This article explains the JavaFX StackPane layout.
JavaFX StackPane is a rather unusual layout component when compared to the others. As the name implies, StackPane “stacks” all the components in it onto each other. For this reason, the kind of GUI components we use with StackPane will be completely different.
StackPane can be imported using the javafx.scene.layout.StackPane
name.
StackPane Layout Example
In this StackPane layout example, we create a Rectangle and a Circle using the Rectangle and Circles classes respectively. Next we use the setFill()
to actually give them some color and appearance. Finally we pass the three GUI components we created (including a label) into the StackPane class we finish it.
package application;
import javafx.application.Application;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.layout.StackPane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
import javafx.scene.shape.Rectangle;
import javafx.scene.text.Font;
public class Tutorial extends Application {
public static void main(String args[]){
launch(args);
}
@Override
public void start(Stage primaryStage) throws Exception {
Label label = new Label("A StackPane Example");
label.setFont(new Font(30));
// Creates a YELLOW Circle
Circle circle = new Circle(100, 100, 70);
circle.setFill(Color.YELLOW);
// Creates a BLUE Rectangle
Rectangle rectangle = new Rectangle(100, 100, 300, 160);
rectangle.setFill(Color.LIGHTBLUE);
StackPane layout = new StackPane(circle, rectangle, label);
Scene scene = new Scene(layout, 400, 250);
primaryStage.setTitle("CodersLegacy");
primaryStage.setScene(scene);
primaryStage.show();
}
}
The GUI output of the above code:
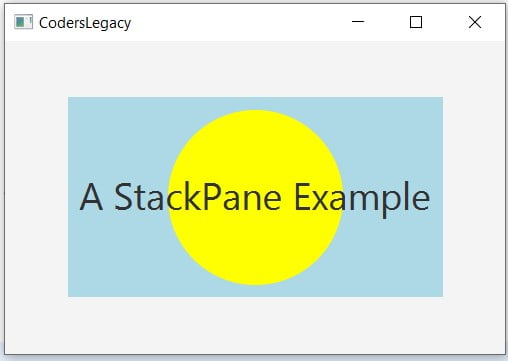
The only situation I would use regular JavaFX widget with StackPane if it was just one. StackPane will nicely center the widget without us having to add any extra code. A small example is shown below.
TextField textfield = new TextField();
textfield.setMaxWidth(100);
StackPane layout = new StackPane(textfield);
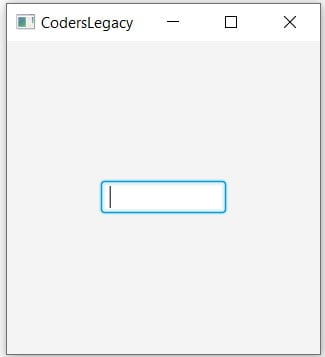
Alignment Options
Although StackPane by default centers all of it’s components, you can change this using setAlignment()
function and the different alignment options in JavaFX that we’ve listed below.
- Pos.BASELINE_LEFT
- Pos.BASELINE_RIGHT
- Pos.BASELINE_CENTER
- Pos.BOTTOM_LEFT
- Pos.BOTTOM_RIGHT
- Pos.BOTTOM_CENTER
- Pos.CENTER_LEFT
- Pos.CENTER_RIGHT
- Pos.CENTER
- Pos.TOP_LEFT
- Pos.TOP_RIGHT
- Pos.TOP_CENTER
A small example of the Alignment function in StackPane.
layout.setAlignment(Pos.BOTTOM_CENTER);
Adding the above line resulted in the following GUI.
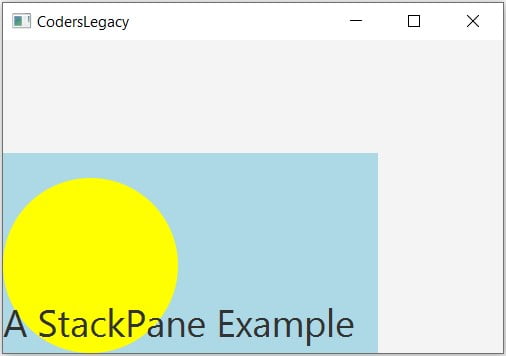
StackPane Methods
A list of common, but important methods for the StackPane component.
Method | Description |
---|---|
setAlignment(Pos) | Used to set the alignment of the StackPane. |
setAlignment(Node, Pos) | Used to set the alignment of a child component. |
getAlignment() | Returns the alignment position of the StackPane. |
getAlignment(Node) | Returns the alignment of the specified child component. |
setMargin(Node, Insets) | Used to assign margins to a child component. |
getMargin(Node) | Returns the Insets value of the child component. |
Learn about the other layout components:
- VBox Layout
- HBox Layout
- TilePane Layout
This marks the end of the JavaFX StackPane tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.