Selenium is a powerful tool for automating web applications, but one common challenge faced by automation testers is dealing with browser caching. Caching can sometimes interfere with the accuracy of your Selenium tests, leading to unexpected results. In this tutorial, we’ll explore how to disable the cache in Selenium to ensure that your automated tests run smoothly and produce reliable results.
Why Disable Cache in Selenium?
Browser caching is a mechanism that stores copies of web pages to reduce loading times. While this is beneficial for regular browsing, it can be a hindrance during automated testing.
For example, during stress testing for a website, if the cache is used after the initial page load, then it will not be a good stress test, as the only time the server is used, is the initial page load. Furthermore, caching may lead to outdated content being served, making it difficult to verify the correctness of your web application through Selenium tests.
How to Disable the Browser Cache in Selenium
Disabling the browser cache is no easy task. Selenium itself has little control over the browser cache, thus we cannot directly disable it. It also does not help that other factors come into play and make things difficult, such as different browsers exhibiting different behaviors, and different versions for each browser causing certain methods to fail or become outdated.
There are various techniques that we have compiled here for you to try out, with a short description on how they work, and what effect they have on the cache.
1. Setting cache size to 0
This sets the size of the cache to 0, ideally preventing the cache from ever forming.
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service as ChromeService
from selenium import webdriver
chrome_options = webdriver.ChromeOptions()
chrome_options.add_argument("--disk-cache-size=0") <--
service = ChromeService(executable_path=ChromeDriverManager().install())
driver = webdriver.Chrome(service=service, options=chrome_options)
Note, that arguments can vary from browser to browser. This may not work for other browsers other than Chrome.
2. Use incognito mode
Activating incognito mode disables many things like cache and cookies.
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service as ChromeService
from selenium import webdriver
chrome_options = webdriver.ChromeOptions()
chrome_options.add_argument("--incognito") <--
service = ChromeService(executable_path=ChromeDriverManager().install())
driver = webdriver.Chrome(service=service, options=chrome_options)
For Firefox, you will use the –private argument instead. Other browsers may have different versions of this argument too.
from webdriver_manager.firefox import GeckoDriverManager
from selenium.webdriver.firefox.service import Service as FirefoxService
from selenium import webdriver
firefox_options = webdriver.FirefoxOptions()
firefox_options.add_argument('--private')
service = FirefoxService(executable_path=GeckoDriverManager().install())
driver = webdriver.Chrome(service=service, options=firefox_options)
3. Forcing a hard Refresh
You can re-load the page without the cache in most browser by using a keyboard shortcut. For Chrome and Firefox, the shortcut is CTRL + SHIFT + R (command instead of control for apple devices). These keys must be sent on an element however, so locate an important/big container element on the web page you are accessing (e.g. body), and send these keys on that element.
driver.get("https://example.com")
element = driver.find_element(By.XPATH, "//body")
element.send_keys(Keys.CONTROL, Keys.SHIFT, "R")
CTRL + F5 is another popular shortcut for refreshing that works on most browsers.
4. Automate the Clear Cache Action
One foolproof method of bypassing the cache, is to simply automate the clear cache action in the browser, the way you normally would (as a human). On the Chrome Browser, if you visit the URL, chrome://settings/clearBrowserData, you will see the following window popup.
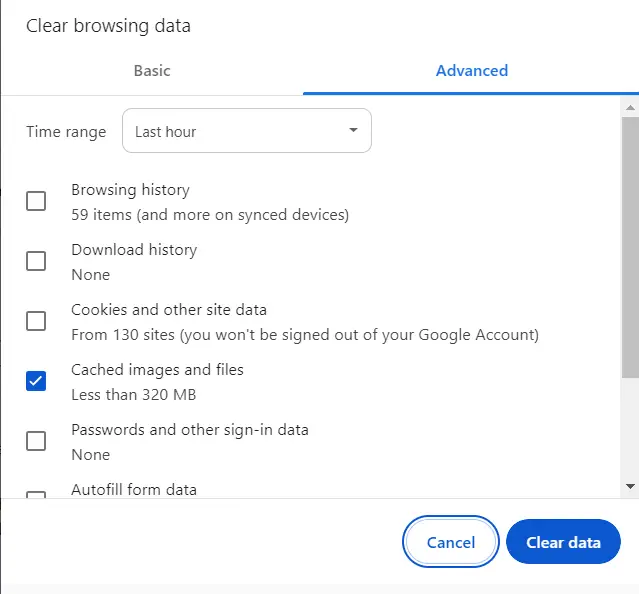
All we have to do is automate the “click” action for that button. This can be done with the following code:
import time
from selenium.webdriver.common.keys import Keys
from selenium.webdriver.common.actions import mouse_button
from selenium.webdriver.common.by import By
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service as ChromeService
from selenium import webdriver
chrome_options = webdriver.ChromeOptions()
service = ChromeService(executable_path=ChromeDriverManager().install())
driver = webdriver.Chrome(service=service, options=chrome_options)
driver.get("chrome://settings/clearBrowserData")
element = driver.find_element(By.XPATH, "//settings-ui")
time.sleep(1)
element.send_keys(mouse_button.MouseButton.LEFT)
element.send_keys(Keys.ENTER)
time.sleep(3)
driver.quit()
This code looks a little complex, because of the various imports and setup statements required. It is also a bit complicated due to the fact that Chrome uses a rather strange way of creating the popup, meaning we cannot directly access the button. Hence, we have to select the popup container (settings-ui), click on it (left mouse button) to focus it, and then the enter button to submit the clear cache request.
This marks the end of the “How to disable the Cache in Selenium” Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comment section below.