This is a tutorial on the JavaFX ProgressBar component.
Progress bars is a great way to visualize progression of computer operations such as file transferring, downloading, uploading, copying etc. In contrast, text based progress meters are rather bland and boring.
Progress Bars in JavaFX, have a range of values ranging from 0.0
to 1.0
which represent the “progress” of the progressbar. 0.0
represents zero progress, or an empty bar where 1.0
represents a full progressbar.
The JavaFX ProgressBar is represented by the javafx.scene.control.ProgressBar
class.
JavaFX ProgressBar Example
In this section we’ll explain how to create progress-bars, increase (set) their progress and return (get) the progress value.
In this example below, we’ll create two buttons, one for increasing the value of the ProgressBar using the setProgress()
method and the other button gets or “returns” the value of the progress bar using the getProgress()
method.
You should always initialize your progress bars from zero, rather than leaving the brackets of the ProgressBar class empty. This will cause problems if you try using the getProgress()
method and you haven’t defined a value.
package application;
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.ProgressBar;
import javafx.scene.layout.GridPane;
public class Tutorial extends Application {
public static void main(String args[]){
launch(args);
}
@Override
public void start(Stage primaryStage) throws Exception {
ProgressBar progress = new ProgressBar(0);
Button button1 = new Button("Increase");
Button button2 = new Button("Get Value");
button1.setOnAction(e -> {
progress.setProgress(progress.getProgress() + 0.1);
});
button2.setOnAction(e -> {
System.out.println(progress.getProgress());
});
GridPane layout = new GridPane();
layout.setPadding(new Insets(20, 20, 20, 20));
layout.setHgap(10);
layout.setVgap(10);
layout.add(button1, 1, 0);
layout.add(button2, 1, 1);
layout.add(progress, 0, 0)
Scene scene = new Scene(layout, 250, 200);
primaryStage.setTitle("CodersLegacy");
primaryStage.setScene(scene);
primaryStage.show();
}
}
The GUI output of the above progressbar example:
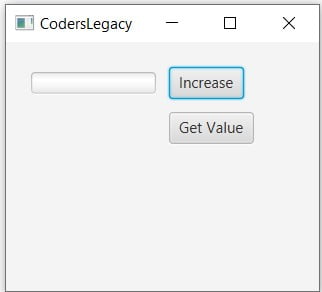
After clicking the increase the button a few times:
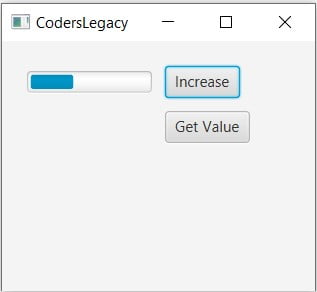
Normally you wouldn’t tie this to a button though, rather an action such as files being copied or tasks that are being complicated.
If you don’t define any value and leave the parameters blank, the progress bar will not appear empty (like it would if the value was 0). There will be a moving blue dot that represents the fact that it is undefined.
You can use the isIndeterminate() method to find out whether or whether not the value of the JavaFX ProgressBar is is Indeterminate or not. Indeterminate means undefined or not set.
Other JavaFX Widgets:
- JavaFX Hyperlink
- JavaFX DatePicker
- JavaFX FileChooser
- JavaFX Font
- JavaFX Layout Tutorial
This marks the end of the JavaFX ProgressBar Tutorial. Any suggestions or contributions for CodersLegacy are than welcome. Questions regarding the article can be asked in the comments section.