In this wxPython Tutorial, we will demonstrate how to use the ComboBox Widget, alongside it’s various styles, features and functions. A complete list of options will be included here, alongside several code examples for your convenience.
wxPython ComboBox Syntax
The syntax structure required to create a ComboBox Widget in wxPython.
ComboBox(parent, id, value, pos, size, choices, style, validator, name)
parent
: This is the Widget to which it is parented, such as a Panel.id
: Widget ID. Default value is ID_ANY, which gives it the next available ID.value:
The Text which you want to display on the Widget as the Initial Selection.pos:
A tuple containing the coordinates of where the topleft corner of the StaticText Widget should begin from.size:
A tuple which defines the dimensions of the area occupied by the Text.choices:
A list of values which will be used as Options on the Combobox.style:
Used for styling the Widget
wxPython Combobox Styles
A list of available styles for the ComboBox Widget.
Style | Description |
---|---|
wx.CB_SIMPLE | Creates a combobox with a permanently displayed list (Windows only) |
wx.CB_DROPDOWN | Creates a combobox with a drop-down list |
wx.CB_READONLY | This prevents the User from editing the text and entering a choice of their own. |
wx.CB_SORT | Sorts the entries in the list alphabetically |
wx.TE_PROCESS_ENTER | If enabled, it will enable the generation of the wx.EVT_TEXT_ENTER event. |
wxPython Combobox Methods
A list of available methods for the ComboBox Widget.
Method | Description |
---|---|
FindString(string) | Searches for an Item that matches the given string, and returns it’s index position. |
GetCount() | Returns the number of Items in the ComboBox. |
GetCurrentSelection() | Returns the index number of the Item currently being selected. |
GetStringSelection() | Returns the string label of the currently selected Item. |
GetSelection() | Returns the index number of the selected Item. |
GetString(int) | Returns the Label of the Item at the given index position. |
SetSelection(int) | Sets the selection to the Item at the given index position. |
SetString(int, string) | Sets the Label of the Item at the given index position to the give String. |
SetValue(string) | Sets the text for the combobox text field |
wxPython ComboBox Events
A list of available events for the ComboBox Widget.
Event | Description |
---|---|
EVT_COMBOBOX | Occurs when an Item on the List is Selected. |
EVT_TEXT | Occurs when the Text on the ComboBox is changed. |
EVT_TEXT_ENTER | Occurs when RETURN is pressed in the ComboBox. |
EVT_COMBOBOX_DROPDOWN | Occurs when the Drop Down Menu on the ComboBox is displayed. |
EVT_COMBOBOX_CLOSEUP | Occurs when the Drop Down Menu on the ComboBox closes. |
Example Code
In this example we create a basic ComboBox widget and bind it to a function.
After creating the base code, we create a list of Strings, which will be used as options on the ComboBox. As the initial value, we simply assign the first value in the array.
Lastly, we bind the ComboBox to the ComboBoxEvent()
function, which prints out the label of the currently selected item.
import wx
class Window(wx.Frame):
def __init__(self, title):
super().__init__(parent = None, title = title)
self.panel = wx.Panel(self)
values = ["Butter", "Milk", "Biscuits", "Cheese"]
cb = wx.ComboBox(self.panel, value = values[0], choices = values, pos = (50, 50))
cb.Bind(wx.EVT_COMBOBOX, self.ComboBoxEvent)
self.Centre()
self.Show()
def ComboBoxEvent(self, e):
cb = e.GetEventObject()
print(cb.GetStringSelection())
app = wx.App()
window = Window("WxPython Tutorial")
app.MainLoop()
The output of the above code:
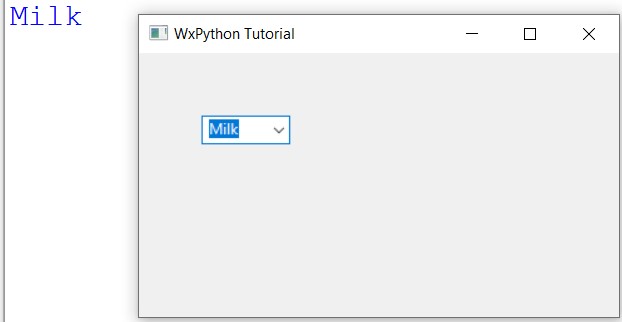
This marks the end of the wxPython ComboBox Widget Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.