In this tutorial we will discuss how to center a Tkinter Window on the Screen!
It’s rather annoying isn’t it? When you launch your Tkinter program, and it appears somewhere in the corner of the screen. Although this might seem acceptable to you, but it certainly won’t to any end-users you may distribute your software too.
Luckily we have two methods which we will be discussing in this tutorial on how to adjust the Screen position upon startup.
Using the Geometry() Function
Often when making GUI applications with Tkinter, you may want to define a size for the Tkinter Window. Now there are many different ways of doing this, such as using a Frame with a specified size. Another popular and easy way to do so is using the Geometry() function.
The Geometry function can also be extended to adjust the initial starting point of the Window.
In the below example, we have used a couple of useful functions to determine the size of the Screen (e.g: 1920×1080) and then doing some simple calculations to determine where the top-left corner of the Tkinter Window should spawn so that it’s perfectly centered.
import tkinter as tk
root = tk.Tk()
width = 600 # Width
height = 300 # Height
screen_width = root.winfo_screenwidth() # Width of the screen
screen_height = root.winfo_screenheight() # Height of the screen
# Calculate Starting X and Y coordinates for Window
x = (screen_width/2) - (width/2)
y = (screen_height/2) - (height/2)
root.geometry('%dx%d+%d+%d' % (w, h, x, y))
root.mainloop()
Output:
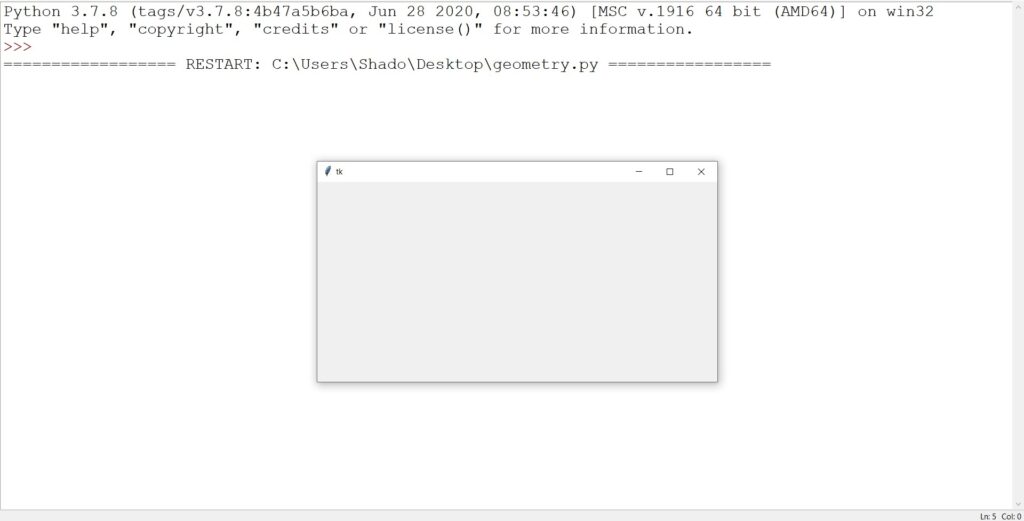
Tkinter eval() function
Another short and very easy way of centering the Tkinter Screen is to use the eval()
function on the Tkinter instance with the appropriate parameter as shown below.
import tkinter as tk
root = tk.Tk()
label = tk.Label(root, text = "A Window")
label.place(x = 70, y = 80)
root.eval('tk::PlaceWindow . center')
root.mainloop()
Try running the code for yourself to the see the magic unfold!
Note: I personally like the first method more, as it gives one alot of control over how things are handled, and can be further customized.
This marks the end of the How to center a Window on the Screen in Tkinter Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.
root.geometry(
'%dx%d+%d+%d'
%
(w, h, x, y)) should be
root.geometry(
'%dx%d+%d+%d'
%
(width, height, x, y))
omg the eval function makes it so easy! thank you so much