Welcome to this tutorial on how to change the taskbar icon in Tkinter! The taskbar icon, also known as the application icon or window icon, is the small image that appears on the taskbar and in the window title bar. By default, Tkinter uses the system’s default icon for its windows. However, you might want to customize this icon to give your application a more personalized look.
In this tutorial, we’ll walk you through the process of changing the taskbar icon step-by-step.
Prepare Your Icon Image
Regardless of the method we use, the first step is to prepare the icon that you want to use for your application. The icon image should be in the .ico
format, which is the standard format for icons on Windows. You can create or find suitable icons using various graphic design tools or online resources.
Method 1: Using ctypes
(Windows Only)
This method involves using the ctypes
library to modify the App User Model ID (AppID) of the current process. This AppID is used by the Windows operating system to determine how to display the application in the taskbar and other UI elements.
Step 1: Import the ctypes
module
Begin by importing the ctypes
module, which provides a way to access functions in shared libraries (DLLs) on Windows.
import ctypes
Step 2: Define and Set your AppID
Create a unique identifier for your application. Replace 'mycompany.myproduct.subproduct.version'
with your actual identifier (while maintaining the same format). Alternatively, you could just use this placeholder string.
myappid = u'mycompany.myproduct.subproduct.version'
ctypes.windll.shell32.SetCurrentProcessExplicitAppUserModelID(myappid)
It should be in Unicode however, which is why we have added the prefix “u” to our string.
Now we need to create our Tkinter application, and set the window icon (the one that appears on the window’s top-left corner).
Step 4: Create Your Tkinter Application
Create a basic Tkinter application as you normally would. This includes creating the main application window, setting its title, dimensions, and any other desired properties. Import the tkinter
module and other necessary modules at the beginning of your script.
import tkinter as tk
from PIL import Image, ImageTk
# Create the main application window
root = tk.Tk()
root.title("Change Taskbar Icon Tutorial")
Step 5: Load and set the Window Icon Image
Load your icon image using the ImageTk
class from the PIL
module. This class allows you to load image files, including ICO files. It is compatible with more file types than the standard Tkinter PhotoImage class, and more reliable.
Here is the icon that we are using, called bulb.ico
:

Our code:
# Load the icon image using PIL
icon = Image.open("bulb.ico")
icon = ImageTk.PhotoImage(icon)
# Set the taskbar icon
root.iconphoto(True, icon)
root.mainloop()
Here is a screenshot showing updated taskbar icon:
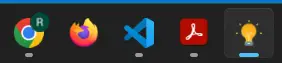
Note: You don’t have to use .ico
files. Other filetypes work too.
Method 2: Use a .PY to EXE converter like PyInstaller
PyInstaller is a popular Python library used to convert Python scripts into standalone executable applications. It’s particularly useful for distributing applications to users who might not have Python installed on their systems. In the context of changing the taskbar icon in a Tkinter application, using PyInstaller can help package your application with the necessary icon files and dependencies.
Step 1: Install PyInstaller
If you haven’t already, install PyInstaller using pip:
pip install pyinstaller
Step 2: Prepare your Tkinter application
Your Python code does not effect the icon created by PyInstaller, so your code doesn’t matter. However, it will be a good idea to add the icon as a window icon in your tkinter application (the one that appears in the top-left corner) so that it matches the taskbar icon.
Step 3: Compile your application using PyInstaller
You need to compile your application using PyInstaller, with an additional command line argument that defines the icon for your executable.
pyinstaller --icon=bulb.ico myscript.py
Conclusion
Congratulations! You’ve successfully learned how to change the taskbar icon in Tkinter. By following these steps, you can now customize the icon of your Tkinter application to give it a more distinct appearance. Remember that icons should be in the .ico
format, and you can use the PIL
library to load and manipulate images for your application. Have fun customizing your Tkinter applications!