In this wxPython Tutorial, we will demonstrate how to create a Menu using Menu and MenuBar Widgets, alongside it’s various styles, features and functions. A complete list of options will be included here, alongside several code examples for your convenience.
Creating a Simple Menu
In this example we’ll create a simple MenuBar with a File Menu.
class Window(wx.Frame):
def __init__(self, title):
super().__init__(parent = None, title = title)
self.panel = wx.Panel(self)
#--------------
menuBar = wx.MenuBar()
fileMenu = wx.Menu()
newFileItem = fileMenu.Append(wx.ID_NEW, '&New\tCTRL+N', "Create New File")
openFileItem = fileMenu.Append(wx.ID_OPEN, '&Open\tCTRL+O', "Open File")
saveFileItem = fileMenu.Append(wx.ID_SAVE, '&Save\tCTRL+S', "Save File")
self.Bind(wx.EVT_MENU, self.NewFile, newFileItem)
self.Bind(wx.EVT_MENU, self.OpenFile, openFileItem)
self.Bind(wx.EVT_MENU, self.SaveFile, saveFileItem)
menuBar.Append(fileMenu, 'File')
#----------------
self.SetMenuBar(menuBar)
self.Centre()
self.Show()
def NewFile(self, e):
print("New File")
def OpenFile(self, e):
print("Open File")
def SaveFile(self, e):
print("Save File")
app = wx.App()
window = Window("WxPython Tutorial")
app.MainLoop()
menuBar = wx.MenuBar()
We start off by creating a wx.MenuBar()
called menuBar
. Any Menu’s that we create later will be added into this MenuBar.
fileMenu = wx.Menu()
newFileItem = fileMenu.Append(wx.ID_NEW, '&New\tCTRL+N', "Create New File")
openFileItem = fileMenu.Append(wx.ID_OPEN, '&Open\tCTRL+O', "Open File")
saveFileItem = fileMenu.Append(wx.ID_SAVE, '&Save\tCTRL+S', "Save File")
Next we create a Menu called fileMenu
with a bunch of file related commands. We create three “MenuItems” for this Menu using the Append()
function. (The Append
function returns a Menu Item)
The Append Function takes three parameters. The first parameter is the ID of the MenuItem. We’ve used the standard ID’s for New, Open and Save. The second parameter takes a string for the text to appear on the MenuItem. (The \tCTRL + N part is for the shortcut).
Lastly, the third parameter is the “help message” text which will appear if a status bar is present. (optional)
self.Bind(wx.EVT_MENU, self.NewFile, newFileItem)
self.Bind(wx.EVT_MENU, self.OpenFile, openFileItem)
self.Bind(wx.EVT_MENU, self.SaveFile, saveFileItem)
Here we Bind our MenuItem’s to wx.EVT_MENU
and the appropriate functions which we want called.
To make things easier, just remember the first parameter will always be wx.EVT_MENU
, the second will be the function we want called when the MenuItem is clicked, and the third is the MenuItem we are binding to.
menuBar.Append(fileMenu, 'File')
#----------------
self.SetMenuBar(menuBar)
Lastly, we append the FileMenu to the MenuBar, then set the MenuBar to the Frame. (It won’t appear on the Window otherwise)
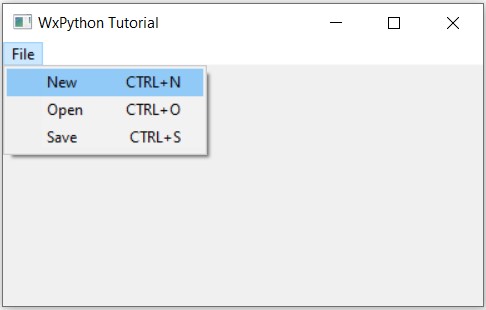
(On certain systems, using the proper ID’s will automatically insert proper images and shortcuts into the MenuItem’s)
Creating a Submenu
Here we will create a Submenu, which is basically a menu within a menu.
All we have to do is create a new Menu, add in some items to it, and then use the AppendSubMenu()
function on our parent Menu. The AppendSubMenu()
function takes two parameters, the Submenu to be appended, and the name of the Submenu.
import wx
class Window(wx.Frame):
def __init__(self, title):
super().__init__(parent = None, title = title)
self.panel = wx.Panel(self)
#--------------
menuBar = wx.MenuBar()
fileMenu = wx.Menu()
fileMenu.Append(wx.ID_NEW, '&New\tCTRL+N', "Create New File")
fileMenu.Append(wx.ID_OPEN, '&Open\tCTRL+O', "Open File")
fileMenu.Append(wx.ID_SAVE, '&Save\tCTRL+S', "Save File")
subMenu = wx.Menu()
subMenu.Append(wx.ID_ANY, "Recent Files", "View Recent Files")
subMenu.Append(wx.ID_ANY, "Import File", "Import external file")
subMenu.Append(wx.ID_ANY, "Export File")
fileMenu.AppendSubMenu(subMenu, "File Options")
menuBar.Append(fileMenu, 'File')
#----------------
self.SetMenuBar(menuBar)
self.Centre()
self.Show()
app = wx.App()
window = Window("WxPython Tutorial")
app.MainLoop()
We’ve used the default ID wx.ID_ANY
because there are no standard ID’s which are available for making new Menu Items.
