In this tutorial we will discuss the Python Tkinter Geometry method, and the various ways in which it can used to manipulate the Tkinter Window on the Screen.
There are two main uses of the geometry()
method, first to adjust the Tkinter Window Size, and secondly to adjust the Tkinter Window’s position on the Screen.
Adjusting Tkinter Screen Size
Often when making GUI applications with Tkinter, you may want to define a size for the Tkinter Window. Now there are many different ways of doing this, such as using a Frame with a specified size. Another popular and easy way to do so is using the Geometry() function.
All you need to do is call the geometry()
function on the Tkinter instance created from the Tk()
function, and pass in the screen size that you want as parameter.
import tkinter as tk
root = tk.Tk()
label = tk.Label(root, text = "A 200x150 Window")
label.place(x = 50, y = 60)
root.geometry('200x150')
root.mainloop()
The output:
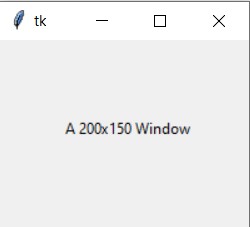
Now let’s try adjusting the size a bit, and try again.
import tkinter as tk
root = tk.Tk()
label = tk.Label(root, text = "A 400x300 Window")
label.place(x = 150, y = 120)
root.geometry('400x300')
root.mainloop()
The output:
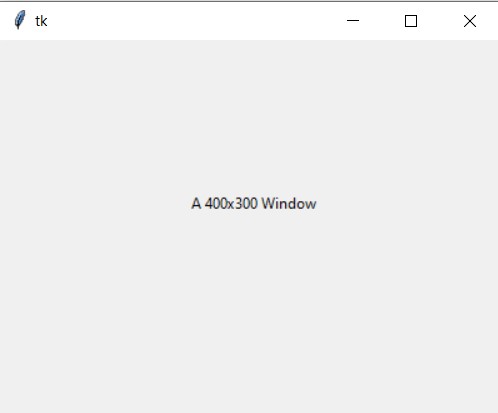
Centering Tkinter Window with Geometry
Another cool use of geometry()
is adjusting the initial startup position for the Window. All we need to do is add two more values into the string passed into the geometry()
. Instead of num1 x num2 it’s going to be num1 + num2 because we are adding an X and Y offset.
We have used a couple of useful functions to determine the size of the Screen (e.g: 1920×1080) and then doing some simple calculations to determine where the top-left corner of the Tkinter Window should spawn so that it’s perfectly centered.
import tkinter as tk
root = tk.Tk()
w = 600 # Width
h = 300 # Height
screen_width = root.winfo_screenwidth() # Width of the screen
screen_height = root.winfo_screenheight() # Height of the screen
# Calculate Starting X and Y coordinates for Window
x = (screen_width/2) - (w/2)
y = (screen_height/2) - (h/2)
root.geometry('%dx%d+%d+%d' % (w, h, x, y))
root.mainloop()
The output: (Try it out on your screen!)
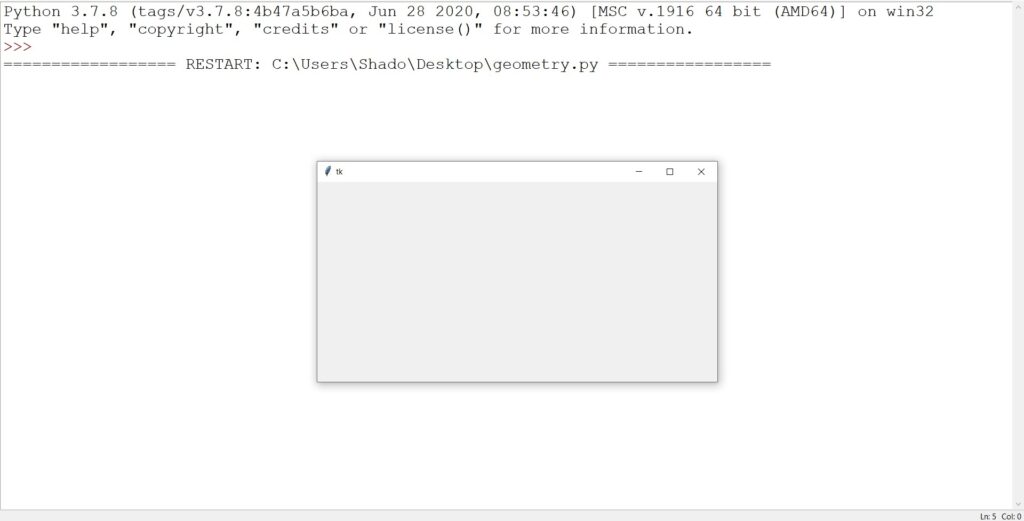
If you think this is too much code for such a simple task, there is another method for centering Windows that you can check out.
This marks the end of the Python Tkinter Geometry Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.