used a library which wasn’t supported by pyinstaller and wasn’t compiled properly. Maybe your exe
file is not working properly. Or maybe you don’t want that console window popping up next to your GUI software. We can’t do anything about non supported libraries, but we can help you with the rest.
Other Settings
This is a setting which I recommend you try if the default method shown above doesn’t work according to how you want it to. The –onefile setting creates a one-file bundled executable which you can use to launch your program.

Other less important settings include the –noconsole setting which prevents any command line windows from showing up when you run the exe
.

You’ll might want this if you’re using a GUI software, as I did once. Alternatively there is also the –console setting, which brings up a command line window when you run the exe
.
Adding Images and other files to the Pyinstaller Exe
Often when bundling your Python application, you might want to include additional files/folders which contain important data. Such as a game with textures and assets that it needs. With Pyinstaller, we need to link of all these such files.
We can do so using the --add-data
to them as shown below.
pyinstaller --add-data "D:/Tutorials/M_Cat_Loaf.jpeg;."
This format works for both individual files, as well as entire folders as well.
Optimizations and Features
There are several optimizations and interesting features you can use to improve your experience with pyinstaller. The optimizations can be a bit lengthy, so we separated them out into separate tutorials for your convenience. Here is our current list of optimizations:
Is this a fool proof solution?
So is this it? Will pyinstaller forever stop any possibility of someone pirating your software? Unfortunately no. There are tools out there which can be used to reverse engineer or de-compile your compiled code back into it’s source code.
Remember, you can never create something that’s piracy proof. What you can do however is make sure the average hacker out there can’t crack your code. We’ve discussed further techniques of protecting your software in our blog post about creating Python software.
Common Problems
This a small section meant to address issues related to the conversion of .py files into exe.
Problems with path: Probably the most common issue, that arises due to a misunderstanding of how file paths work. People often use additional files and images in their software, and then once they have converted the program to an exe, it shows an error about missing files (or something similar).
What are the rules for file paths in Python? If the file or image is in the same directory as your .py file, you only need to write it’s name as shown below.
file = open("data.txt")
But what if it’s not? Then you write the complete path. Remember, this also applies to images and anything else that involves file paths.
file = open("C://Users/CodersLegacy/Desktop/Data.txt")
Most of the time, people simply place the .py and images/files in the same directory. But once you convert the .py to an exe this immediately breaks apart because the directory of the exe file is completely different.
Hopefully, you’ve understand the issue by now. Now to fix it, you can do two things. Once the conversion is complete, you can pick up all the images and files and place them in the same file path as the exe. The exe created by pyinstaller looks something like this (can vary a bit depending on the settings)

The better idea is to create a new folder in the same folder as the exe called Images or Files and store everything in their. Although for this method, you will have to make sure you change the .py prior to conversion. Example shown below.
file = open("/Images/data.txt")
If you have any other issues regarding Python pyinstaller that you want addressed, please mention so in the comments below.
This marks the end of the Python pyinstaller article. Any suggestions or contributions for CodersLegacy are more than welcome. Questions can be directed to the comments section below.