The output:
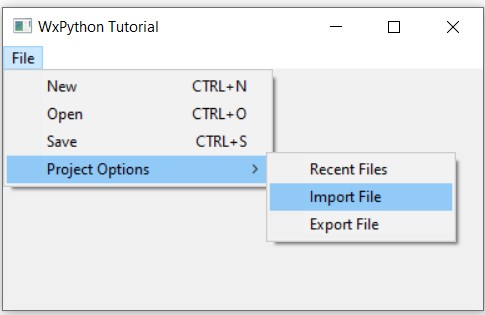
Appending other Items
In this example code, we just have one small change. Instead of using Append()
, we can also use AppendCheckItem()
, AppendRadioItem()
or AppendSeparator()
, which will add a CheckButton, a RadioButton and a separator line respectively.
The syntax for AppendCheckItem()
and AppendRadioButton()
is identical to that of Append()
, whereas AppendSeparator()
takes no parameters.
import wx
class Window(wx.Frame):
def __init__(self, title):
super().__init__(parent = None, title = title)
self.panel = wx.Panel(self)
#--------------
menuBar = wx.MenuBar()
fileMenu = wx.Menu()
fileMenu.Append(wx.ID_NEW, '&New\tCTRL+N', "Create New File")
fileMenu.Append(wx.ID_OPEN, '&Open\tCTRL+O', "Open File")
fileMenu.Append(wx.ID_SAVE, '&Save\tCTRL+S', "Save File")
fileMenu.AppendSeparator()
subMenu = wx.Menu()
subMenu.AppendCheckItem(wx.ID_ANY, "Auto Save", "Auto Save every 5 mins")
subMenu.AppendCheckItem(wx.ID_ANY, "Dark Mode", "Enable Dark Theme")
subMenu.AppendCheckItem(wx.ID_ANY, "Enlarge Text", "Increase Text Size")
fileMenu.AppendSubMenu(subMenu, "Project Options")
menuBar.Append(fileMenu, 'File')
#----------------
self.SetMenuBar(menuBar)
self.Centre()
self.Show()
app = wx.App()
window = Window("WxPython Tutorial")
app.MainLoop()
The output: (Can you see the separator in the menu below?)
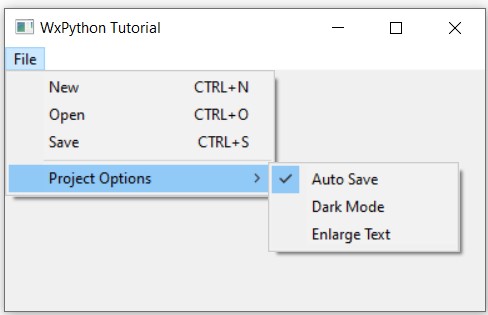
Using MenuItem
Besides the wxPython Menu and MenuBar classes, we also have MenuItem. Normally we don’t need to explicitly define one of these, as the Append()
functions return a MenuItem object. If you wish to create the MenuItem separately though, the below example will show you how.
(We’ve also used a Bitmap image which we have attached to the MenuItem using the SetBitmap()
function).
import wx
class Window(wx.Frame):
def __init__(self, title):
super().__init__(parent = None, title = title)
self.panel = wx.Panel(self)
#--------------
menuBar = wx.MenuBar()
fileMenu = wx.Menu()
exitItem = wx.MenuItem(fileMenu, wx.ID_EXIT, '&Quit\tCtrl+Q')
exitItem.SetBitmap(wx.Bitmap("deleteSticky.bmp"))
fileMenu.Append(exitItem)
self.Bind(wx.EVT_MENU, self.onQuit, exitItem)
menuBar.Append(fileMenu, 'File')
#----------------
self.SetMenuBar(menuBar)
self.Centre()
self.Show()
def onQuit(self, e):
print("Exit Window")
self.Close()
app = wx.App()
window = Window("WxPython Tutorial")
app.MainLoop()
The output:
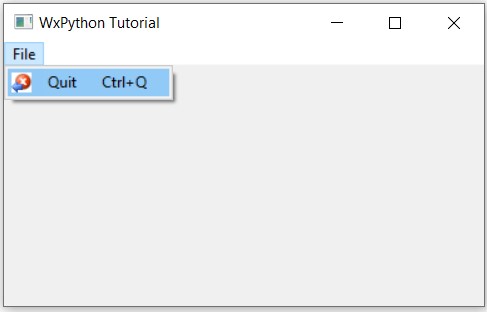
wxPython Menu Methods
A list of available methods for the Menu Widget.
Method | Description |
---|---|
Append(id, label, help) | Appends an Item to the Menu. |
AppendCheckItem(id, label, help ) | Appends a CheckButton Item to the Menu. |
AppendRadioItem(id, label, help ) | Appends a RadioButton Item to the Menu |
AppendSeparator(id, label, help ) | Appends a Separator to the Menu |
AppendSubMenu(menu, label) | Appends another menu as a submenu. |
Break() | Adds a break into the Menu, causing the next Item to appear in a new column. |
Delete(id) | Deletes the item at the specified index. |
Enable(id, bool) | Enables/Disables the item at the specified index. |
GetLabel(id) | Returns the string value of the text of the Item at the specified index. |
Insert(id, item) | Inserts an item at the specified index. (Similar variants exists for other items, like InsertCheckItem()) |
SetLabel(id, label) | Sets the label of the item at the specified index. |
wxPython MenuBar Methods
A list of available methods for the MenuBar Widget.
Method | Description |
---|---|
EnableTop(id, bool) | Enables/Disables the Menu at the given index. |
GetMenu(id) | Returns the Menu at the given index. |
GetMenuCount() | Returns the number of Menu’s in the MenuBar |
Insert(id, menu, title) | Inserts a menu item at the given index, with the specified name (title). |
This marks the end of the wxPython Menu with MenuBar and MenuButton Widgets Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.