This article covers VB.NET Strings.
Strings are an important Data type for any language due to their ability to store text. VB.NET offers several functions that can be used on strings to find out more about the information they contain and retrieve data from them. We’ll also discuss some string related techniques in this article.
String Length
VB.NET gives us the Len
function which takes a String as it’s parameters and returns the length of that string as an integer value.
Dim s As String
s = "Hello World"
Console.WriteLine(Len(s))
11
String Iterations
Iterating over a String allows us to print out each character from it individually. Often comes in handy when dealing with string parsing and regex.
Module Module1
Sub Main()
Dim s As String
s = "Hello World"
For Each x As String In s
Console.WriteLine(x)
Next
End Sub
End Module
Trimming a String
The Trim function removes all leading and trailing white spaces in a string and returns the result. Keep in mind that this does not alter the original string but returns a new string instead.
s = " Hello World "
Console.WriteLine(s.Trim())
Console.Read()
Changing the Case
The Function ToLower() returns a copy of a string with all characters converted to lowercase.
s = "Hello World"
Console.WriteLine(s.ToLower())
Console.Read()
hello world
The Function ToUpper() returns a copy of a string with all it’s characters converted to uppercase.
s = "Hello World"
Console.WriteLine(s.ToUpper())
Console.Read()
HELLO WORLD
Join function
The Join function is part of the String Class. You can use it to combine two or more strings together to form a larger string. The Join function’s first parameter is called the delimiter. It defines what character will be used between each String.
Below we explore three different types of delimiters and experiment with more than two strings as well.
Module Module1
Sub Main()
Dim a, b As String
a = "Hello"
b = "World"
Console.WriteLine(String.Join("-", a, b))
Console.WriteLine(String.Join("", a, b))
Console.WriteLine(String.Join(" ", "Hi", "Every", "One"))
End Sub
End Module
Hello-World
HelloWorld
Hi Every One
VB.NET String Indexes
Strings in VB.NET have a wide variety of different functions related to their indexes.
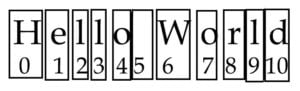
The indexOf
function is applied on a string, and returns the index of the character(s) you wish to find within that string. Only returns the index of the first character that it encounters. Returns -1 if not found.
Dim s As String = "Hello World"
Console.writeline(s.IndexOf('e'))
Console.writeline(s.IndexOf('l'))
Console.writeline(s.IndexOf('a'))
For the character l
only the first index is returned, which is at 2. The l
at index three was not reached.
1
2
-1
You aren’t restricted to just single characters either. You can insert entire Strings in the indexOf
function. Keep in mind however, the index returned will be of the start of the string, or in other words the index of the first character of the string.
Dim s As String = "Hello World"
Console.writeline(s.IndexOf("Hello"))
Console.writeline(s.IndexOf("World"))
0
6
The indexOf
function allows us to use two parameters, where the first is the string or character we’re looking for and the second is the starting position. By default the starting position is 0.
We’ll use this function creatively to find the location of both l’s in Hello World.
Dim s As String = "Hello World"
Dim pos As Integer
Console.WriteLine(s.IndexOf('l'))
pos = s.IndexOf('l')
Console.WriteLine(s.IndexOf('l', pos+1))
First, we found the location of the first l and then did ran the indexOf
function again, starting after the location of the first l
. pos
has the value 2, the location of the first l, so we add one, so that the indexOf
function starts from 3.
Finally, we can add a third parameter called count
. By default the IndexOf
function reads till the end of the String, but count
allows us to control how many characters the function reads from it’s starting position.
Dim s As String = "Hello World"
Console.WriteLine(s.IndexOf("l", 4, 2))
Since only two characters were read, from position 4 and 5, the l and index 9 wasn’t reached.
-1
Insert function
The VB.NET insert function is used to insert a string into another String. All it requires is the target index and the string to be inserted.
Module Module1
Sub Main()
Dim a, b As String
a = "Hello World"
b = "Wonderful "
Dim result As String = a.Insert(6, b)
Console.WriteLine(result)
End Sub
End Module
In the example above we insert the String “Wonderful” into the String “Hello World” at index 6. The result is shown below.
Hello Wonderful World
StartsWith and EndsWith
The startsWith()
function is used on a string to see if starts with a specific string or character. Keep in mind that this function can distinguish between Upper and Lower case characters. In some cases, you might find it useful to convert the string to all upper or lower case before using the startsWith()
function.
s = "Hello World"
Console.WriteLine(s.StartsWith("H"))
Console.WriteLine(s.StartsWith("Hello"))
Console.WriteLine(s.StartsWith("h"))
True
True
False
The opposite of the startsWith()
function, the endsWidth()
checks to see if a string ends with a certain character or string.
s = "Hello World"
Console.WriteLine(s.EndsWith("W"))
Console.WriteLine(s.EndsWith("World"))
Console.WriteLine(s.EndsWith("d"))
False
True
True
VB.NET Split
The VB.NET split
function takes a string as input and splits the string into smaller strings. The splits occur around points we call the delimiter
. By default, the delimiter
is set to a white space (" "
). This setting causes the string to break around all the white spaces in it.
Module Module1
Sub Main()
Dim s As String
Dim kit() As String
s = "Welcome to VB.NET Strings"
kit = Split(s)
For Each x As String In kit
Console.WriteLine(x)
Next
End Sub
End Module
In the code above, we use the split function with it’s default delimiter. This returns an iterable object to use. We then proceed to iterate over it using a For Each loop, printing out each value one by one. (The variable kit is an Array, due to an array being returned from the Split function).
Welcome
to
VB.NET
Strings
Next we’ll try the delimiter with a different value.
Module Module1
Sub Main()
Dim s As String
Dim kittens() As String
s = "1#2#3#4#5"
kittens = Split(s, "#")
For Each x As String In kittens
Console.WriteLine(x)
Next
End Sub
End Module
1
2
3
4
5
This example is pretty self explanatory.
This marks the end of the VB.NET Strings Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Any questions about the tutorial content can be directed to the comments section below.
Like!! I blog frequently and I really thank you for your content. The article has truly peaked my interest.