Creating your own Widget Styles and Custom Themes can take up alot of space inside your Python file. Especially if you are creating your own theme, which requires you to define the styles for pretty much every widget. To help you resolve this problem, we will teach you how to import Tkinter ttk Styles from a separate file where we can store all of our Styling code.
Import Widget Styles in Tkinter (from a File)
We will be creating two different files in todays tutorial. Here is our main file, called “mainfile.py
“. The main Tkinter code is located in this file, along with all the widgets that will show up on our Window.
import tkinter as tk
import tkinter.ttk as ttk
root = tk.Tk()
root.geometry('200x150')
button = ttk.Button(root, text = "Click Me!")
button.pack()
root.mainloop()
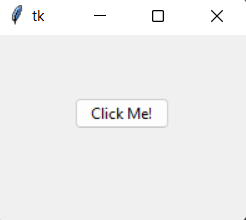
The goal of this tutorial is to define some custom styles for this button in a separate file called customstyles.py
. We will create a new function in the customstyles.py
file, which returns a “style” object.
All styling and theme customizations are done through this style object. All we have to do is create this style object in our customstyles.py
file, apply whatever customizations we want, and then return it back to the main file.
Here is the code for it.
import tkinter as tk
import tkinter.ttk as ttk
def CreateStyle():
return ttk.Style().configure("Custom.TButton",
font = "Verdana 16",
foreground = "green")
The above styles will create a new custom style called Custom.TButton
, which a font-size of 16, font family of Verdana and will have green text.
Now we need to import this file in our main file, and run this function.
import customstyles
import tkinter as tk
import tkinter.ttk as ttk
root = tk.Tk()
root.geometry('200x150')
style = styles.CreateStyle()
button = ttk.Button(root, text = "Click Me!", style = "Custom.TButton")
button.pack(padx=50, pady=50)
root.mainloop()
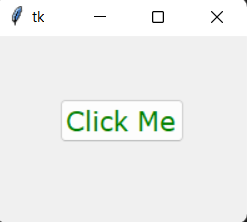
Remember to pass in the style name to the button as well! You won’t have to do this if you modified the default TButton style though.
If you are interested in learning more about Tkinter Styling options, and how to create your own themes, refer to these Styles tutorials.
This marks the end of the How to Import Tkinter ttk Styles from separate file? Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the Tutorial content can be asked in the comments section below.