In this tutorial we will take a look at how to send emails in Gmail using a Python program. Automation is one of the powerful tools in today’s world, and automating tasks such as receiving and sending emails in Python is just one of many examples.
Account Security
Due to security settings within Gmail, you will not be able to login using your Python script. This is to prevent unauthorized apps from accessing your Gmail account. There are two ways we can deal with this problem.
The easier way is to simply visit this link, and change your security settings for apps on Google. Just click on the button to allow less secure apps to be able to access your Gmail account. (Of course, any app will still need the login details so it’s not going to let just anyone or anything access your account).
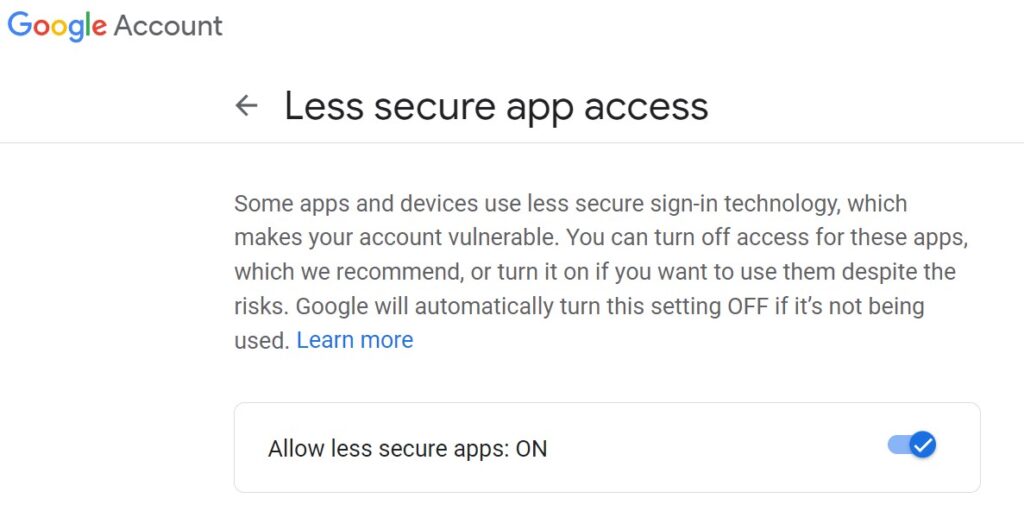
This is not the best approach however. You might want to use proper authentication by setting up a Google Cloud Project, especially if you are distributing applications to customers or something similar. It’s alot more complex and harder to setup though, so if you just want to use the application personally then the first option is perfectly fine.
Follow this link if you are interested in learning how to use Oauth2 authentication with your program.
As a third option, you can just create a new Gmail account, and then lower the security settings on that instead. (So even in the worst case scenario, your main account is perfectly safe)
Sending Emails with Gmail in Python
Once you have completed either one of the two methods, you are now ready to begin sending emails in Python through Gmail.
Setting up a Connection with SMTP
The first step is to create a SMTP object that you can use to execute various methods.
smtpobject = smtplib.SMTP('smtp.gmail.com', 587)
The first parameter in the SMTP function defines the server name we wish to connect to. For a Gmail account the server name we use is ‘smtp.gmail.com
‘. Different providers (like Hotmail) have different server names.
The second parameter is the port 587 is the port used by TLS, the encryption standard. May be different in some rare occasions.
Once the SMTP()
connection has been made, you can start the TLS session using the starttls()
function.
smtpobject.starttls()
If the TLS call fails, you can always use SSL, another encryption protocol. The SSL connection operates on the 465 port. You can call it using the SMTP_SSL()
function.
smtpobject = smtplib.SMTP_SSL('smtp.gmail.com', 465)
SSL is also a pretty secure format for sending messages across the internet.
Now that we’ve established a connection and created end-to-end encryption we can begin safely with the login process. To login in to your Gmail account, use the login() function which takes your email and password as it’s parameters.
smtpobject.login('[email protected]', 'password')
Sending an Email with SMTP
Finally, it’s time to use the sendmail()
function. It takes three parameters, your email, the recipent’s email and the message to be sent.
You can use the newline character “\n
” to to separate the lines in your message. Keep in mind that your email message must begin with "Subject: subject\n"
.
smtpobject.sendmail('[email protected]', '[email protected]',
'''Subject: Appreciation\n
Dear Contributor, Your contributed article is greatly appreciated''')
The return value from this function is a dictionary. If this dictionary is empty, it means your message was successfully sent to the recipient(s). Otherwise, there will be one key:value pair in the dictionary for each recipient the message failed to be sent.
Trying to write out the whole message in a single function can be a bit messy. So we can store it in a separate variable first in the form of a multiline string. Just remember that in the Multiline string, each new line represents a new line in the message. If you store it all in one consecutive line, it might put the whole text in the subject. (The subject is regarded as the first line)
The complete code:
import smtplib
smtpobject = smtplib.SMTP('smtp.gmail.com', 587)
smtpobject.starttls()
smtpobject.login('[email protected]', 'password')
message = '''\
Subject: Appreciation\n
Dear Contributor, Your contributed article is greatly appreciated.
Looking forward to future contributions!'''
smtpobject.sendmail('[email protected]', '[email protected]', message)
smtpobject.quit()
Once you’re done with everything we use the smtpobject.quit()
to close the SSL/TLS connection.
If you are interested in learning more about the SMTP and IMAP (receiving emails) Libraries, refer to this tutorial on automating emails in python.
This marks the end of the How to Send Emails with Gmail in Python Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.